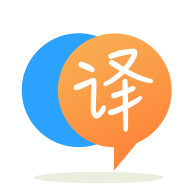
[英]How can I manipulate JSON data into javascript and display onto HTML
[英]How can i manipulate json values
我有一个休息api返回数据库查询的json和json看起来像
{"keys": "[{'aht': Decimal('238'), 'month': 'April '}, {'aht': Decimal('201'), 'month': 'August '}, {'aht': Decimal('236'), 'month': 'December '}, {'aht': Decimal('230'), 'month': 'February '}, {'aht': Decimal('228'), 'month': 'January '}, {'aht': Decimal('202'), 'month': 'July '}, {'aht': Decimal('201'), 'month': 'June '}, {'aht': Decimal('239'), 'month': 'March '}, {'aht': Decimal('214'), 'month': 'May '}, {'aht': Decimal('235'), 'month': 'November '}, {'aht': Decimal('221'), 'month': 'October '}, {'aht': Decimal('147'), 'month': 'September'}]", "success": true}
所以从下面
{'aht': Decimal('238'), 'month': 'April '}
删除Decimal()
预期:
{'aht': '238', 'month': 'April '}
我可以在python 3.6或js中处理它。
您可以尝试先对JSON字符串进行预处理,然后再在应用程序中使用它,如下所示。
或者,如果您希望REST API发送此输出以与其他东西一起使用,并且唯一的问题是JS / Python(当您想将其作为JSON处理时),请为其余端点实现一个参数,以指定是否进行清理(JSON格式) )是否必要。 根据该参数,在服务器端进行必要的处理以删除Decimal等。
let str = `{"keys": "[{'aht': Decimal('2'), 'month': 'April '}, {'aht': Decimal('20'), 'month': 'August '}, {'aht': Decimal('236'), 'month': 'December '}, {'aht': Decimal('230'), 'month': 'February '}, {'aht': Decimal('228'), 'month': 'January '}, {'aht': Decimal('202'), 'month': 'July '}, {'aht': Decimal('201'), 'month': 'June '}, {'aht': Decimal('239'), 'month': 'March '}, {'aht': Decimal('214'), 'month': 'May '}, {'aht': Decimal('235'), 'month': 'November '}, {'aht': Decimal('221'), 'month': 'October '}, {'aht': Decimal('147'), 'month': 'September'}]", "success": true}`; let cleanJSON = str.replace(/Decimal\\('([0-9]*)'\\)/g, function(x) { return x.substring(9, x.length - 2); }); console.log(JSON.parse(cleanJSON));
正如@Jaromanda所说,您的API数据不是您想要的格式。 请再次检查。 如果您得到的数据像我在这里提到的那样,则可以使用我的方法。
var jsonData = {"keys": [{'aht': "Decimal('238')", 'month': 'April '}, {'aht': "Decimal('201')", 'month': 'August '}, {'aht': "Decimal('236')", 'month': 'December '}, {'aht': "Decimal('230')", 'month': 'February '}, {'aht': "Decimal('228')", 'month': 'January '}, {'aht': "Decimal('202')", 'month': 'July '}, {'aht': "Decimal('201')", 'month': 'June '}, {'aht': "Decimal('239')", 'month': 'March '}, {'aht': "Decimal('214')", 'month': 'May '}, {'aht': "Decimal('235')", 'month': 'November '}, {'aht': "Decimal('221')", 'month': 'October '}, {'aht': "Decimal('147')", 'month': 'September'}], "success": true} if( jsonData.keys ) { jsonData.keys.map( ( data ) => { if( data.aht && data.aht.match( /Decimal\\(/ ) ) { data.aht = data.aht.replace( "Decimal('", "" ); data.aht = data.aht.replace( "')", "" ); } } ) } console.log(jsonData.keys)
将其转换为dict的字符串表示形式,然后使用ast.literal_eval
进行实际的dict(比使用纯Python eval
更安全)会更容易。 您必须执行以下步骤:
ast.literal_eval
评估整体字典 ast.literal_eval
的第二次调用来评估“键” dict值 看起来像这样:
import re
import ast
def make_dict(s):
# extract integer part of Decimal values
ss = re.sub(r"Decimal\('(\d+)'\)", r"\1", s)
# convert boolean literals
ss = ss.replace("true", "True")
ss = ss.replace("false", "False")
# convert dict
dictval = ast.literal_eval(ss)
# convert 'keys' entry
dictval['keys'] = ast.literal_eval(dictval['keys'])
return dictval
它在起作用:
text = """{"keys": "[{'aht': Decimal('238'), 'month': 'April '}, {'aht': Decimal('201'), 'month': 'August '}, {'aht': Decimal('236'), 'month': 'December '}, {'aht': Decimal('230'), 'month': 'February '}, {'aht': Decimal('228'), 'month': 'January '}, {'aht': Decimal('202'), 'month': 'July '}, {'aht': Decimal('201'), 'month': 'June '}, {'aht': Decimal('239'), 'month': 'March '}, {'aht': Decimal('214'), 'month': 'May '}, {'aht': Decimal('235'), 'month': 'November '}, {'aht': Decimal('221'), 'month': 'October '}, {'aht': Decimal('147'), 'month': 'September'}]", "success": true}"""
import pprint
pprint.pprint(make_dict(text))
给出:
{'keys': [{'aht': 238, 'month': 'April '},
{'aht': 201, 'month': 'August '},
{'aht': 236, 'month': 'December '},
{'aht': 230, 'month': 'February '},
{'aht': 228, 'month': 'January '},
{'aht': 202, 'month': 'July '},
{'aht': 201, 'month': 'June '},
{'aht': 239, 'month': 'March '},
{'aht': 214, 'month': 'May '},
{'aht': 235, 'month': 'November '},
{'aht': 221, 'month': 'October '},
{'aht': 147, 'month': 'September'}],
'success': True}
请通过使用str
将人们将dict转换为字符串来告诉人们停止将dicts存储在数据库中。
您可以像下面这样简单地将字符串replace()
与RegEx一起使用:
var keysObj = {"keys": "[{'aht': Decimal('238'), 'month': 'April '}, {'aht': Decimal('201'), 'month': 'August '}, {'aht': Decimal('236'), 'month': 'December '}, {'aht': Decimal('230'), 'month': 'February '}, {'aht': Decimal('228'), 'month': 'January '}, {'aht': Decimal('202'), 'month': 'July '}, {'aht': Decimal('201'), 'month': 'June '}, {'aht': Decimal('239'), 'month': 'March '}, {'aht': Decimal('214'), 'month': 'May '}, {'aht': Decimal('235'), 'month': 'November '}, {'aht': Decimal('221'), 'month': 'October '}, {'aht': Decimal('147'), 'month': 'September'}]", "success": true}; keysObj.keys = keysObj.keys.replace(/Decimal[\\(^0-9]|\\)/g, ''); console.log(keysObj);
就像每个人都说的那样,您可以做的最好的事情就是告诉中间件人完成这项工作,因为这对于前端来说是不必要的负担。 否则,您可以使用正则表达式(JavaScript)替换Decimal()和'。
var jsonObj = {"keys": "[{'aht': Decimal('238'), 'month': 'April '}, {'aht': Decimal('201'), 'month': 'August '}, {'aht': Decimal('236'), 'month': 'December '}, {'aht': Decimal('230'), 'month': 'February '}, {'aht': Decimal('228'), 'month': 'January '}, {'aht': Decimal('202'), 'month': 'July '}, {'aht': Decimal('201'), 'month': 'June '}, {'aht': Decimal('239'), 'month': 'March '}, {'aht': Decimal('214'), 'month': 'May '}, {'aht': Decimal('235'), 'month': 'November '}, {'aht': Decimal('221'), 'month': 'October '}, {'aht': Decimal('147'), 'month': 'September'}]", "success": true} // cleans the JSON object to generate a valid JSON. jsonObj.keys = jsonObj.keys.replace(/Decimal[\\(^0-9]|\\)/g, '').replace(/'/gm, '"'); jsonObj.keys = JSON.parse(jsonObj.keys); console.log(jsonObj);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.