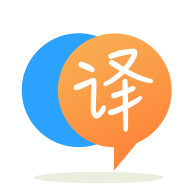
[英]typeerror slice indices must be integers or none or have an __index__ method
[英]Use strings as slice indices in Python ? (TypeError: slice indices must be integers or None or have an __index__ method)
我有一个排序数组:
arr = ['Alexander', 'Belman', 'Erik', 'Nicholas', ... , 'Zahir']
我想做这样的事情:
arr['B':'M'] # ['Belman', 'Erik']
我如何创建一个类并实现
__getitem__
__index__
以正确的方式实现这一目标?
我正在考虑使用类似的东西
def __getitem__(self, key):
if isinstance(key, slice):
return [self.list[i] for i in range(key.start, key.stop)]
return self.list[key]
但我不知道如何索引字符串。 我怎样才能创建一个
__index__
将 binarySearch 应用于self.list
并返回正确索引的方法?
我认为您可以通过如下简单的实现来逃脱:
from collections import UserList
class MyList(UserList):
def __getitem__(self, key):
if isinstance(key, slice):
return [e for e in self.data if key.start <= e < key.stop]
# TODO implement the rest of the usecases and/or error handling...
# for now slicing with integers will miserably fail,
# and basic integer indexing returns None
arr = MyList(['Alexander', 'Belman', 'Erik', 'Nicholas', 'Zahir'])
print(arr['B':'M'])
这将输出
['Belman', 'Erik']
同样地,
print(arr['Alex':'Er'])
会输出
['Alexander', 'Belman']
请注意,我使用key.start <= e < key.stop
来符合在整个 python 中使用的 inclusive:exclusive ( [)
) 行为。
另请注意,我仅实现了字符串切片用例。 您可以根据需要实施其他用例和错误处理。
我还使用 numpy 通过二分搜索发布了我的解决方案
class Stock():
def __init__(self, name, date):
self.name = name
self.date = np.array(date)
def __getitem__(self, key):
if isinstance(key, slice):
if key.start is not None and key.stop is not None:
return self.date[np.searchsorted(self.date, key.start, side='left', sorter=None):np.searchsorted(self.date, key.stop, side='left', sorter=None)]
elif key.start is not None:
return self.date[np.searchsorted(self.date, key.start, side='left', sorter=None):]
elif key.stop is not None:
return self.date[:np.searchsorted(self.date, key.stop, side='left', sorter=None)]
else:
return self.date[:]
i = np.searchsorted(self.date, key, side='left', sorter=None)
if key != self.date[i]:
raise KeyError('key: {} was not found!'.format(key))
else:
return self.date[i]
aapl = Stock('aapl', ['2010','2012', '2014', '2016', '2018'])
print(aapl['2011':])
print(aapl['2014':'2017'])
print(aapl[:'2016'])
print(aapl['2010'])
print(aapl['2013'])
'''
['2012' '2014' '2016' '2018']
['2014' '2016']
['2010' '2012' '2014']
2010
Traceback (most recent call last):
File "C:\Users\...\Desktop\...\stock.py", line ##, in <module>
print(aapl['2013'])
File "C:\Users\...\Desktop\...\stock.py", line ##, in __getitem__
raise KeyError('key: {} was not found!'.format(key))
KeyError: 'key: 2013 was not found!'
'''
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.