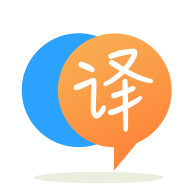
[英]How can i make this use multiple threads as clients - who all perform a task each within the switch statement
[英]How can I make an actionPerformed within a switch statement?
我正在尝试使用基于命令提示符的 GUI 制作一个简单的程序(即输入命令并单击回车按钮)。 每当用户单击 enter 按钮时,他们在 JTextArea 中键入的所有信息都会被处理,然后根据他们的输入(例如键入 /exit 以关闭程序)给出 output。
每当用户输入 /exit 时,都会出现一个提示,询问用户是否确定要退出。 但是,一旦弹出,它就会被我输入的无效输入消息所取代,如果用户输入了无法识别的内容。 我对为什么会发生这种情况的唯一原因是 ActionListener class 仍然将 /exit 存储在 inputHolder 字符串中,这是我用来存储用户输入并对其进行处理的字符串。
package msai;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class MainFrame extends JFrame implements ActionListener {
JTextArea output;
JButton inputButton;
JTextArea commandLine;
String inputHolder;
String invalid = "Invalid input!";
public MainFrame() {
super("MSAI");
setLookAndFeel();
setSize(650, 350);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
FlowLayout flo = new FlowLayout();
setLayout(flo);
output = new JTextArea("Hi! I'm MSAI. Type any command to get started, or type /help for a list of commands and syntax!", 8, 40);
inputButton = new JButton("ENTER");
inputButton.addActionListener(this);
commandLine = new JTextArea(8, 40);
commandLine.setLineWrap(true);
commandLine.setWrapStyleWord(true);
JScrollPane scroll = new JScrollPane(commandLine, JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
add(output);
add(inputButton);
add(scroll);
setVisible(true);
}
public void actionPerformed(ActionEvent event) {
inputHolder = commandLine.getText();
switch(inputHolder) {
"/exit":
output.setText("Are you sure you want to terminate this session?");
inputHolder = commandLine.getText();
switch(inputHolder.toUpperCase()) {
case "YES":
System.exit(0);
break;
case "NO":
output.setText("Your session has not been terminated.");
break;
default:
output.setText(invalid);
break;
}
}
}
private void setLookAndFeel() {
try {
UIManager.setLookAndFeel(
"javax.swing.plaf.nimbus.NimbusLookAndFeel"
);
} catch (Exception exc) {
//ignore error
}
}
public static void main(String[] args) {
MainFrame frame = new MainFrame();
}
}
目前我关于如何解决这个问题的唯一思考过程是将另一个 actionPerformed 放在 /exit switch 语句中,但我不确定如何做到这一点,或者这是否是最好的解决方案。 我会欢迎任何答案和反馈,只是请不要有毒!
当你这样做
output.setText("Are you sure you want to terminate this session?");
inputHolder = commandLine.getText();
用户尚未更新commandLine
并按下按钮,因此没有任何变化。 您需要“等待”直到用户第二次响应。
您需要将请求的“状态”转移到下一次调用ActionPerformed
时,例如......
private boolean wantsToExit = false
public void actionPerformed(ActionEvent event) {
inputHolder = commandLine.getText();
if (wantsToExit) {
if (wantsToExit && inputHolder.equals("YES")) {
// Better to close the frame and let the system deal with it
} else if (wantsToExit && inputHolder.equals("NO")) {
output.setText("Your session has not been terminated.");
}
wantsToExit = false;
} else {
switch(inputHolder) {
"/exit":
wantsToExit = true;
output.setText("Are you sure you want to terminate this session?");
break;
}
}
}
如果您想在单个流程中执行此操作,那么您应该考虑使用JOptionPane
来提示用户
问题是您的 actionPeformed 中的代码完全执行,用户无法输入 YES 或 NO 并单击“ENTER”按钮。 内部开关执行时 inputHolder 的值为“/exit”,这导致默认情况。
您将需要一种区分操作的方法。 您可以使用标志来指示用户是否已发出退出命令。 如果标志为真,则执行内部开关。 否则,从方法返回。
这是试图接近您最初想法的代码。
boolean exitCommand = false; //has the user issued exit command?
public void actionPerformed(ActionEvent event) {
inputHolder = commandLine.getText();
if (exitCommand){
switch (inputHolder.toUpperCase()) {
case "YES":
System.exit(0);
break;
case "NO":
exitCommand = false; //forget exit command.
output.setText("Your session has not been terminated.");
break;
default:
output.setText(invalid);
break;
}
}
switch (inputHolder) {
case "/exit":
exitCommand = true;
commandLine.setText("");
output.setText("Are you sure you want to terminate this session?");
inputHolder = commandLine.getText();
if (!exitCommand) {
exitCommand = true;
return;
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.