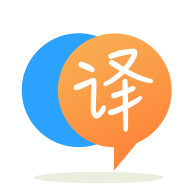
[英]How can I allow a user to become an actor of a service account in Google Cloud Platform (GCP)?
[英]How I can get access to GCP cloud function from python code using service account?
我部署了一个简单的 GCP Cloud Function,它返回“Hello World.”。 我需要这个 function 获得授权,我取消选中“允许未经身份验证的调用”复选框。 所以只有经过身份验证的调用才能调用此代码:我还创建了服务帐户并赋予了下一个角色:- Cloud Functions Invoker - Cloud Functions Service Agent
我的代码:
from google.oauth2 import service_account
from google.auth.transport.urllib3 import AuthorizedHttp
if __name__ == '__main__':
credentials = service_account.Credentials.from_service_account_file('service-account.json',
scopes=['https://www.googleapis.com/auth/cloud-platform'],
subject='service-acc@<project_id>.iam.gserviceaccount.com')
authed_session = AuthorizedHttp(credentials)
response = authed_session.urlopen('POST', 'https://us-central1-<project_id>.cloudfunctions.net/main')
print(response.data)
我得到了回应:
b'\n<html><head>\n<meta http-equiv="content-type" content="text/html;charset=utf-8">\n<title>401 Unauthorized</title>\n</head>\n<body text=#000000 bgcolor=#ffffff>\n<h1>Error: Unauthorized</h1>\n<h2>Your client does not have permission to the requested URL <code>/main</code>.</h2>\n<h2></h2>\n</body></html>\n'
如何获得授权?
您的示例代码正在生成访问令牌。 下面是一个生成身份令牌并使用该令牌调用 Cloud Functions 端点的真实示例。 该函数需要具有用于授权的服务帐户的 Cloud Function Invoker 角色。
import json
import base64
import requests
import google.auth.transport.requests
from google.oauth2.service_account import IDTokenCredentials
# The service account JSON key file to use to create the Identity Token
sa_filename = 'service-account.json'
# Endpoint to call
endpoint = 'https://us-east1-replace_with_project_id.cloudfunctions.net/main'
# The audience that this ID token is intended for (example Google Cloud Functions service URL)
aud = 'https://us-east1-replace_with_project_id.cloudfunctions.net/main'
def invoke_endpoint(url, id_token):
headers = {'Authorization': 'Bearer ' + id_token}
r = requests.get(url, headers=headers)
if r.status_code != 200:
print('Calling endpoint failed')
print('HTTP Status Code:', r.status_code)
print(r.content)
return None
return r.content.decode('utf-8')
if __name__ == '__main__':
credentials = IDTokenCredentials.from_service_account_file(
sa_filename,
target_audience=aud)
request = google.auth.transport.requests.Request()
credentials.refresh(request)
# This is debug code to show how to decode Identity Token
# print('Decoded Identity Token:')
# print_jwt(credentials.token.encode())
response = invoke_endpoint(endpoint, credentials.token)
if response is not None:
print(response)
您需要获得身份令牌才能 (http) 触发您的云 function 和
您的服务帐户至少需要具有 Cloud Functions Invoker 角色。
(为了尽可能安全,创建一个只能调用云函数的服务帐户,不能调用其他任何东西 -> 以避免在您的服务帐户密钥文件落入坏人之手时出现问题。)
然后你可以运行以下命令:
import os
import google.oauth2.id_token
import google.auth.transport.requests
import requests
os.environ['GOOGLE_APPLICATION_CREDENTIALS'] = 'service_account_key_file.json'
google_auth_request = google.auth.transport.requests.Request()
cloud_functions_url = 'https://europe-west1-your_project_id.cloudfunctions.net/your_function_name'
id_token = google.oauth2.id_token.fetch_id_token(
google_auth_request, cloud_functions_url)
headers = {'Authorization': f'Bearer {id_token}'}
response = requests.get(cloud_functions_url, headers=headers)
print(response.content)
这个问题+答案也帮助了我:
如何检索 id_token 以访问 Google Cloud Function?
如果您需要通过 python 获取访问令牌(而不是身份令牌),请在此处查看:
如何使用 python 以编程方式获取 GCP Bearer 令牌
错误是因为调用未经过身份验证。
由于现在调用来自您的本地主机,这应该对您有用。
curl https://REGION-PROJECT_ID.cloudfunctions.net/FUNCTION_NAME \
-H "Authorization: bearer $(gcloud auth print-identity-token)"
这将使用您在本地主机上在 gcloud 上活动的帐户进行调用。
进行调用的帐户必须具有Cloud Functions Invoker角色
将来只需确保调用此函数的服务具有提到的角色。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.