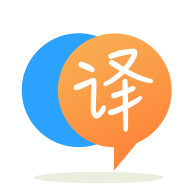
[英]How to run my maven project (angular+springboot) on tomcat?
[英]How to Package Angular Project with SpringBoot application
我的 eclipse 工作区中有一个名为 Test 的 SpringBoot Maven 项目。
之后,我在系统的另一个文件夹中创建(使用 ng,跟随 Angular 英雄之旅)一个 Angular 客户端。 现在,如果我使用命令行启动:
ng serve --open
在我的 angular 项目文件夹中,如果我启动我的 springboot 服务器应用程序,我可以运行 API GEt 和其他..
暂时我手动添加了命令获取到的dist文件夹的内容
ng build
在我在 SpringBoot 项目中手动创建的src/main/resources/static文件夹中。
当我通过 spring-boot:run 和 go 运行到 localhost:4200 它说连接被拒绝,检查你的代理或防火墙
目标将是 package 前端和后端到 tomcat 下可运行的单个战争。
你能帮助我吗? 谢谢你们。
您可以通过将 Angular CLI 配置为直接在 src/main/resources/static 文件夹中进行构建来避免复制过程。 如果您使用的是Angular 6 或更高版本,则可以在angular.json文件中更改构建输出路径-
{
"$schema": "./node_modules/@angular-devkit/core/src/workspace/workspace-schema.json",
"version": 1,
"newProjectRoot": "projects",
"projects": {
"angular.io-example": {
"root": "",
"projectType": "application",
"prefix": "app",
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser",
"options": {
"outputPath": "MODIFIED DIST PATH",
...
修改后的 dist 路径将是静态文件夹的相对路径。
如果您使用的是Angular 5或更低版本,您可以在.angular-cli.json文件中执行相同的操作 -
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
"project": {
"name": "angular5-sample"
},
"apps": [
{
"root": "src",
"outDir": "MODIFIED DIST PATH",
...
示例 - 如果您的 angular 源代码位于 src/main/webapp 文件夹中,则 outputPath 或 outDir 属性中修改后的 dist 路径将是 - '../resources/static/dist'。
这将直接在 src/main/resources/static 文件夹中创建 dist 文件夹。 要告诉 SpringBoot index.html 文件在 src/main/resources/static/dist 文件夹中,您应该通过在application.properties文件中添加 dist 文件夹的类路径来更新静态位置。
如果你在上面的例子中将 outputPath/outDir 设置为 '../resources/static',那么构建文件将直接在 src/main/resources/static 文件夹中生成。 但是,每次进行 Angular 构建时,Angular 构建过程都会删除整个静态文件夹,并使用新的构建文件创建一个新的静态文件夹。 您将丢失静态文件夹中存在的任何其他文件。 所以最好在静态文件夹内有一个单独的 dist 文件夹。
希望这可以帮助!
我找到了一个粗略的解决方案,但现在就足够了:
通过以下方式创建(每个人)一个 Angular 项目:
ng new angular-client
通过以下方式构建和部署它:
ng build --prod
将 dist 文件的内容放在 src/main/resources/static 中
maven clean install
spring-boot:run
它的工作原理。 显然,复制过程应该由 Maven 自动化
这是您的项目的示例目录布局:
src
├── main
│ ├── java
│ │ └── com
│ │ └── domain
│ │ └── project
│ │ ├── Run.java
│ ├── resources
│ │ ├── banner.txt
│ └── webapp
│ ├── WEB-INF
│ ├── index.html
│ ├── app
│ │ ├── app.module.js
│ │ ├── app.utils.js
不要将 Angular 文件放在src/main/resources/static
,而是尝试在src/main/webapp
创建一个新的 web 文件夹,并在其中创建一个新的app
文件夹来存储所有 Angular 代码。 这也是 Maven 中 web 项目的默认布局。
在你的pom.xml
你可以添加这个插件:
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<warSourceDirectory>src/main/webapp/</warSourceDirectory>
</configuration>
</plugin>
</plugins>
同样在您的@SpringBootApplication
类Run.java
添加此代码段,以便所有 404 请求都路由回index.html
以便它们由 Angular 处理:
@Bean
public ErrorPageRegistrar errorPageRegistrar() {
return (ErrorPageRegistry epr) -> {
epr.addErrorPages(new ErrorPage(HttpStatus.NOT_FOUND, "/index.html"));
};
}
您还可以使用以下 maven 插件依赖项来打包您的应用程序。 项目目录结构应如下所示。
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
<warSourceIncludes>WEB-INF/**,resources/public/build/**</warSourceIncludes>
<packagingIncludes>WEB-INF/**,resources/public/build/**</packagingIncludes>
</configuration>
</plugin>
<plugin>
<groupId>com.github.eirslett</groupId>
<artifactId>frontend-maven-plugin</artifactId>
<version>1.6</version>
<configuration>
<!-- optional: where to download node from. Defaults to https://nodejs.org/dist/ -->
<nodeDownloadRoot>http://nodejs.org/dist/</nodeDownloadRoot>
<!-- optional: where to download npm from. Defaults to https://registry.npmjs.org/npm/-/ -->
<npmDownloadRoot>http://registry.npmjs.org/npm/-/</npmDownloadRoot>
<workingDirectory>src/main/webapp/resources/public</workingDirectory>
</configuration>
<executions>
<execution>
<id>install node and npm</id>
<phase>process-classes</phase>
<goals>
<goal>install-node-and-npm</goal>
</goals>
<configuration>
<!-- See https://nodejs.org/en/download/ for latest node and npm (lts) versions -->
<nodeVersion>v8.9.4</nodeVersion>
<npmVersion>5.6.0</npmVersion>
</configuration>
</execution>
<execution>
<id>npm config</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<!-- Optional configuration which provides for running any npm command -->
<configuration>
<arguments>config set registry http://registry.npmjs.org/</arguments>
</configuration>
</execution>
<execution>
<id>npm install</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<!-- Optional configuration which provides for running any npm command -->
<configuration>
<arguments>install</arguments>
</configuration>
</execution>
<execution>
<id>npm run build</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<configuration>
<workingDirectory>src/main/webapp/resources/public</workingDirectory>
<arguments>run build</arguments>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
通过执行mvn clean install
您的应用程序将被打包并且可以使用 java 和 Angular 组件执行。
希望这可以帮助!
将 angular build 后的dist
内容放入src/main/resources/public
下的 Spring Boot Java 项目中,然后启动您的 Spring Boot 应用程序。
我在 angular 项目中创建了 dist 文件夹,并尝试在 spring boot 应用程序中运行它获取白标签页面错误并通过在应用程序中添加属性以某种方式解决了这个问题。 特性。
现在我的项目中出现 servlet 错误。
是否有任何配置可以在 spring boot 项目中运行我的 angular 应用程序
更新 spring boot application.properties 和 static 资源属性 spring.web.resources.static-locations=classpath:/static/dist/app-ui
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.