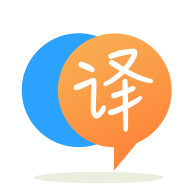
[英]Segmentation fault (core dumped) in dynamic memory allocation using a pointer(member) from struct
[英]Segmentation fault (core dumped) error in dynamic memory allocation
我是编码新手,也是 C++ 新手。 我正在尝试编写一个输入矩阵元素的代码。 之后,应删除一列,并添加一行。 删除一列工作正常。 但是,在为新的行数重新分配 memory 后,我收到消息:分段错误(核心转储)。 我正在使用指针来创建和编辑我的矩阵。 这是我的代码。 谢谢你的帮助!
#include <stdio.h>
#include <stdlib.h>
int main()
{
int c,r,**p,column,row;
printf("Enter the number of rows and columns:\n");
scanf("%d\n%d",&r,&c);
p=(int**)calloc(r,sizeof(int*));
if(p==NULL) printf("Memory not allocated.\n");
else{
for(int i=0;i<r;i++){
*(p+i)=(int*)calloc(c,sizeof(int));
printf("Enter %d. row\n",i+1);
for(int j=0;j<c;j++){
scanf("%d",*(p+i)+j);
}
}
printf("Original matrix:\n");
for(int i=0;i<r;i++){
for(int j=0;j<c;j++){
printf("%d ",*(*(p+i)+j));
}
printf("\n");
}
printf("Which column do you want to remove?");
scanf("%d",&column);
while(column<1||column>c){
printf("Wrong entry, enter again:");
scanf("%d",&column);
}
for(int i=0;i<=r-1;i++){
for(int j=column-1;j<=c-2;j++)
*(*(p+i)+j)=*(*(p+i)+j+1);
*(p+i)=(int*)realloc(*(p+i),(c-1)*sizeof(int));
}
printf("Matrix without %d. column:\n",column);
for(int i=0;i<r;i++){
for(int j=0;j<c-1;j++)
printf("%d ",*(*(p+i)+j));
printf("\n");
}
printf("Which row do you want to replace?\n");
scanf("%d",&row);
while(row<1||row>r){
printf("Wrong entry, enter again:\n");
scanf("%d",&row);
}
p=(int**)realloc(p,(r+1)*sizeof(int*));
if(p==NULL)
printf("Memory not allocated.\n");
else{
printf("Enter %d. row",row);
for(int i=r+1;i>row-1;i++){
*(p+i)=*(p+i-1);
}
for(int j=0;j<c-2;j++)
scanf("%d",*(p+row-1)+j);
printf("New matrix:\n");
for(int i=0;i<=r;i++){
for(int j=0;j<c-2;j++)
printf("%d ",*(*(p+i)+j));
printf("\n");
}
}
}
return 0;
}
当您为p
重新分配 memory 时,您会向该数组添加一个新行(一个额外的指针)。 但是您永远不会为该新行分配 memory,最终您访问了这个未初始化的指针。
但这不是真正的问题。 在循环中,您要向上提升指针以为插入的行腾出空间,您的初始值和循环增量都是错误的。 使用int i=r+1
的初始值,第一次写入*(p+i)
将访问已分配空间(即p[0]
到p[r]
)之后的一个空间。 循环增量应该是减量, --i
。 完成此循环后,您可以为新行分配空间。
正如这里的其他人所说,使用 std::vector 绝对更容易做到这一点。
但是,我假设您将其作为学习练习,因此我绝对建议您完成此操作并了解您出错的地方。 你很接近,看起来你只是对你的索引感到困惑。 尝试使用更具描述性的变量名称并将内容拆分为函数,以使其更难迷失在自己的代码中。
我修改了你的代码,让它做我认为你想做的事情。 看看,让我知道这是否有帮助。
但是,是的,如果你将来做任何 C++ 工作,请避免做 C 风格的 malloc、alloc、free 等......并考虑使用标准库。
#include <stdio.h>
#include <stdlib.h>
int main()
{
int c, r, ** p, column, row;
printf("Enter the number of rows and columns:\n");
scanf_s("%d\n%d", &r, &c);
p = (int**)calloc(r, sizeof(int*));
if (p == NULL) printf("Memory not allocated.\n");
else {
for (int i = 0; i < r; i++) {
*(p + i) = (int*)calloc(c, sizeof(int));
printf("Enter %d. row\n", i + 1);
for (int j = 0; j < c; j++) {
scanf_s("%d", *(p + i) + j);
}
}
printf("Original matrix:\n");
for (int i = 0; i < r; i++) {
for (int j = 0; j < c; j++) {
printf("%d ", *(*(p + i) + j));
}
printf("\n");
}
printf("Which column do you want to remove?");
scanf_s("%d", &column);
while (column<1 || column>c) {
printf("Wrong entry, enter again:");
scanf_s("%d", &column);
}
for (int i = 0; i <= r - 1; i++) {
for (int j = column - 1; j <= c - 2; j++)
* (*(p + i) + j) = *(*(p + i) + j + 1);
*(p + i) = (int*)realloc(*(p + i), (c - 1) * sizeof(int));
}
c -= 1;
printf("Matrix without %d. column:\n", column);
for (int i = 0; i < r; i++) {
for (int j = 0; j < c; j++)
printf("%d ", *(*(p + i) + j));
printf("\n");
}
printf("Which row do you want to replace?\n");
scanf_s("%d", &row);
while (row<1 || row>r) {
printf("Wrong entry, enter again:\n");
scanf_s("%d", &row);
}
p = (int**)realloc(p, (r + 1) * sizeof(int*));
if (p == NULL)
printf("Memory not allocated.\n");
else {
printf("Enter %d. row", row);
for (int i = 0; i < c; i++)
scanf_s("%d", *(p + row -1) + i);
printf("New matrix:\n");
for (int i = 0; i < r; i++) {
for (int j = 0; j < c; j++)
printf("%d ", *(*(p + i) + j));
printf("\n");
}
}
}
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.