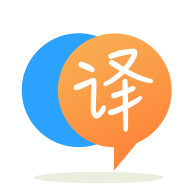
[英]How to run my maven project (angular+springboot) on tomcat?
[英]How to Package Angular Project with SpringBoot application
我的 eclipse 工作區中有一個名為 Test 的 SpringBoot Maven 項目。
之后,我在系統的另一個文件夾中創建(使用 ng,跟隨 Angular 英雄之旅)一個 Angular 客戶端。 現在,如果我使用命令行啟動:
ng serve --open
在我的 angular 項目文件夾中,如果我啟動我的 springboot 服務器應用程序,我可以運行 API GEt 和其他..
暫時我手動添加了命令獲取到的dist文件夾的內容
ng build
在我在 SpringBoot 項目中手動創建的src/main/resources/static文件夾中。
當我通過 spring-boot:run 和 go 運行到 localhost:4200 它說連接被拒絕,檢查你的代理或防火牆
目標將是 package 前端和后端到 tomcat 下可運行的單個戰爭。
你能幫助我嗎? 謝謝你們。
您可以通過將 Angular CLI 配置為直接在 src/main/resources/static 文件夾中進行構建來避免復制過程。 如果您使用的是Angular 6 或更高版本,則可以在angular.json文件中更改構建輸出路徑-
{
"$schema": "./node_modules/@angular-devkit/core/src/workspace/workspace-schema.json",
"version": 1,
"newProjectRoot": "projects",
"projects": {
"angular.io-example": {
"root": "",
"projectType": "application",
"prefix": "app",
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser",
"options": {
"outputPath": "MODIFIED DIST PATH",
...
修改后的 dist 路徑將是靜態文件夾的相對路徑。
如果您使用的是Angular 5或更低版本,您可以在.angular-cli.json文件中執行相同的操作 -
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
"project": {
"name": "angular5-sample"
},
"apps": [
{
"root": "src",
"outDir": "MODIFIED DIST PATH",
...
示例 - 如果您的 angular 源代碼位於 src/main/webapp 文件夾中,則 outputPath 或 outDir 屬性中修改后的 dist 路徑將是 - '../resources/static/dist'。
這將直接在 src/main/resources/static 文件夾中創建 dist 文件夾。 要告訴 SpringBoot index.html 文件在 src/main/resources/static/dist 文件夾中,您應該通過在application.properties文件中添加 dist 文件夾的類路徑來更新靜態位置。
如果你在上面的例子中將 outputPath/outDir 設置為 '../resources/static',那么構建文件將直接在 src/main/resources/static 文件夾中生成。 但是,每次進行 Angular 構建時,Angular 構建過程都會刪除整個靜態文件夾,並使用新的構建文件創建一個新的靜態文件夾。 您將丟失靜態文件夾中存在的任何其他文件。 所以最好在靜態文件夾內有一個單獨的 dist 文件夾。
希望這可以幫助!
我找到了一個粗略的解決方案,但現在就足夠了:
通過以下方式創建(每個人)一個 Angular 項目:
ng new angular-client
通過以下方式構建和部署它:
ng build --prod
將 dist 文件的內容放在 src/main/resources/static 中
maven clean install
spring-boot:run
它的工作原理。 顯然,復制過程應該由 Maven 自動化
這是您的項目的示例目錄布局:
src
├── main
│ ├── java
│ │ └── com
│ │ └── domain
│ │ └── project
│ │ ├── Run.java
│ ├── resources
│ │ ├── banner.txt
│ └── webapp
│ ├── WEB-INF
│ ├── index.html
│ ├── app
│ │ ├── app.module.js
│ │ ├── app.utils.js
不要將 Angular 文件放在src/main/resources/static
,而是嘗試在src/main/webapp
創建一個新的 web 文件夾,並在其中創建一個新的app
文件夾來存儲所有 Angular 代碼。 這也是 Maven 中 web 項目的默認布局。
在你的pom.xml
你可以添加這個插件:
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<warSourceDirectory>src/main/webapp/</warSourceDirectory>
</configuration>
</plugin>
</plugins>
同樣在您的@SpringBootApplication
類Run.java
添加此代碼段,以便所有 404 請求都路由回index.html
以便它們由 Angular 處理:
@Bean
public ErrorPageRegistrar errorPageRegistrar() {
return (ErrorPageRegistry epr) -> {
epr.addErrorPages(new ErrorPage(HttpStatus.NOT_FOUND, "/index.html"));
};
}
您還可以使用以下 maven 插件依賴項來打包您的應用程序。 項目目錄結構應如下所示。
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
<warSourceIncludes>WEB-INF/**,resources/public/build/**</warSourceIncludes>
<packagingIncludes>WEB-INF/**,resources/public/build/**</packagingIncludes>
</configuration>
</plugin>
<plugin>
<groupId>com.github.eirslett</groupId>
<artifactId>frontend-maven-plugin</artifactId>
<version>1.6</version>
<configuration>
<!-- optional: where to download node from. Defaults to https://nodejs.org/dist/ -->
<nodeDownloadRoot>http://nodejs.org/dist/</nodeDownloadRoot>
<!-- optional: where to download npm from. Defaults to https://registry.npmjs.org/npm/-/ -->
<npmDownloadRoot>http://registry.npmjs.org/npm/-/</npmDownloadRoot>
<workingDirectory>src/main/webapp/resources/public</workingDirectory>
</configuration>
<executions>
<execution>
<id>install node and npm</id>
<phase>process-classes</phase>
<goals>
<goal>install-node-and-npm</goal>
</goals>
<configuration>
<!-- See https://nodejs.org/en/download/ for latest node and npm (lts) versions -->
<nodeVersion>v8.9.4</nodeVersion>
<npmVersion>5.6.0</npmVersion>
</configuration>
</execution>
<execution>
<id>npm config</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<!-- Optional configuration which provides for running any npm command -->
<configuration>
<arguments>config set registry http://registry.npmjs.org/</arguments>
</configuration>
</execution>
<execution>
<id>npm install</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<!-- Optional configuration which provides for running any npm command -->
<configuration>
<arguments>install</arguments>
</configuration>
</execution>
<execution>
<id>npm run build</id>
<phase>process-classes</phase>
<goals>
<goal>npm</goal>
</goals>
<configuration>
<workingDirectory>src/main/webapp/resources/public</workingDirectory>
<arguments>run build</arguments>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
通過執行mvn clean install
您的應用程序將被打包並且可以使用 java 和 Angular 組件執行。
希望這可以幫助!
將 angular build 后的dist
內容放入src/main/resources/public
下的 Spring Boot Java 項目中,然后啟動您的 Spring Boot 應用程序。
我在 angular 項目中創建了 dist 文件夾,並嘗試在 spring boot 應用程序中運行它獲取白標簽頁面錯誤並通過在應用程序中添加屬性以某種方式解決了這個問題。 特性。
現在我的項目中出現 servlet 錯誤。
是否有任何配置可以在 spring boot 項目中運行我的 angular 應用程序
更新 spring boot application.properties 和 static 資源屬性 spring.web.resources.static-locations=classpath:/static/dist/app-ui
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.