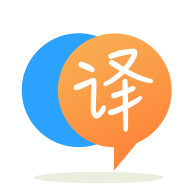
[英]Retrieve secrets from AWS Secrets Manager in a Lambda function
[英]AWS Secrets Manager call from Lambda returning null/none
我正在使用 AWS Lambda function 调用 AWS Secrets Manager 来检索秘密值,但它只返回值 None/Null。
这是我的代码:
# Secrets Manager
import boto3
import base64
from botocore.exceptions import ClientError
def lambda_handler(event, context):
# Secrets Manager
def get_secret():
secret_name = "arn:aws:secretsmanager:region:accountid:secret:full-secret-name"
region_name = "region"
# Create a Secrets Manager client
session = boto3.session.Session()
client = session.client(
service_name='secretsmanager',
region_name=region_name
)
# Only handle the specific exceptions for the 'GetSecretValue' API.
# See https://docs.aws.amazon.com/secretsmanager/latest/apireference/API_GetSecretValue.html
# We rethrow the exception by default.
try:
get_secret_value_response = client.get_secret_value(
SecretId=secret_name
)
except ClientError as e:
if e.response['Error']['Code'] == 'DecryptionFailureException':
# Secrets Manager can't decrypt the protected secret text using the provided KMS key.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InternalServiceErrorException':
# An error occurred on the server side.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InvalidParameterException':
# You provided an invalid value for a parameter.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InvalidRequestException':
# You provided a parameter value that is not valid for the current state of the resource.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'ResourceNotFoundException':
# We can't find the resource that you asked for.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
else:
# Decrypts secret using the associated KMS CMK.
# Depending on whether the secret is a string or binary, one of these fields will be populated.
if 'SecretString' in get_secret_value_response:
secret = get_secret_value_response['SecretString']
else:
decoded_binary_secret = base64.b64decode(get_secret_value_response['SecretBinary'])
secretValue = get_secret()
print(secretValue)
return secretValue
在回报中,我得到null
并在打印中得到None
。 不太确定我在这里做错了什么,但它不会出错也不会得到我的价值。
Secret 已加密,但 Lambda IAM 角色具有 KMS 密钥的使用权限,并且 KMS 密钥也已授予 IAM 角色使用权。
我也试过像这样将 secret_name 设置为 Secrets Name:
secret_name = "full-secret-name-no-arn"
问候
解析度:
# Secrets Manager
import json
import boto3
import base64
import logging
from botocore.exceptions import ClientError
logger = logging.getLogger()
logger.setLevel(logging.INFO)
def lambda_handler(event, context):
logger.info("Inside lambda_handler...")
# SecretsManager
secretValues = json.loads(get_secret())
def get_secret():
logger.info("Inside get_secret...")
secret_name = "full-arn-goes-here"
region_name = "region-goes-here"
# Create a Secrets Manager client
session = boto3.session.Session()
client = session.client(
service_name='secretsmanager',
region_name=region_name
)
try:
get_secret_value_response = client.get_secret_value(SecretId=secret_name)
logger.info("Received Response")
except ClientError as e:
if e.response['Error']['Code'] == 'DecryptionFailureException':
# Secrets Manager can't decrypt the protected secret text using the provided KMS key.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InternalServiceErrorException':
# An error occurred on the server side.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InvalidParameterException':
# You provided an invalid value for a parameter.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'InvalidRequestException':
# You provided a parameter value that is not valid for the current state of the resource.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
elif e.response['Error']['Code'] == 'ResourceNotFoundException':
# We can't find the resource that you asked for.
# Deal with the exception here, and/or rethrow at your discretion.
raise e
else:
# Please see https://docs.aws.amazon.com/secretsmanager/latest/apireference/CommonErrors.html for all the other types of errors not handled above
raise e
else:
# Decrypts secret using the associated KMS CMK.
# Depending on whether the secret is a string or binary, one of these fields will be populated.
if 'SecretString' in get_secret_value_response:
logger.info("Inside string response...")
return get_secret_value_response['SecretString']
else:
logger.info("Inside binary response...")
return base64.b64decode(get_secret_value_response['SecretBinary'])
只需将secretValues = json.loads(get_secret())
放入您的处理程序即可。
您的 get_secret() 没有 return 语句
除上述更改外,请确保您的 Lambda 执行角色具有访问机密管理器和 KMS 服务的适当权限。 这是附加到执行角色的示例 IAM 策略。
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "logs:CreateLogGroup",
"Resource": "arn:aws:logs:us-west-2:<ACCOUNT_ID>:*"
},
{
"Effect": "Allow",
"Action": [
"logs:CreateLogStream",
"logs:PutLogEvents"
],
"Resource": [
"arn:aws:logs:us-west-2:<ACCOUNT_ID>:log-group:/aws/lambda/sampletest*:*"
]
},
{
"Effect": "Allow",
"Action": [
"secretsmanager:GetSecretValue",
"secretsmanager:DescribeSecret",
"secretsmanager:ListSecretVersionIds",
"secretsmanager:PutSecretValue",
"secretsmanager:UpdateSecret",
"secretsmanager:TagResource",
"secretsmanager:UntagResource"
],
"Resource": [
"arn:aws:secretsmanager:us-west-2:<ACCOUNT_ID>:secret:<SECRET_NAME>"
]
},
{
"Effect": "Allow",
"Action": [
"kms:Decrypt"
],
"Resource": "arn:aws:kms:us-west-2:<ACCOUNT_ID>:key/<KMS_ID>"
}
]
}
这个问题很棘手……因为 AWS 不会为您提供任何有关缺少访问机密管理器服务的权限的有价值的日志信息。 您需要处理您正在运行的进程的 IAM 角色,并为 Secrets Manager 访问添加适当的权限,它将通过 go 没问题!!!
AWS 只是返回“None”而没有给您任何错误消息,这真是令人沮丧:(
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.