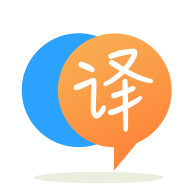
[英]Inheritance without inheriting, is there create dinamically attributes that point to other class variables?
[英]A point class and a rectangle class without using inheritance
我正在尝试创建一个点类,它具有浮点值“x”和“y”的数据属性,因此定义了一个点在 2D 空间中的位置。 此外,我想要init方法,例如它是默认值 x = 0 和 y = 0 的初始化。然后是一个移动函数,它接受“x”和“y”作为该点的新位置。 最后是一个函数,它告诉一个点的距离。 我希望这能返回从这一点到 x, y 处的另一个点的欧几里得距离。 这将如何完成?
这是我迄今为止用于上述描述的代码:
import math
class Point:
def __init__(self):
self.x = 0 # initialize to 0
self.y = 0 # initialize to 0
def move(self, x, y):
self.x = x
self.y = y
可以使用此帮助以及从该点到 x,y 处的另一点的欧几里安距离。 不知道到目前为止我是否有正确的想法。 python的新手所以不知道如何测试这段代码的功能。 将不胜感激的帮助!
在那部分之后,我将能够在矩形的两个对角处定义两个点,并在不使用继承的情况下使用上面定义的点。 关于如何创建这个类的任何想法?
你可以做这样的事情(代码中的注释):
class Point:
def __init__(self, x: float=0.0, y: float=0.0)-> None: # assign default values
self.x = x
self.y = y
def move_by(self, dx: float, dy: float)-> None: # move Point by dx, dy
self.x += dx
self.y += dy
def move_to(self, new_x: float, new_y: float)-> None: # relocate Point to new x, y position
self.x = new_x
self.y = new_y
def distance(self, other: 'Point')-> float: # calculates and returns the Euclidian distance between self and other
if isinstance(other, Point):
x0, y0 = self.x, self.y
x1, y1 = other.x, other.y
return ((x1 - x0)**2 + (y1 - y0)**2) ** 0.5
return NotImplemented
def __str__(self)-> str: # add a nice string representation
return f'Point({self.x}, {self.y})'
p = Point(1, 2)
q = Point()
print(p, q, p.distance(q) == 5**0.5)
p.move_by(.1, -.1)
print(p)
Point(1, 2) Point(0.0, 0.0) True
Point(1.1, 1.9)
而 Rectangle 类可能是这样的: [编辑添加默认Point
值]
在Rectangle.__init__
,对作为参数提供的Points
的 x 和 y 值的最小值和最大值进行排序,以确定定义矩形的左上角和右下角点
class Rectangle:
def __init__(self, p0: Point=Point(), p1: Point=Point(1.0, 1.0))-> None: # <--- [edit]: now with default values
x0, y0 = p0.x, p0.y
x1, y1 = p1.x, p1.y
self.topleft = Point(min(x0, x1), max(y0, y1)) # calculate the topleft and bottomright
self.bottomright = Point(max(x0, x1), min(y0, y1)) # of the bounding box
def __str__(self)-> str:
return f'Rectangle defined by bbox at: {self.topleft}, {self.bottomright})'
p = Point(1, 2)
q = Point()
print(p, q, p.distance(q) == 5**0.5)
p.move_by(.1, -.1)
print(p)
r = Rectangle(p, q)
print(r)
Point(1, 2) Point(0.0, 0.0) True
Point(1.1, 1.9)
Rectangle defined by bbox at: Point(0.0, 1.9), Point(1.1, 0.0))
s = Rectangle()
print(s)
Rectangle defined by bbox at: Point(0.0, 1.0), Point(1.0, 0.0))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.