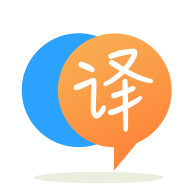
[英]Printing the values of a dictionary when its key is equal to the value of a list element
[英]Comparing element inside value of a key in a dictionary is equal to the next key
d = {
0:{1,2,3},
1:{567},
2:{2,3,5,8},
3:{4,5,7,9},
4:{6,7,8}
}
我想将第一个kv对的值与下一个kv对的键进行比较。
示例:要检查{1,2,3}
是否存在1
或{567}
是否存在2
,如果确实存在,那么我想删除值中存在的k。
输出应如下所示:
d = {
0:{1,2,3},
2:{2,3,5,8}
}
我尝试使用具有各种排列和组合的字典迭代器,但没有结果。 达到结果的最佳方法是什么?
Python字典不是按顺序排列的,因此我不确定您在这里真的可以说“上一个”的“下一个”。 使用pandas
系列会更合适。
但是,您仍然可以遍历键并将其定义为订单。
previous = {}
dict_items = list(d.items())
for k,v in dict_items:
if k in previous:
del d[k]
previous = v
编辑:确保键的顺序正确,更改dict_items
为:
dict_items = sorted(d.items(),key=lambda x:x[0])
会做到的
根据您的示例和要求进行猜测,您使用的是Python 3.6+,这些字典会保留插入顺序。 你可以做:
In [57]: d = {
...: 0:{1,2,3},
...: 1:{567},
...: 2:{2,3,5,8},
...: 3:{4,5,7,9},
...: 4:{6,7,8}
...: }
# Get an iterator from dict.keys
In [58]: keys_iter = iter(d.keys())
# Get the first key
In [59]: first_key = next(keys_iter)
# Populate output dict with first key-value
In [60]: out = {first_key: d[first_key]}
# Populate out dict with key-values based on condition by
# looping over the `zip`-ed key iterator and dict values
In [61]: out.update({k: d[k] for k, v in zip(keys_iter, d.values())
if k not in v})
In [62]: out
Out[62]: {0: {1, 2, 3}, 2: {2, 3, 5, 8}}
我花了一段时间才弄明白你想要什么。 在下面,我重新措辞了您的示例:
EXAMPLE:
Suppose the input dictionary is as follows:
0:{1,2,3},
1:{567},
2:{2,3,5,8},
3:{4,5,7,9},
4:{6,7,8}
We have...
key of 0:{1,2,3} is 0
value of 0:{1,2,3} is {1,2,3}
key of 2:{2,3,5,8} is 2
value of 2:{2,3,5,8} is {2,3,5,8}
We execute code similar to the following:
if key of 1:{567} in value of 0:{1,2,3}:
# if 1 in {1,2,3}:
delete 1:{567}
if key of 2:{2,3,5,8} in value of 1:{567}:
# if 2 in {567}:
delete 2:{2,3,5,8}
and so on...
"""
以下代码应该可以实现您的目标:
def cleanup_dict(in_dict):
# sort the dictionary keys
keys = sorted(indict.keys())
# keys_to_delete will tell us whether to delete
# an entry or not
keys_to_delete = list()
try:
while True:
prv_key = next(keys)
nxt_key = next(keys)
prv_val = in_dict[prv_key]
if (nxt_key in prv_val):
keys_to_delete.append(nxt_key)
except StopIteration:
pass
for key in keys_to_delete:
del in_dict[key]
return
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.