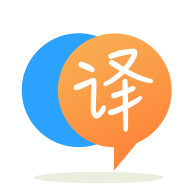
[英]How to do a recursion function that receives an array, find the sum of it and the integers in the array that divide the sum
[英]How to find the sum of sums of an array using recursion?
我正在尝试创建一个使用递归对数组的总和求和的程序。 例如,如果我有一个数组 {1, 8, 6, 15, 10},我将首先对元素 0-4 求和,然后对元素 1-4 求和,然后对 2-4 求和,直到我到达末尾数组的末尾。 一旦我得到所有的总和,我会把它们加在一起(0 到 4 + 1 到 4 + 2 到 4 + 3 到 4 + 4)。 所以这种情况下的结果是 145。到目前为止,我只能使用以下代码对整个数组求和:
public static int Sums(int[] array1, int length)
{
if (length <= 0)
{
return 0;
}
else
{
return Sums(array1, (length - 1)) + array1[length - 1];
}
}
我想我会先尝试得到整个数组的总和,但现在我被卡住了,我不知道如何继续。 我如何获得其他总和并将它们全部相加? 我什至不确定我是否从右脚开始。
传递数组中的起始索引而不是长度,它更简单。 在您的代码中,您实际上也没有将数组从开始索引到数组末尾求和。
public int sum_array(int[] arr, int start_index = 0)
{
//stop recursion once you've reached the end of the array
if (start_index >= arr.Length)
{
return 0;
}
else
{
//sum the array from the starting element to the end of the array
int sum = 0;
for (int i = start_index; i < arr.Length; i++)
sum += arr[i];
//add that sum to the sum of the result of the next recursive call
return sum + sum_array(arr, start_index+1);
}
}
编辑:
我突然想到你可能一直在询问一次递归一个元素。 我会为索引添加一个额外的参数。 因此,您将拥有 start_index,它本质上是定义数组的子数组。 然后你有索引参数,它跟踪你在那个子数组中的位置。
public int sum_array_2(int[] arr, int start_index = 0, int index = 0)
{
if (start_index >= arr.Length)
{
return 0;
}
else
{
//increment to the next element in the array
if (index < arr.Length - 1)
return arr[index] + sum_array_2(arr, start_index, index + 1);
//increment the start index and set the current index to the start index
return arr[index] + sum_array_2(arr, start_index + 1, start_index + 1);
}
}
static void Main(string[] args)
{
Console.Write(ArraySum(arr, arr.Length));
Console.ReadLine();
}
static int[] arr = { 10, 20, 30, 40, 50 };
static int ArraySum(int[] arr, int N)
{
if (N <= 0)
return 0;
return (ArraySum(arr, N - 1) + arr[N - 1]);
}
创建一个 for 循环,查看数组中的每个元素,然后使用嵌套的 for 循环将初始循环所在元素的所有元素相加。
public static int Sums(int[] array1){
int sum = 0;
for(int i = 0; i < array1.Length; i++)
{
for (int j = i; j < array1.Length; j++)
sum += array1[j];
}
return sum;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.