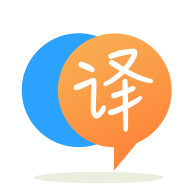
[英]Inherit Class1 and implement Interface1 both in case Class1 already implements Interface1
[英]How to fix this ISeries interface implementation in Class1?
我正在从 ISeries 接口的 Setstart(2) 函数开始开发一系列数字。 我试图在 Class1 中实现这个接口,但它向我抛出了一个错误。 我被这个错误困住了,无法解决这个问题。 我错过了什么? 请帮忙
我试图将接口中的所有函数设为公开 我试图从接口中删除公共访问说明符
public interface ISeries {
void Setstart (int a);
int GetNext ();
void Reset ();
}
class Class1 : ISeries {
int val;
void Setstart (int a) {
val = a;
}
int GetNext () {
return val++;
}
void Reset () {
val = 0;
}
static void Main () {
Class1 c = new Class1 ();
c.Setstart (2);
Console.WriteLine (c.GetNext ());
c.Reset ();
Console.WriteLine ();
}
}
我希望输出为 3 并且正在生成 0 错误
您需要public
您的方法,以便可以从类外部访问该方法,除了您的代码看起来不错之外,还缺少该类。
您需要根据您的场景将所有 3 种方法设为公开,例如:
public void Setstart (int a) {
并且您需要先加 1,然后返回以获取 3 作为输出,因为val++
将返回当前值,然后将其增加 1:
public int GetNext () {
// return val++; // Post increment will not work according to question.
val = val + 1;
return val;
}
具有完整接口实现的类将如下所示:
public class Class1 : ISeries {
int val;
public void Setstart (int a) {
val = a;
}
public int GetNext () {
val = val + 1;
return val;
}
public void Reset () {
val = 0;
}
}
你应该尝试这样的事情。
因为您必须使用一个变量,所以在这种情况下您必须使用ref
` 关键字。
并且您必须将class
中的所有方法标记为public
否则您将无法访问main
这些方法
代码:
using System;
namespace StackoverflowProblem
{
public interface ISeries
{
void Setstart(ref int value);
int GetNext(ref int value);
void Reset(ref int value);
}
public class Class1 : ISeries
{
public int val { get; set; }
public void Setstart(ref int value)
{
this.val = value;
}
public int GetNext(ref int value)
{
value = value + 1;
this.val = value;
return this.val;
}
public void Reset(ref int value)
{
// Resetting val.
value = 0;
this.val = value;
}
}
class Program
{
static void Main(string[] args)
{
Class1 c = new Class1();
int value = 2;
c.Setstart(ref value);
Console.WriteLine(" " + c.GetNext(ref value));
c.Reset(ref value);
Console.WriteLine();
}
}
}
输出:
val++ 将在返回当前值后递增,++val 将先递增,然后返回值,您现在应该得到 3,并使其适当范围以从外部访问方法
class Class1 : ISeries {
int val;
public void Setstart (int a) {
val = a;
}
public int GetNext () {
return ++val;
}
public void Reset () {
val = 0;
}
static void Main () {
Class1 c = new Class1();
c.Setstart(2);
Console.WriteLine (c.GetNext());
c.Reset();
Console.WriteLine ("");
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.