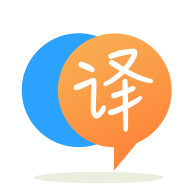
[英]How do fix the "missing 1 required positional argument: 'self'" error?
[英]How do I fix a required positional argument?
我正在研究员工管理系统,但遇到了立场论据的问题。 我对编码很陌生,这是一个课堂项目。 我遵循了一些教程和书籍,但我不断收到此错误:
Traceback (most recent call last):
File "C:\Users\jmcra\Desktop\CPT200\Employment Management System - Functionality 4.1.py", line 152, in <module>
searchEmployeeSSN()
TypeError: searchEmployeeSSN() missing 1 required positional argument: 'isForEdit'
我尝试了几种补救措施,但它只会导致更多错误。 有人可以帮我吗:
任何帮助是极大的赞赏!
#initialize list to store employee' information
employee_info = [ ]
#initialize global variable to save the SSN
employeeSSN = 0
#initialize global variable to save the index of the found record
employeeIndex = 0
#clear the screen
def cls():
print('\n' * 50)
#displaythe employee information in the required format
def employeeFormatedInfo(name, ssn, phone, email, salary):
print('')
print('--------------- {0:s} --------------------'.format(name))
print('SSN: {0:s}'.format(ssn))
print('Phone: {0:s}'.format(phone))
print('Email: {0:s}'.format(email))
print('Salary: ${0:s}'.format(salary))
print('------------------------------------------')
print('')
#view all employees in the system
def viewEmployeeInfo():
cls()
print('------------------------------------------')
print(' View all employees\n')
print('------------------------------------------\n')
if(len(employee_info)==0):
print('No employees entered.\n')
else:
for i in range(0, len(employee_info)):
line = employee_info[i].split(',')
employeeFormatedInfo(line[0], line[1], line[2], line[3], line[4])
input('Press any button to go to Main Menu')
cls()
#add employee
def addEmployee():
cls()
print('-------------------------------------------')
print(' Add Employee')
print('-------------------------------------------\n')
try:
#users can enter employee Name, SSN, Phone, Email and Salary
name=input('Employee Name: ')
ssn=input('Employee SSN: ')
phone=input('Employee phone number: ')
email=input('Employee email: ')
salary=input('Employee salary: $')
line = name +',' +ssn +',' +phone +',' +email +',' +salary
index = len(employee_info)
employee_info.insert(index, line)
except:
cls()
addEmployee()
print('\nEmployee info has been added!!\n')
print('--------------------------------------------\n')
try:
#users can add new employee or return to Main Menu
option=input('Enter q/Q to return to Main Menu or A to add another employee')
if option.lower() == 'q':
cls()
else:
cls()
addEmployee()
except:
cls()
addEmployee()
#display the Main Menu
def printOptions():
print('--------- Employee Management System -------\n')
print('There are ({0:2d} ) employees in the system.'.format(len(employee_info)))
print('--------------------------------------------\n')
print('1. View all employees\n')
print('2. Add new employee\n')
print('3. Search employee by SSN\n')
print('4. Edit employee information\n')
#check the user choice
try:
answer=int(input('Please enter your choice by number: '))
except ValueError:
print('Not a number')
return 100
print('--------------------------------------------\n')
return answer
def searchEmployeeSSN(isForEdit):
cls()
print('--------------------------------------------\n')
print(' Search for Employee by SSN\n')
print('--------------------------------------------\n')
if(len(employee_info)==0):
input('No employee in the list.\n')
cls()
else:
try:
ssn=input('Enter SSN to search for employee or q/Q to exit to Main : ')
global employeeSSN
employeeSSN = ssn
if ssn.lower() == 'q':
return 0
except ValueError:
searchEmployeeSSN(0)
for i in range(0, len(employee_info)):
line = employee_info[i].split(',')
if(line[1] == ssn):
global employeeIndex
employeeIndex = i
employeeFormatedInfo(line[0], line[1], line[2], line[3], line[4])
break
else:
print('\n No employees with that SSN.\n')
return 0
try:
if (isForEdit == 0):
option=input('Enter q/Q to exit or any key to add another employee:')
if option.lower() == 'q':
cls()
else:
searchEmployeeSSN(0)
except:
cls()
def editEmployeeInfo():
cls()
#reuse the searchEmployeeSSN
result = searchEmployeeSSN(1)
if(result != 0):
name=input('Employee new Name: ')
phone=input('Employee new phone number: ')
email=input('Employee new email: ')
salary=input('Employee new salary: $')
#delete the old information
del employee_info[employeeIndex]
line = name +',' +employeeSSN +',' +phone +',' +email +',' +salary
#add the new information
employee_info.insert(employeeIndex, line)
input('\nEmployee information has been updated. Please press any key to go to the Main Menu.\n')
while True:
cls()
mode = printOptions()
if mode == 1:
cls()
viewEmployeeInfo()
if mode == 2:
cls()
addEmployee()
if mode == 3:
cls()
searchEmployeeSSN()
if mode == 4:
cls()
editEmployeeInfo()
让我们分解您遇到的错误。
类型错误:searchEmployeeSSN() 缺少 1 个必需的位置参数:'isForEdit'
这是一个与函数searchEmployeeSSN
相关的错误。 参数是调用函数时传递给函数的值,因此我们需要查看调用函数的位置。
在 Python 中,您可以通过编写函数名称后跟一些括号来调用函数,例如: searchEmployeeSSN()
。 那么这有什么问题呢? 参数位于括号内,错误消息告诉您代码“ searchEmployeeSSN()
”缺少 1 个参数。
您像这样定义了函数:
def searchEmployeeSSN(isForEdit):
...
在这里, searchEmployeeSSN
有一个名为isForEdit
参数。 当您使用参数searchEmployeeSSN(5)
调用函数时,参数isForEdit
被分配参数的值。
您还可以为参数指定默认值,如下所示:
def searchEmployeeSSN(isForEdit=10):
...
没有给定默认值的参数称为位置参数,因为它们仅根据传递给函数的参数的位置分配值; 在您的情况下, isForEdit
是第一个参数,因此被分配了第一个参数的值。 位置参数是必需的,否则 Python 将不知道为它们分配什么值。
给定默认值的参数称为关键字参数,因为它们也可以使用参数名称赋值:
def f(x, m=5, c=0):
return m * x + c
f(10) # 50
f(10, 6) # 60
f(10, m=6) # 60
f(10, c=5) # 55
我希望你已经看到你需要做什么。 在底部的while True
块中,您调用searchEmployeeSSN()
而不传递任何参数。 您必须在此处传递参数或更改函数以使其没有位置参数。
我还建议对表示是/否、真/假类型值的变量使用布尔值。 在 Python 中,您可以使用值True
和False
。
def searchEmployeeSSN(isForEdit):
searchEmployeeSSN()
这两行是不一致的。 您正在定义需要参数的函数,但在没有参数的情况下调用它。
根据您的情况,有几种方法可以解决此问题。
1
和0
而不是True
和False
,您可能希望修复一些问题。def searchEmployeeSSN(isForEdit = False):
。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.