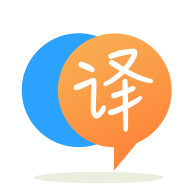
[英]How can we Concatenate vector elements before specific separator and make new vector in Rust
[英]How can I concatenate two `&str` type elements of Rust Vector and add to Zero Index
let mut item = vec!["2", "3", "5"];
我想连接这个向量的第一个和第二个索引并用零索引的值替换。
item[0] = item[0] + item[1];
但是由于向量元素的类型是&str
并且我在连接后得到的结果是String
,Rust 不允许我更新向量的值。
获得结果的一种方法是通过编译器驱动开发。
从...开始:
fn main() {
let mut item = vec!["2","3","5"];
let first_item = &(item[0].to_owned() + item[1]);
item[0] = item[0] + item[1];
println!("item {:?}", item);
}
我们有:
error[E0369]: binary operation `+` cannot be applied to type `&str`
--> src/main.rs:6:22
|
6 | item[0] = item[0] + item[1];
| ------- ^ ------- &str
| | |
| | `+` cannot be used to concatenate two `&str` strings
| &str
|
help: `to_owned()` can be used to create an owned `String` from a string reference. String concatenation appends the string on the right to the string on the left and may require reallocation. This requires ownership of the string on the left
|
6 | item[0] = item[0].to_owned() + item[1];
| ^^^^^^^^^^^^^^^^^^
按照编译器的建议:
item[0] = item[0].to_owned() + item[1];
现在我们得到:
|
6 | item[0] = item[0].to_owned() + item[1];
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^
| |
| expected `&str`, found struct `std::string::String`
| help: consider borrowing here: `&(item[0].to_owned() + item[1])`
然后再次应用该建议:
|
6 | item[0] = &(item[0].to_owned() + item[1]);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^- temporary value is freed at the end of this statement
| |
| creates a temporary which is freed while still in use
7 |
8 | println!("item {:?}", item);
| ---- borrow later used here
现在问题与生命周期范围有关,按照编译器的建议,这是最终版本:
fn main() {
let mut item = vec!["2","3","5"];
let first_item = &(item[0].to_owned() + item[1]);
item[0] = first_item;
println!("item {:?}", item);
}
编译器已经找到了解决方案,但在每种情况下,您都必须仔细考虑该解决方案是否满足您的应用程序的要求。 在这种情况下,您必须注意生命周期范围。
正如已经建议的那样,另一个更惯用的解决方案可能是使用字符串的 Vec:
let mut item: Vec<String> = vec!["2".to_owned(), "3".to_owned(), "5".to_owned()];
item[0] = format!("{}{}", item[0], item[1]);
假设您想在运行时执行此操作,这是不可能的。 &str
是非拥有类型,不能将两个&str
连接成一个,因为它们指向的存储可能是非连续的。
您可以使用String
实现目标:
let mut item = vec![String::from("2"), String::from("3"), String::from("5")];
// Vec is not empty and contains more than 1 element, it is safe to unwrap
let (first, rest) = item.split_first_mut().unwrap();
// Append the second element to the end of the first element
first.push_str(rest[0].as_str());
println!("{}", &first); // 23
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.