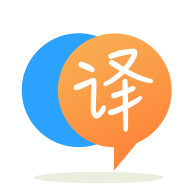
[英]C#: Define methods implementation in base class and properties in derived classes
[英]C# - How to make the following class a base class so that child classes can have the same properties, methods?
我创建了以下类,该类可用于特定的数据库表。 如何使此类成为基类,以便其他类可以具有相同的属性和方法,但是返回正确的类型,而我唯一要做的就是分配正确的tableName?
预先感谢,希望我的问题清楚。
这是课程:
public class AccountType
{
private static string tableName = "accountTypes";
private int id = -1;
public int Id
{
get
{
return id;
}
set
{
id = value;
}
}
private string name = "";
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
private static List<AccountType> accountTypes = new List<AccountType> ();
public static List<AccountType> AccountTypes
{
get
{
return accountTypes;
}
}
public AccountType ()
{
}
public AccountType Clone ()
{
AccountType o = (AccountType)this.MemberwiseClone ();
return o;
}
public static AccountType Fill (DataRow row)
{
int id = Convert.ToInt32 (row["id"].ToString ());
string name = row["name"].ToString ();
AccountType o = new AccountType ();
o.id = id;
o.name = name;
return o;
}
public static List<AccountType> FillAll (DataRowCollection rows)
{
List<AccountType> objs = new List<AccountType> ();
foreach (DataRow row in rows)
{
AccountType o = Fill (row);
if (o != null)
objs.Add (o);
}
return objs;
}
public static List<AccountType> GetAll ()
{
if (AccountType.accountTypes.Count > 0)
return AccountType.accountTypes;
List<AccountType> objs = new List<AccountType> ();
string query = "SELECT * \r\n" +
"FROM " + AccountType.tableName + " \r\n" +
"WHERE id > -1 \r\n" +
"ORDER BY name";
DataSet result = Global.Db.ExecuteQuery (query);
if (
(result == null)
|| (result.Tables[0] == null)
|| (result.Tables[0].Rows.Count < 1)
)
{
return objs;
}
objs = FillAll (result.Tables[0].Rows);
return objs;
}
public static AccountType GetById (int id)
{
foreach (AccountType at in AccountType.accountTypes)
{
if (at.id == id)
return at;
}
AccountType o = null;
string query = "SELECT * \r\n" +
"FROM " + AccountType.tableName + " \r\n" +
"WHERE id = " + id + " \r\n";
DataSet result = Global.Db.ExecuteQuery (query);
if (
(result == null)
|| (result.Tables[0] == null)
|| (result.Tables[0].Rows.Count < 1)
)
{
return o;
}
o = Fill (result.Tables[0].Rows[0]);
return o;
}
public static void Load ()
{
AccountType.accountTypes = AccountType.GetAll ();
}
public void Save ()
{
string tn = AccountType.tableName;
string query = "INSERT INTO " + tn + " (name) " +
"VALUES ( @name)";
SQLiteCommand command = new SQLiteCommand ();
command.CommandText = query;
command.CommandType = CommandType.Text;
command.Parameters.Add (new SQLiteParameter("@currencyPair", this.name));
Common.Global.Db.ExecuteNonQuery (command);
}
public void Update ()
{
string query = "UPDATE " + AccountType.tableName + " \r\n" +
"SET name = @name \r\n" +
"WHERE id = @id";
SQLiteCommand command = new SQLiteCommand ();
command.CommandText = query;
command.CommandType = CommandType.Text;
command.Parameters.Add (new SQLiteParameter("@id", this.id));
command.Parameters.Add (new SQLiteParameter("@name", this.name));
Common.Global.Db.ExecuteNonQuery (command);
}
}
我有80%的把握确保我了解您的问题,因此希望这个答案会有所帮助。 我认为奇怪的重复模板模式可能会有所帮助
想法是您执行此操作(我刚刚实现了随机位,以期希望它可以为您做些什么)
public abstract class BaseClass<T> where T : BaseClass<T>
{
public string Id {get; set;}
public string OtherProperty {get; set;}
public T WorkWithTAndReturn(T instanceOfASubClass)
{
//you can access base properties like
var theId = T.Id;
T.OtherProperty = "Look what I can do";
return T
}
public T Clone()
{
var newT = DoCloneStuff(instanceToClone);//do clone stuff here
return newT;
}
public static T Clone(T instanceToClone)
{
return (T)instanceToClone.MemberwiseClone();
}
}
注意:'BaseClass'是抽象的,因为您不想实例化该实例。 因为T必须是BaseClass<T>
的子类,所以您必须实例化该子类,因此不必执行此新的BaseClass,因为那只是不必要的和奇怪的。 使它抽象也可以使实现者在需要时提供一些功能
因此,然后创建一个从BaseClass<T>
派生的类
public class MySubClass : BaseClass<MyClass>
{
//put what ever implementation you want in here
}
它可以用来
var mySubClass = new MySubClass();
SubClass clone = mySubClass.Clone();
SubClass otherClone = SubClass.Clone(mySubClass);
编辑:更多信息
public static T Fill(DataRow row)
{
int id = Convert.ToInt32(row["id"].ToString());
string name = row["name"].ToString();
T o = new T();
o.id = id;
o.name = name;
return o;
}
public static List<T> FillAll(DataRowCollection rows)
{
List<T> objs = new List<T>();
foreach (DataRow row in rows)
{
T o = Fill(row);
if (o != null)
objs.Add(o);
}
return objs;
}
这种模式解决了静态函数的一些问题。 但是,静态字段或属性只能在实现它们的类上访问。 它们不是继承的。 即使B类继承自A类,A类上的静态字符串属性也不属于B类。
我认为这种模式将为您指明正确的方向,但是您将需要重新思考一下您的模式
编辑2:好的,这就是绕过您拥有的静态表名字段的这一障碍的想法
public abstract class TableDesciptor
{
public abstract string TableName { get; }
}
public class AccountType<T,U>
where T : AccountType<T,U>, new()
where U : TableDesciptor, new()
{
private static string tableName = new U().TableName;
public static List<T> AccountTypes
{
get
{
return accountTypes;
}
}
public int Id { get; set; }
public string Name { get; set; }
private static List<T> accountTypes = new List<T>();
public AccountType()
{
}
public T Clone()
{
T o = (T)this.MemberwiseClone();
return o;
}
public static T Fill(DataRow row)
{
int id = Convert.ToInt32(row["id"].ToString());
string name = row["name"].ToString();
T o = new T();
o.Id = id;
o.Name = name;
return o;
}
public static List<T> FillAll(DataRowCollection rows)
{
List<T> objs = new List<T>();
foreach (DataRow row in rows)
{
T o = Fill(row);
if (o != null)
objs.Add(o);
}
return objs;
}
public static List<T> GetAll()
{
if (accountTypes.Count > 0)
return accountTypes;
List<T> objs = new List<T>();
string query = "SELECT * \r\n" +
"FROM " + AccountType<T,U>.tableName + " \r\n" +
"WHERE id > -1 \r\n" +
"ORDER BY name";
DataSet result = Global.Db.ExecuteQuery(query);
if (
(result == null)
|| (result.Tables[0] == null)
|| (result.Tables[0].Rows.Count < 1)
)
{
return objs;
}
objs = FillAll(result.Tables[0].Rows);
return objs;
}
public static T GetById(int id)
{
foreach (T at in accountTypes)
{
if (at.Id== id)
return at;
}
T o = null;
string query = "SELECT * \r\n" +
"FROM " + AccountType<T,U>.tableName + " \r\n" +
"WHERE id = " + id + " \r\n";
DataSet result = Global.Db.ExecuteQuery(query);
if (
(result == null)
|| (result.Tables[0] == null)
|| (result.Tables[0].Rows.Count < 1)
)
{
return o;
}
o = Fill(result.Tables[0].Rows[0]);
return o;
}
public static void Load()
{
accountTypes = GetAll();
}
public void Save()
{
string tn = AccountType<T,U>.tableName;
string query = "INSERT INTO " + tn + " (name) " +
"VALUES ( @name)";
SQLiteCommand command = new SQLiteCommand();
command.CommandText = query;
command.CommandType = CommandType.Text;
command.Parameters.Add(new SQLiteParameter("@currencyPair", this.Name));
Common.Global.Db.ExecuteNonQuery(command);
}
public void Update()
{
string query = "UPDATE " + AccountType<T,U>.tableName + " \r\n" +
"SET name = @name \r\n" +
"WHERE id = @id";
SQLiteCommand command = new SQLiteCommand();
command.CommandText = query;
command.CommandType = CommandType.Text;
command.Parameters.Add(new SQLiteParameter("@id", this.Id));
command.Parameters.Add(new SQLiteParameter("@name", this.Name));
Common.Global.Db.ExecuteNonQuery(command);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.