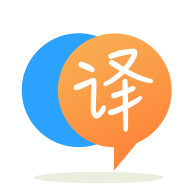
[英]PHP Array filter and create new array based on the result of filtered array
[英]Filter result array based on date filter in PHP
我有一个从 mysql 数据库中获取的结果数组。 我想根据日期对这些数据进行分组,如下所示:
输入数组:
$resultSet = [
0 => [
'id' => 123,
'name' => 'ABC',
'start_date' => '2019-12-18 20:00:00'
],
1 => [
'id' => 124,
'name' => 'CDE',
'start_date' => '2019-12-19 20:00:00'
],
2 => [
'id' => 125,
'name' => 'TEST',
'start_date' => '2019-12-23 20:00:00'
],
3 => [
'id' => 126,
'name' => 'BWM',
'start_date' => '2019-12-18 20:00:00'
],
4 => [
'id' => 127,
'name' => 'XYZ',
'start_date' => '2019-12-19 20:00:00'
],
5 => [
'id' => 128,
'name' => 'GHJ',
'start_date' => '2019-12-21 20:00:00'
],
6 => [
'id' => 129,
'name' => 'GHJK',
'start_date' => '2019-12-22 20:00:00'
],
7 => [
'id' => 130,
'name' => 'GHL',
'start_date' => '2019-12-20 20:00:00'
],
8 => [
'id' => 131,
'name' => 'JKL',
'start_date' => '2019-12-25 20:00:00'
]
];
输出:按过滤器(今天、明天、本周末、下周末等)显示所有产品列表。假设我们在星期三运行脚本。
今天:只显示索引的记录:0, 3(今天的日期:2019-12-18)
明天:仅显示索引的记录:0、3(明天日期:2019-12-19)
本周末:只显示第5、6、7的记录(周五、周六、周日:2019-12-20、2019-12-21、2019-12-22)
Next Week : 只显示索引的记录: 2, 8 (日期: 2019-12-23, 2019-12-25)
结果将动态显示,每周不同的记录将显示如上。
使用的示例代码:
$arrayList = [];
foreach($resultSet as $element){
$arrayList[$element->start_date][] = $element;
}
dd($arrayList);
需要更改上面的代码以按照下面的要求获取结果。
框架:Laravel 5.2
要求:
预期输出:
$output = [
'today' => [
0 => [
'id' => 123,
'name' => 'ABC',
'start_date' => '2019-12-18 20:00:00'
],
1 => [
'id' => 126,
'name' => 'BWM',
'start_date' => '2019-12-18 20:00:00'
]
],
'tomorrow' => [
0 => [
'id' => 124,
'name' => 'CDE',
'start_date' => '2019-12-19 20:00:00'
],
1 => [
'id' => 127,
'name' => 'XYZ',
'start_date' => '2019-12-19 20:00:00'
]
],
'this_weekend' => [
0 => [
'id' => 130,
'name' => 'GHL',
'start_date' => '2019-12-20 20:00:00'
],
1 => [
'id' => 128,
'name' => 'GHJ',
'start_date' => '2019-12-21 20:00:00'
],
2 => [
'id' => 129,
'name' => 'GHJK',
'start_date' => '2019-12-22 20:00:00'
]
],
'next_week' => [
0 => [
'id' => 125,
'name' => 'TEST',
'start_date' => '2019-12-23 20:00:00'
],
1 => [
'id' => 131,
'name' => 'JKL',
'start_date' => '2019-12-25 20:00:00'
]
]
];
我在视图页面中运行简单的 foreach 循环来显示数据。 如果有任何疑问,请随时在下面发表评论。
一开始你应该定义一个有用的日期格式来进一步比较日期:
$s = new DateTime(); // create date value
$s->modify('+2 days'); // in fact today is 2019-12-16
// but you've choose 2019-12-18
// you can comment this line
//echo $s->format('Y-m-d H:i:s').PHP_EOL; // today
$today = $s->format('Ymd'); // format 20191218
echo $today.' <- today'.PHP_EOL; // today
$nextmonday = $s->modify('next monday')->format('Ymd');
echo $nextmonday.' <- next monday'.PHP_EOL.PHP_EOL; // next monday, format 20191223
然后你需要做一个像这样的foreach
循环:
foreach($resultSet as $rec){
$d = date('Ymd', strtotime($rec['start_date'])); // transform to format 20191218
$d2 = date('l', strtotime($rec['start_date'])); // name of the day
echo $d.' --- '.$d2.PHP_EOL;
if ($d == $today) { // 2019-12-18 == 2019-12-18
$res['today'][] = $rec;
} else if ($d - $today == 1) { // 2019-12-19 - 2019-12-18 == 1
$res['tomorrow'][] = $rec;
} else if (in_array($d2,['Friday','Saturday','Sunday']) && $d < $nextmonday){ // < 2019-12-23 and by day name
$res['this_weekend'][] = $rec;
} else if ($d >= $nextmonday){ // next week (not a weekend)
$res['next_weekend'][] = $rec;
}
}
输出将是:
Array
(
[today] => Array
(
[0] => Array
(
[id] => 123
[name] => ABC
[start_date] => 2019-12-18 20:00:00
)
[1] => Array
(
[id] => 126
[name] => BWM
[start_date] => 2019-12-18 20:00:00
)
)
[tomorrow] => Array
(
[0] => Array
(
[id] => 124
[name] => CDE
[start_date] => 2019-12-19 20:00:00
)
[1] => Array
(
[id] => 127
[name] => XYZ
[start_date] => 2019-12-19 20:00:00
)
)
[next_weekend] => Array
(
[0] => Array
(
[id] => 125
[name] => TEST
[start_date] => 2019-12-23 20:00:00
)
[1] => Array
(
[id] => 131
[name] => JKL
[start_date] => 2019-12-25 20:00:00
)
)
[this_weekend] => Array
(
[0] => Array
(
[id] => 128
[name] => GHJ
[start_date] => 2019-12-21 20:00:00
)
[1] => Array
(
[id] => 129
[name] => GHJK
[start_date] => 2019-12-22 20:00:00
)
[2] => Array
(
[id] => 130
[name] => GHL
[start_date] => 2019-12-20 20:00:00
)
)
)
注意:最后一个名为next_weekend
数组,也许您需要将其重命名为next_week
,因为它的日期正好在您当前的周末之后。
我认为您已经理解了一个想法,并且能够添加您的进一步逻辑。
好吧,如果您正在使用 laravel,那么您可以使用 carbon 和 collection 方法的力量来解决此类问题。
您可以使用 carbon 方法映射数组键并对其进行分组。 检查以下片段将为您提供确切的结果。
date_default_timezone_set("Asia/Kolkata");
$resultSet = [
0 => [
'id' => 127,
'name' => 'XYZ',
'start_date' => '2020-01-03 20:00:00'
],
1 => [
'id' => 128,
'name' => 'GHJ',
'start_date' => '2020-01-04 20:00:00'
],
2 => [
'id' => 129,
'name' => 'GHJK',
'start_date' => '2020-01-05 16:00:00'
],
3 => [
'id' => 130,
'name' => 'GHL',
'start_date' => '2020-01-05 20:00:00'
],
4 => [
'id' => 131,
'name' => 'JKL',
'start_date' => '2020-01-18 20:00:00'
]
];
如果您希望将来的日期在“in_future”键中。
$output = collect($resultSet)->map(function ($value) {
$group_by_key = Carbon::parse($value['start_date']);
switch ($group_by_key) {
case $group_by_key->isToday():
$group_by_key = 'today';
break;
case $group_by_key->isTomorrow():
$group_by_key = 'tomorrow';
break;
case $group_by_key->isNextWeek():
$group_by_key = 'next_week';
break;
case $group_by_key->weekOfYear > Carbon::now()->addWeek()->weekOfYear:
$group_by_key = 'in_future';
break;
case $group_by_key->isWeekend():
$group_by_key = 'this_weekend';
break;
default:
$group_by_key = $group_by_key->format('l');;
break;
}
$value['group_by_key'] = $group_by_key;
return $value;
})->groupBy('group_by_key');
var_dump($output);
或者,如果您希望将来的日期在“next_week”键中。
$output = collect($resultSet)->map(function ($value) {
$group_by_key = Carbon::parse($value['start_date']);
switch ($group_by_key) {
case $group_by_key->isToday():
$group_by_key = 'today';
break;
case $group_by_key->isTomorrow():
$group_by_key = 'tomorrow';
break;
case $group_by_key->weekOfYear > Carbon::now()->addWeek()->weekOfYear:
$group_by_key = 'next_week';
break;
case $group_by_key->isWeekend():
$group_by_key = 'this_weekend';
break;
default:
$group_by_key = $group_by_key->format('l');;
break;
}
$value['group_by_key'] = $group_by_key;
return $value;
})->groupBy('group_by_key');
var_dump($output);
试试下面的例子
$group = [];
foreach ($start_date as $item) {
if (!isset($group[$item['start_date']])) {
$group[$item['start_date']] = [];
}
foreach ($item as $key => $value) {
if ($key == 'start_date') continue;
$group[$item['start_date']][$key] = $value;
}
}
我认为你应该制作不同日期的数组:今天,明天,这个周末,下周
检查时间格式的链接: https : //carbon.nesbot.com/docs/
$now = Carbon::now();
echo $now; // 2019-11-20 16:41:03
echo "\n";
$today = Carbon::today();
echo $today; // 2019-11-20 00:00:00
echo "\n";
$tomorrow = Carbon::tomorrow('Europe/London');
echo $tomorrow; // 2019-11-21 00:00:00
echo "\n";
用日期的比较条件检查您的结果并制作数组并将它们组合成一个数组。
$totalarr = array('today'=>$todayarr,'tomorrow'=>$tomorrowarr,'thisweek'=>$thisweek,'nextweek'=>$nextweekarr);
print_r($totalarr);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.