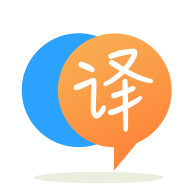
[英]Given an array and integer k find maximum value in each subarray of size k
[英]Given an array of integers and a number k, compute the maximum values of each subarray of length k
问题:给定一个整数数组和一个数字k,其中1 <= k <=数组的长度,计算长度为k的每个子数组的最大值。
在O(n)时间和O(k)空间中执行此操作。 您可以就地修改输入数组,而无需存储结果。 您可以在计算它们时简单地将它们打印出来。
示例:给定数组= [10、5、2、7、8、7]且k = 3,我们应该得到:[10、7、8、8],因为:
10 =最大值(10,5,2)
7 =最大值(5,2,7)
8 =最大值(2,7,8)
8 =最大值(7,8,7)
理念:
我想到了使用std :: max_element()函数来解决这一问题,其中我注意到了一个模式。 使用上面的例子
std :: max_element(0,2)= 10,当0是迭代器的开始位置,2是迭代器的结束位置时。
std :: max_element(1,3)= 7
std :: max_element(2,4)= 8
std :: max_element(3,5)= 8
因此,对于任何k,第一个迭代器将始终从0到n-2,其中n是向量或数组的大小,并且max_element的正确迭代器将始终从k-1到n-1。
尽管不是很简单,但为k的各种值指定正确的迭代器。 从您的代码中可以看到,我坚持了这一点,但我相信我的想法是正确的。 我希望我为其他人理解了这个想法。
码:
#include <iostream>
#include <vector>
#include <random>
#include <ctime>
// Given an array of integers and a number k, where 1 <= k <= length of the array, compute the maximum values
// of each subarray of length k.
// For example, given array = [10, 5, 2, 7, 8, 7] and k = 3, we should get : [10, 7, 8, 8], since :
// 10 = max(10, 5, 2)
// 7 = max(5, 2, 7)
// 8 = max(2, 7, 8)
// 8 = max(7, 8, 7)
// Do this in O(n) time and O(k) space. You can modify the input array in-place and you do not need to
// store the results. You can simply print them out as you compute them.
void printKMax(std::vector<int>& nums, int k)
{
int n = nums.size();
int i = 0;
int j = k - 1;
std::vector<int>::iterator it;
while (j <= n - 1)
{
it = std::max_element(nums.begin() + i, );
i++;
j++;
std::cout << *it << " ";
}
}
int main()
{
std::vector<int> nums = { 10, 5, 2, 7, 8, 7};
int k = 3;
printKMax(nums, k);
std::cin.get();
}
问题:我在为std :: max_element的正确部分找到公式以解决k的各种值时遇到问题。 任何帮助将不胜感激。
您将变量i
和j
保留为检查范围的开始和结束,因此需要将std::max_element
专门应用于该范围。 您正在寻找替换:
it = std::max_element(nums.begin() + i, );
与:
it = std::max_element(nums.begin() + i, nums.begin() + j + 1);
注意+ 1
部分。 这是因为STL标准惯例是对的右手边独家一个范围内工作。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.