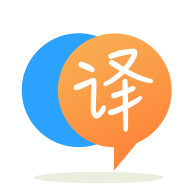
[英]Trouble using ScreenManager to display two screens with Kivy & Python. Getting a black screen
[英]Kivy ScreenManager gives me a black screen
我一直在尝试使用 kivy 创建一个乒乓球游戏。 我按照他们网站上的教程进行操作,效果很好! 但是我想添加一个开始屏幕。 我在谷歌上查看,发现我应该使用 Kivy 的屏幕管理器。 我把它放在我的代码中,但是当我按下开始按钮时,它什么也不做,给我一个黑屏。 我一直在试图弄清楚,但我不明白有什么问题! 可能是因为我对 Kivy 没有太多经验。 这是我的代码。
主文件
from kivy.app import App
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty
from kivy.vector import Vector
from kivy.clock import Clock
from kivy.uix.button import Button
from kivy.uix.screenmanager import ScreenManager, Screen, SlideTransition
class PingPongPaddle(Widget):
score = NumericProperty(0)
def bounce_ball(self, ball):
if self.collide_widget(ball):
vx, vy = ball.velocity
offset = (ball.center_y - self.center_y) / (self.height / 2)
bounced = Vector(-1 * vx, vy)
vel = bounced * 1.1
ball.velocity = vel.x, vel.y + offset
class PingPongBall(Widget):
#Velocity on x and y axis
velocity_x = NumericProperty(0)
velocity_y = NumericProperty(0)
#So ball.velocity can be used
velocity = ReferenceListProperty(velocity_x, velocity_y)
#Moves the ball one step
def move(self):
self.pos = Vector(*self.velocity) + self.pos
class PingPongGame(Widget):
ball = ObjectProperty(None)
player1 = ObjectProperty(None)
player2 = ObjectProperty(None)
def serve_ball(self, vel=(8, 0)):
self.ball.center = self.center
self.ball.velocity = vel
def update(self, dt):
self.ball.move()
#Bounce off the paddles
self.player1.bounce_ball(self.ball)
self.player2.bounce_ball(self.ball)
#Bounce off top and bottom
if (self.ball.y < 0) or (self.ball.top > self.height):
self.ball.velocity_y *= -1
#Went off the side
if self.ball.x < self.x:
self.player2.score += 1
self.serve_ball(vel=(4, 0))
if self.ball.x > self.width:
self.player1.score += 1
self.serve_ball(vel=(-4, 0))
def on_touch_move(self, touch):
#To sense if screen is touched
if touch.x < self.width / 3:
self.player1.center_y = touch.y
if touch.x > self.width - self.width / 3:
self.player2.center_y = touch.y
class GameScreen(Screen):
def play(self):
global game
game = PingPongGame()
game.serve_ball()
#Runs every 60th of a second
Clock.schedule_interval(game.update, 1.0/60.0)
return game
class MainScreen(Screen):
pass
class PingPongApp(App):
def build(self):
screen_manager = ScreenManager(transition=SlideTransition())
screen_manager.add_widget(MainScreen(name="main"))
screen_manager.add_widget(GameScreen(name="game"))
return screen_manager
if __name__ == "__main__":
PingPongApp().run()
乒乓.kv
#:kivy 1.11.1
<MainScreen>:
BoxLayout:
Button:
text: "Start!"
on_press: root.manager.current = "game"
<GameScreen>:
on_enter: self.play()
<PingPongBall>:
size: 75, 75
canvas:
Ellipse:
pos: self.pos
size: self.size
<PingPongPaddle>:
size: 50, 400
canvas:
Rectangle:
pos:self.pos
size:self.size
<PingPongGame>:
ball: PingPong_ball
player1: player_left
player2: player_right
canvas:
Rectangle:
pos: self.center_x - 5, 0
size: 20, self.height
Label:
font_size: 140
center_x: root.width / 4
top: root.top - 50
text: str(root.player1.score)
Label:
font_size: 140
center_x: root.width * 3 / 4
top: root.top - 50
text: str(root.player2.score)
Label:
font_size: 35
center_x: root.width * 6/7
top: root.top - 500
text: "by Me"
PingPongBall:
id: PingPong_ball
center: self.parent.center
PingPongPaddle:
id: player_left
x: root.x
center_y: root.center_y
PingPongPaddle:
id: player_right
x: root.width - self.width
center_y: root.center_y
提前致谢!
我是新来的,但我认为这可能会有所帮助 当我使用 Kivy 时,我也会导入“屏幕”并使用它。 这里是:
self.removeuser = RemoveUser()
screen = Screen(name="RemoveUser") # This will be the naem of screen to be called
screen.add_widget(self.removeuser)
self.screen_manager.add_widget(screen)
然后应该可以工作,返回 self.screen_manager 然后为您的开始按钮创建一个功能
PingPongApp.screen_manger.current = "<name of start screen>"
我通过在 GameScreen 的播放函数中包含这一行来修复它。
self.add_widget(game)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.