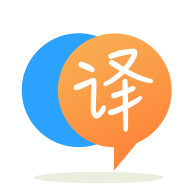
[英]how to iterate using itertools for iterables having different lengths in python?
[英]How to iterate with range using itertools?
考虑其中的以下列表 4 个元组:
players_score = [ ('Joe', 100, 34, 38, 90, 67, 3, 10),
('Bob', 90, 38, 4, 100, 60, 4, 11),
('May', 80, 36, 40, 91, 70, 2, 12),
('Anna', 95, 32, 36, 92, 68, 8, 13) ]
球员们已经打了7场比赛。 在第一场比赛中,乔以 100 分获胜。
我想根据以下内容为每个玩家(每场比赛)评分:
First/best player: 5 points
Second player: 3 points
Third player: 1 point
Fourth player: -1 point -> But 0 points is what I like to assign the fourth player of each game.
到目前为止我的代码:
from itertools import zip_longest as y
zipped = list(y(*[game_score for _, *game_score in players_score]))
tup = list(sorted(i, reverse=True) for i in zipped)
score_dict = {}
for player, *game_score in players_score:
tmp = []
for i,j in zip(game_score, tup):
tmp.append(5-2*j.index(i))
tot_score = sum(tmp)
score_dict[player] = tot_score
print("The overall scores are: ", sorted(score_dict.items(), key=lambda kv: (kv[1], kv[0]), reverse=True))
因此,我的代码将 -1 分应用于每场比赛的第四名玩家,但相反,我希望第四名玩家仅获得 0 分。 我正在努力应用范围 ([0:3]) 或另一种方法,通过这种方法我可以跳过第四名玩家获得分数,因为该玩家只获得了 0 分。
您可以更改评分函数:
tmp.append(5-2*j.index(i) if 5-2*j.index(i) > 0 else 0)
所以如果有负值,则将分数设置为 0
一个非常简单的解决方案
for i,j in zip(game_score, tup):
score_var = 5-2*j.index(i)
if (score_var < 0):
score_var = 0
tmp.append(score_var)
这将确保没有玩家会获得负分。
使用max(0, x)
确保该值至少为0
:
tmp.append(max(0, 5-2*j.index(i)))
我会稍微设置一下。 话虽如此,有不止一种方法可以做到这一点。 我要做的第一件事就是把这个players_score
列表变成一个更漂亮的集合,就像一本字典——这样工作起来会更有趣。
基本上,我们遍历我们的“回合”(游戏),并根据他们在当前回合中的得分(当前回合的获胜者在前)对玩家姓名进行排序。 然后我们用他们应得的相应奖励(分数) zip
排序后的玩家名称,并使用玩家名称作为键更新我们的collections.Counter
集合。
from collections import Counter
players_score = [
("Joe", 100, 34, 38, 90, 67, 3, 10),
("Bob", 90, 38, 4, 100, 60, 4, 11),
("May", 80, 36, 40, 91, 70, 2, 12),
("Anna", 95, 32, 36, 92, 68, 8, 13)
]
original_scores = {player: scores for player, *scores in players_score}
def iter_score(player):
for score in original_scores[player]:
yield player, score
score_rewards = [5, 3, 1, 0]
overall_scores = Counter()
for current_round in zip(*map(iter_score, original_scores)):
sorted_players = map(lambda tpl: tpl[0], sorted(current_round, key=lambda tpl: tpl[1], reverse=True))
for player, score in zip(sorted_players, score_rewards):
overall_scores.update({player: score})
print("The overall scores:")
for player, score in overall_scores.most_common():
print(f"{player}: {score}")
输出:
The overall scores:
Anna: 20
May: 17
Bob: 15
Joe: 11
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.