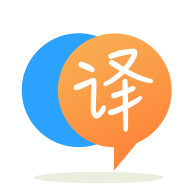
[英]Python while and for loops - can I make the code more efficient?
[英]How can I mix similar code using loops (for/while) in Python?
我重复了一些代码,仅更改了一些数字。
df_h0 = df.copy()
df_h0['hour']='00:00'
df_h0['totalCount']=df.post_time_data.str.split('"00:00","postCount":"').str[1].str.split('","topic').str[0]
df_h0 = df_h0.fillna(0)
df_h1 = df.copy()
df_h1['hour']='01:00'
df_h1['totalCount']=df.post_time_data.str.split('"01:00","postCount":"').str[1].str.split('","topic').str[0]
df_h1 = df_h1.fillna(0)
df_h2 = df.copy()
df_h2['hour']='02:00'
df_h2['totalCount']=df.post_time_data.str.split('"02:00","postCount":"').str[1].str.split('","topic').str[0]
df_h2 = df_h2.fillna(0)
我想通过循环简化此代码,但是由于我是Python的新手,所以我不确定如何从此开始。
我将尝试说明该过程的总体情况,以便将来自己解决这些问题。 但是,它不是自动的-您每次都需要考虑自己在做什么,以便编写出您能做到的最佳代码。
df_h0 = df.copy()
# ^^^ the variable name changes
df_h0['hour']='00:00'
# ^^^^^ the hour string changes
df_h0['totalCount']=df.post_time_data.str.split('"00:00","postCount":"').str[1].str.split('","topic').str[0]
# the delimiter string changes ^^^^^^^^^^^^^^^^^^^^^^^
df_h0 = df_h0.fillna(0)
这将使工作变得更加容易:)
我们有一个小时字符串,它也有所不同,而分隔符字符串,也有所不同。 但是定界符字符串始终具有相同的通用形式,该形式基于小时字符串。 因此,如果有小时字符串,则可以创建定界符字符串。 实际上,只有一条变化的信息-小时。 我们将调整代码以反映这一点:
hour = '00:00' # give the variable information a name
delimiter = f'"{hour}","postCount":"' # compute the derived information
# and then use those values in the rest of the code
df_h0 = df.copy()
df_h0['hour'] = hour
df_h0['totalCount']=df.post_time_data.str.split(delimiter).str[1].str.split('","topic').str[0]
df_h0 = df_h0.fillna(0)
这使我们为创建单个表的过程命名。 我们使用该函数的输入来提供我们在第3步中描述的变化信息。有一件事情发生了变化,因此将有一个参数来表示这一点。 但是,我们也需要提供我们与在这里工作的数据上下文 -的df
数据框中-这样的功能可以访问它。 因此,我们总共有两个参数。
def hourly_data(df, hour):
# since 'hour' was provided, we don't define it here
delimiter = f'"{hour}","postCount":"'
# now we use a generic name inside the function.
result = df.copy()
result['hour'] = hour
result['totalCount']=df.post_time_data.str.split(delimiter).str[1].str.split('","topic').str[0]
# At the last step of the original process, we `return` the value
# instead of simply assigning it.
return result.fillna(0)
现在,我们有了给定'hour'
字符串的代码,只需调用即可生成一个新的数据帧-例如: df_h0 = hourly_data(df, '00:00')
。
我们希望使用每个可能的小时值调用此函数,大概是从“ '00:00'
到“ '23:00'
包括0和0)。 但是,这些字符串具有明显的模式。 如果我们仅将小时数提供给hourly_data
,并让它产生字符串,则会更容易。
def hourly_data(df, hour):
# Locally replace the integer hour value with the hour string.
# The `:02` here is used to zero-pad and right-align the hour value
# as two digits.
hour = f'{hour:02}:00'
delimiter = f'"{hour}","postCount":"'
# The rest as before.
result = df.copy()
result['hour'] = hour
result['totalCount']=df.post_time_data.str.split(delimiter).str[1].str.split('","topic').str[0]
return result.fillna(0)
在Python中,将列表输入“转换”为另一输入列表的自然循环是列表理解 。 看起来像这样:
hourly_dfs = [hourly_data(df, hour) for hour in range(24)]
在这里, range
是一个内置函数,可为我们提供所需的输入值序列。
我们还可以使用for
循环手动构建列表:
hourly_dfs = []
for hour in range(24):
hourly_dfs.append(hourly_data(df, hour))
我们也可以在for
循环的主体内完成工作(其他人可能会附带另一个答案并显示类似的代码)。 但是通过首先创建函数,我们可以获得更易于理解的代码,并且还使我们能够使用列表理解。 列表推导方法更简单,因为我们不必考虑从空开始并.append
每个元素的过程,我们让Python建立列表而不是告诉它如何做。
您可以列出变量并对其进行迭代,然后使用string.format
方法
vars = [df_h0, df_h1, df_h2]
x = 0
for var in vars:
var = df.copy()
var['hour']='0{0}:00'.format(x)
var['totalCount']=df.post_time_data.str.split('0{0}:00", "postCount":'. format(x)).str[1].str.split('","topic').str[0]
var = var.fillna(0)
x += 1
如果您使用Python 3.6+,则也可以使用f strings
代替.format()
希望我没有错过任何事情,但是如果有的话,您可以通过声明诸如x
anither变量来实现与我所使用的相同的逻辑
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.