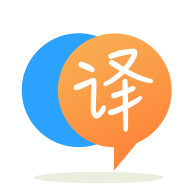
[英]How can I stub a constructor in node.js using sinon in a Cloud Functions for Firebase project?
[英]how can i add the sendgrid webhook event Json response in a firebase cloud firestore using node.js
我不知道如何实现这个东西,但在此之前,我已经完成了 SendGrid 的一部分,其中创建了任何文档,然后它将电子邮件发送给用户。 但这部分我要问的是我不知道如何继续。这是我这个实现的第一部分,其中如果创建了新记录,则任何集合都会向特定电子邮件发送电子邮件,并且有一个名为事件对象 I 的响应想写一个云函数来存储数据。 我不知道如何启动此功能或继续解决此问题。
"use strict";
const functions = require("firebase-functions");
const admin = require("firebase-admin");
var serviceAccount1 = require("./key.json");
const newProject = admin.initializeApp({
credential: admin.credential.cert(serviceAccount1),
databaseURL: "xyz"
});
const sgMail = require("@sendgrid/mail");
const sgMailKey = "key";
sgMail.setApiKey(sgMailKey);
exports.sentMail = functions.firestore
.document("/Offices/{officeId}")
.onCreate((documentSnapshot,event) => {
const documentData = documentSnapshot.data()
const officeID = event.params.officeId;
console.log(JSON.stringify(event))
const db = newProject.firestore();
return db.collection("Offices").doc(officeID).get()
.then(doc => {
const data = doc.data();
const msg = {
to: "amarjeetkumars34@gmail.com",
from: "singhamarjeet045@gmail.com",
text: "hello from this side",
templateId: "d-8ecfa59aa9d2434eb8b7d47d58b4f2cf",
substitutionWrappers: ["{{", "}}"],
substitutions: {
name: data.name
}
};
return sgMail.send(msg);
})
.then(() => console.log("payment mail sent success"))
.catch(err => console.log(err));
});
我的问题的预期输出就像一个集合名称 XYZ,其中一个对象有三个字段,例如
{email:"xyz@gmail.com",
event:"processed",
timestamp:123555558855},
{email:"xyz@gmail.com",
event:"recieved",
timestamp:123555558855},
{email:"xyz@gmail.com",
event:"open",
timestamp:123555558855}
正如您将在Sendgrid 文档中读到的:
SendGrid 的事件 Webhook 将通过 HTTP POST 通知您选择的 URL,其中包含有关在 SendGrid 处理您的电子邮件时发生的事件的信息
要在 Firebase 项目中实现 HTTP 端点,您将实现一个HTTPS 云函数,该函数将由 Sendgrid Webhook 通过 HTTPS POST 请求调用。
来自 Sendgrid webhook 的每个调用都将涉及一个特定的事件,您将能够在您的云函数中获取事件的值(已processed
、已delivered
等...)。
现在,您需要在您的 Cloud Function 中将特定事件与之前通过您的 Cloud Function 发送的特定电子邮件相关联。 为此,您应该使用自定义参数。
更准确地说,您将向您的msg
对象(您传递给send()
方法)添加一个唯一标识符。 经典值是 Firestore 文档 ID,例如event.params.officeId
但可以是您在 Cloud Function 中生成的任何其他唯一 ID。
在发送电子邮件的云功能,通过officeId
在custom_args
对象,如下图所示:
exports.sentMail = functions.firestore
.document("/Offices/{officeId}")
.onCreate((documentSnapshot,event) => {
const documentData = documentSnapshot.data();
const officeId = event.params.officeId;
const msg = {
to: "amarjeetkumars34@gmail.com",
from: "singhamarjeet045@gmail.com",
text: "hello from this side",
templateId: "d-8ecfa59aa9d2434eb8b7d47d58b4f2cf",
substitutionWrappers: ["{{", "}}"],
substitutions: {
name: documentData.name
},
custom_args: {
"officeId": officeId
}
};
return sgMail.send(msg)
.then(() => {
console.log("payment mail sent success"));
return null;
})
.catch(err => {
console.log(err)
return null;
});
});
请注意,您通过documentSnapshot.data()
获取新创建的文档(触发 Cloud Function 的documentSnapshot.data()
:您不需要在 Cloud Function 中查询相同的文档。
然后,创建一个简单的HTTPS Cloud Function ,如下所示:
exports.sendgridWebhook = functions.https.onRequest((req, res) => {
const body = req.body; //body is an array of JavaScript objects
const promises = [];
body.forEach(elem => {
const event = elem.event;
const eventTimestamp = elem.timestamp;
const officeId = elem.officeId;
const updateObj = {};
updateObj[event] = true;
updateObj[event + 'Timestamp'] = eventTimestamp;
promises.push(admin.firestore().collection('Offices').doc(officeId).update(updateObj));
});
return Promise.all(promises)
.then(() => {
return res.status(200).end();
})
})
部署它并获取它在终端中显示的 URL:它应该像https://us-central1-<your-project-id>.cloudfunctions.net/sendgridWebhook
。
请注意,这里我使用admin.firestore().collection('Offices')...
。 您可以使用const db = newProject.firestore(); ... db.collection('Offices')...
const db = newProject.firestore(); ... db.collection('Offices')...
还要注意,Sendgrid webhook 发送的 HTTPS POST 请求的主体包含一个 JavaScript 对象数组,因此我们将使用Promise.all()
来处理这些不同的对象,即使用officeId
将不同的事件写入 Firestore 文档。
然后,您需要在 Sendgrid 平台中的“邮件设置/事件通知”部分中设置 Webhook,如文档中所述,如下所示。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.