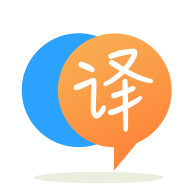
[英]Initialization of std::string from std::vector<unsigned char> causes error
[英]Letting a `std::vector<unsigned char>` steal memory from a `std::string`
假设我们有std::string s
持有原始数据缓冲区,但我们想要std::vector<uint8_t> v
代替。 缓冲区长度以百万计。 有没有一种简单的方法可以让v
窃取s
的内存,从而避免复制缓冲区?
就像std::vector<uint8_t>::vector(std::string&&)
那样,但是从STL的外部以某种方式进行。
或者,是否可以通过与ss.str()
一样有效的操作从std::stringstream ss
获取v
?
好,那里有很多评论,让我们尝试将它们放在一起,因为我需要练习,并且可能会获得一些积分[更新:没有:(]。
我对“现代C ++”还不陌生,因此请随便使用。 可能需要迟到C ++ 17,我还没有仔细检查过。 任何批评都值得欢迎,但我希望编辑自己的帖子。 并且在阅读本文时请记住,OP 实际要执行的操作是从文件中读取其字节。 谢谢。
更新:调整以处理以下情况,即文件大小根据下面@Deduplicator的注释在stat()
调用与fread()
调用之间改变...然后将fread
替换为std::ifstream
,我认为现在在那里。
#include <string>
#include <vector>
#include <optional>
#include <iostream>
#include <fstream>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <stdio.h>
#include <errno.h>
using optional_vector_of_char = std::optional <std::vector <char>>;
// Read file into a std::optional <std::vector <char>>.
// Now handles the file size changing when we're not looking.
optional_vector_of_char SmarterReadFileIntoVector (std::string filename)
{
for ( ; ; )
{
struct stat stat_buf;
int err = stat (filename.c_str (), &stat_buf);
if (err)
{
// handle the error
return optional_vector_of_char (); // or maybe throw an exception
}
size_t filesize = stat_buf.st_size;
std::ifstream fs;
fs.open (filename, std::ios_base::in | std::ios_base::binary);
if (!fs.is_open ())
{
// handle the error
return optional_vector_of_char ();
}
optional_vector_of_char v (filesize + 1);
std::vector <char>& vecref = v.value ();
fs.read (vecref.data (), filesize + 1);
if (fs.rdstate () & std::ifstream::failbit)
{
// handle the error
return optional_vector_of_char ();
}
size_t bytes_read = fs.gcount ();
if (bytes_read <= filesize) // file same size or shrunk, this code handles both
{
vecref.resize (bytes_read);
vecref.shrink_to_fit ();
return v; // RVO
}
// File has grown, go round again
}
}
int main ()
{
optional_vector_of_char v = SmarterReadFileIntoVector ("abcde");
std::cout << std::boolalpha << v.has_value () << std::endl;
}
现场演示 。 当然没有可用的实际文件可供读取,因此...
另外 :您是否考虑过编写自己的简单容器来映射文件视图? 只是一个想法。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.