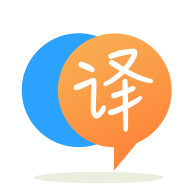
[英]Is it possible to use pipe (||) to set default parameters inside JavaScript object value
[英]JavaScript: correct way to set object parameters with name and default value
我要做的是将一个对象传递给一个函数,其中可以设置某些默认值,整个对象应该有一个名称将它传递给另一个函数。
以下代码,没有命名参数,工作得很好。
function test({ item1, item2 = 0 }) { console.log(item1 + " " + item2); } test({ item1: "foo" }); function print(obj) { console.log(obj.item1 + " " + obj.item2); }
但是,如果我现在开始设置obj = {...}
以传递给print()
我会收到语法错误:
function test(obj = { item1, item2 = 0 }) { print(obj); } test({ item1: "foo" }); function print(obj) { console.log(obj.item1 + " " + obj.item2); }
如果我写item2: 0
,将没有错误,但是在print
item2
没有找到。
从下面的答案来看,这似乎是迄今为止最适合我的方式:
function test(obj) { obj = Object.assign({ item1: undefined, item2: 0 }, obj); print(obj); } test({ item1: "foo" }); function print(obj) { console.log(obj.item1 + " " + obj.item2); }
解构从传递给函数的对象中提取属性,并将这些属性放入独立变量中 - 这就是全部。 您要做的是改变其中一个参数 ,而不是将参数中的属性提取到独立变量中 。
你不能改变参数列表中的参数 - 对于你正在寻找的那种逻辑,你必须在test
的函数体内test
:
function test(obj) { if (!obj.hasOwnProperty('item2')) { obj.item2 = 0; } print(obj); } test({ item1: "foo" }); function print(obj) { console.log(obj.item1 + " " + obj.item2); }
如果要为默认值分配许多属性,可以使用Object.assign
:
function test(obj) { const filledObj = Object.assign({ item2: 0, item3: 'item3' }, obj); print(filledObj); } test({ item1: "foo" }); function print(obj) { console.log(obj); }
如果您只希望将具有某些属性的对象传递给print
,那么在参数列表中提取这些属性就像您最初所做的那样,然后传递仅包含这些属性的重建对象进行print
:
function test({ item1, item2 = 0 }) { const obj = { item1, item2 }; print(obj); } test({ item1: "foo" }); function print(obj) { console.log(obj.item1 + " " + obj.item2); }
=
对于在javascript中分配值无效,您正在寻找{key: value}
。
将=
更改为:
以修复错误:
// You want to destructure you object, drop the `obj =` function test({item1,item2 = 0}) { // If you were to modify this data, // use `Object.assign({}, {item1,item2})` to prevent mutating your data print({item1,item2}); } // save some space by destructuring the items you want function print({item1, item2}) { console.log(`${item1} ${item2}`); } // Initial expected result test({item1: "foo"});
对象解构与默认值
我假设你期望item2
的值等于0
正确吗? 它不等于0
因为您传入的是一个覆盖函数参数中对象的新对象。
就像你如何设置一样:
function(a = 1){}
并且传入一个值, a
将不再等于1
因为它已被替换为新值。
你在第一个代码片段中得到预期行为的原因(没有obj = {...}
)是因为你正在解构一个对象。 这不是一个赋值,而是从对象中提取所需的片段。
当你有一个带有如下参数的函数时:
function({arg1, arg2}){}
JavaScript将从您传入的对象中提取这些键。
分配默认值
另一方面,如果你想传递一个没有解构的对象,你可以这样做:
function(obj = {a: 'default'}){}
但是,如果你在上面的函数中传入一个对象,那么对象及其所有键( a
或任何其他键)的默认值将被替换为你传入的任何对象。 这是一个关于默认参数如何工作的链接在javascript中 。
我强烈建议您在解构时采取措施,在javascript中处理对象或数组时非常有用。
希望这可以帮助,
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.