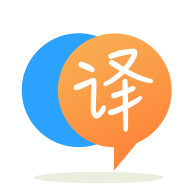
[英]Comparing each element of a list to their corresponding index in another
[英]What is the fastest way to compare each element of a list with corresponding element of another list?
我想将一个列表的每个元素与另一个列表的对应元素进行比较,以查看它的大小是多少。
list1 = [4,1,3]
list2 = [2,5,2]
因此,将4
与2
相比较,将1
与5
相比较,将3
与2
。
除了使用for循环以外,还有其他快速的方法吗?
您可以为此使用numpy
库。 而且其速度明显更快
>>> import numpy as np
>>> list1 = np.array([4,1,3])
>>> list2 = np.array([2,5,2])
>>> list1 < list2
array([False, True, False])
运行该功能所花费的时间
>>> import timeit
>>> timeit.timeit("""
... import numpy as np
... list1 = np.array([4,1,3])
... list2 = np.array([2,5,2])
... print(list1 < list2)
... """,number=1)
[False True False]
0.00011205673217773438
如果您研究numpy的实现,那么numpy基本上是用C,C ++编写的事实使它变得相当快。
您可以使用zip()
联接列表元素,并使用列表推导来创建结果列表:
list1 = [4,1,3]
list2 = [2,5,2]
list1_greater_list2_value = [a > b for a,b in zip(list1,list2)]
print ("list1-value greater then list2-value:", list1_greater_list2_value)
输出:
list1-value greater then list2-value: [True, False, True]
这与普通循环的工作原理相同-但看起来更像pythonic。
你可以这样
使用lambda:
In [91]: map(lambda x,y:x<y,list1,list2)
Out[91]: [False, True, False]
使用zip
和for循环:
In [83]: [i<j for i,j in zip(list1,list2)]
Out[83]: [False, True, False]
lambda和for循环的执行时间:
In [101]: def test_lambda():
...: map(lambda x,y:x>y,list1,list2)
...:
In [102]: def test_forloop():
...: [i<j for i,j in zip(list1,list2)]
...:
In [103]: %timeit test_lambda
...:
10000000 loops, best of 3: 21.9 ns per loop
In [104]: %timeit test_forloop
10000000 loops, best of 3: 21 ns per loop
您可以将两个列表映射到一个运算符,例如int.__lt__
(“小于”运算符):
list(map(int.__lt__, list1, list2))
使用您的样本输入,将返回:
[False, True, False]
为了更好地进行比较,如果所有方法都以相同的方式计时:
paul_numpy: 0.15166378399999303
artner_LCzip: 0.9575707100000272
bhlsing_map__int__: 1.3945185019999826
rahul_maplambda: 1.4970900099999653
rahul_LCzip: 0.9604789950000168
用于计时的代码:
setup_str = '''import numpy as np
list1 = list(map(int, np.random.randint(0, 100, 1000000)))
list2 = list(map(int, np.random.randint(0, 100, 1000000)))'''
paul_numpy = 'list1 = np.array(list1); list2 = np.array(list2);list1 < list2'
t = timeit.Timer(paul_numpy, setup_str)
print('paul_numpy: ', min(t.repeat(number=10)))
artner = '[a > b for a,b in zip(list1,list2)]'
t = timeit.Timer(artner, setup_str)
print('artner_LCzip: ', min(t.repeat(number=10)))
blhsing = 'list(map(int.__lt__, list1, list2))'
t = timeit.Timer(blhsing, setup_str)
print('bhlsing_map__int__: ', min(t.repeat(number=10)))
rahul_lambda = 'list(map(lambda x,y:x<y,list1,list2))'
t = timeit.Timer(rahul_lambda, setup_str)
print('rahul_maplambda: ', min(t.repeat(number=10)))
rahul_zipfor = '[i<j for i,j in zip(list1,list2)]'
t = timeit.Timer(rahul_zipfor, setup_str)
print('rahul_LCzip: ', min(t.repeat(number=10)))
使用枚举消除了此处的任何zip
或map
的需要
[item > list2[idx] for idx, item in enumerate(list1)]
# [True, False, True]
实际上,我应该说,除了循环之外,没有其他更快的方法来执行所需的操作,因为如果您更接近问题,可以发现通过使用算法,该操作至少需要O(n)
才能完成。 因此所有答案都是正确的,并且可以使用Zip
或map
或...来实现,但是这些解决方案只会使您的实现更加美观和Pythonic。 速度不太快,在某些情况下(如急速回答),由于代码行少而不是时间复杂,所以速度稍快。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.