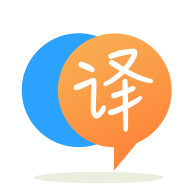
[英]Calling a python method to fetch data, from a javascript function on the html page
[英]How to access function inside a javascript fetch from html page
我正在尝试根据一些用户上传的 json 文件制作一个显示图表的网站。 我正在使用 fetch 从 servlet 获取 JSON 数据,并在 fetch 中制作图形,这很好用。 只有我想做一个 addData() 函数来向同一个图中添加额外的数据。 问题是我无法访问 fetch 请求之外的数据,并且如果它在我的 javascript 文件中的 fetch 中,我无法从我的 html 页面访问 addData() 函数。 有没有解决这个问题的好方法?
我的 JavaScript 代码:
fetch('/json_storage')
.then((response) => {
return response.json();
})
.then((myJson) => {
let hrdata = myJson[0].heartRate;
let timestamp = hrdata.map(t => { return t.timestamp });
let bpm = hrdata.map( b => {return b.bpm});
let ctx = document.getElementById('canvas').getContext('2d');
let canvas = new Chart(ctx, {
type: 'line',
data: {
labels: timestamp,
datasets: [{
label: new Date(timestamp[0]),
data: bpm,
backgroundColor: 'rgba(255,0,55,0.25)',
pointRadius: 0
}]
},
options: {
title: {
display: true,
text: 'The course of your heart rate during the day'
},
scales: {
xAxes: [{
type: 'time',
time: {
unit: 'hour'
}
}]
}
}
});
function addData() {
for (let i =1; i < myJson.length; i++){
let hrdata = myJson[i].heartRate;
let bpm = hrdata.map( b => {return b.bpm});
let timestamp = hrdata.map(t => { return t.timestamp });
canvas.data.data = bpm;
canvas.data.labels = timestamp;
canvas.update();
}
}
});
和我的 HTML 代码:
<button id="addData">Add dataset</button>
<canvas id="canvas" width="300" height="300"></canvas>
在你的代码中, addData
和myJson
的作用域你的诺言的回调,这是不可用之外里面-无论你需要公开这些对全球范围内或写一个可重复使用的功能暴露(回报)的addData
。
我将您的 fetch 函数修改为一个可重用的类,您可以在其中访问其上下文中的所有数据。
class LineChart { constructor(ctx, dataUrl, initialData = []) { this.ctx = ctx; this.data = initialData; this.dataUrl = dataUrl; this.canvas = null; this.fetchData(); } fetchData = () => fetch(this.dataUrl) .then((response) => { return response.json(); }) .then((myJson) => { this.data = myJson; this.draw(); return this.data; }); draw = () => { if (!this.ctx) { return false; } let hrdata = this.data[0].heartRate; let timestamp = hrdata.map(t => { return t.timestamp }); let bpm = hrdata.map(b => { return b.bpm }); this.canvas = new Chart(this.ctx, { type: 'line', data: { labels: timestamp, datasets: [{ label: new Date(timestamp[0]), data: bpm, backgroundColor: 'rgba(255,0,55,0.25)', pointRadius: 0 }] }, options: { title: { display: true, text: 'The course of your heart rate during the day' }, scales: { xAxes: [{ type: 'time', time: { unit: 'hour' } }] } } }); } addData = () => { alert(`in addData function ${JSON.stringify(this.data)}`); for (let i = 1; i < this.data.length; i++) { let hrdata = this.data[i].heartRate; let bpm = hrdata.map(b => { return b.bpm }); let timestamp = hrdata.map(t => { return t.timestamp }); this.canvas.data.data = bpm; this.canvas.data.labels = timestamp; this.canvas.update(); } } } //usage const chart = new LineChart( document.getElementById('canvas').getContext('2d'), '/json_storage' ); document.getElementById('addData').onclick = chart.addData;
<button id="addData">Add dataset</button> <canvas id="canvas" width="300" height="300"></canvas>
要执行您想要的操作,您需要将迄今为止构建的数据存储在页面中持久化的数据结构中。 如果您使用的是像React
或Angular
这样的 UI 框架,那么您可以将其存储为状态的一部分,然后当有人单击“添加数据”按钮时,调用一个函数来相应地修改该状态数据并重新呈现结果。
另一种方法是重新获取数据并将其与添加的数据组合,但这样您将丢失任何先前添加的数据。
如果您没有使用 UI 框架,您是否尝试过将获取的数据简单地保存在 javascript 文件顶部定义的变量中? 获取后,将其设置在该变量中并具有单独生成图表的函数。 每次单击“添加数据”按钮时,应先将数据添加到之前保存的数据中,并根据新数据集重新绘制图表。
您需要将数据的存储和canvas
与数据的处理分开,以便在添加新数据时,可以更新canvas
,但这超出了您所问问题的范围,但也许这样的事情是可行的:
const allJson = [];
const ctx = document.getElementById("canvas").getContext("2d");
const canvas = new Chart(ctx, {
type: "line",
data: {
labels: timestamp,
datasets: [
{
label: new Date(timestamp[0]),
data: bpm,
backgroundColor: "rgba(255,0,55,0.25)",
pointRadius: 0
}
]
},
options: {
title: {
display: true,
text: "The course of your heart rate during the day"
},
scales: {
xAxes: [
{
type: "time",
time: {
unit: "hour"
}
}
]
}
}
});
fetch("/json_storage")
.then(response => response.json())
.then(myJson => {
addData(myJson[0]);
});
function addData(newJson) {
allJson.push(newJson);
let bpm = [];
let timestamp = [];
for (let i = 0; i < allJson.length; i++) {
let hrdata = myJson[i].heartRate;
bpm = bpm.concat(hrdata.map(b => b.bpm));
timestamp = timestamp.concat(hrdata.map(t => t.timestamp));
}
canvas.data.data = bpm;
canvas.data.labels = timestamp;
canvas.update();
}
请注意,我只是在猜测为图表准备数据的正确方法,因为我不知道来自fetch
的数据的确切形式以及它们的结构。 但总体思路是:
addData
设置它
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.