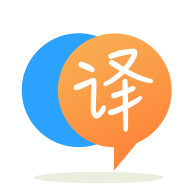
[英]Does Dijkstra algorithm keep track of visited nodes to find the shortest path?
[英]graph to find shortest path to origin using nodes I visited
给定方向和路线,我想知道最后只使用我以前去过的地方回到我开始的地方(原点)的最短路径
(下图示例,N 为北,S 为南等)
示例输入:NNEEEENWNENNSEEEWWWSWSESWWWNNNNWWWSSNNNNEE
样品 Output:14
我在 java 工作,想使用广度优先搜索算法并想使用图表,这是我可以构建这个图表并查看这个问题的最佳方式?
有人可以帮忙吗?
您可以通过读取输入生成已访问单元格的图表,然后从最后一个 position 在此图表上运行 BFS。
要生成图表,您可以执行以下操作:
for each direction :
create node for the cell if not already existing
for each adjacent cell, if visited then connect them together
只需运行一个 BFS,从末端开始并到达您已经通过的位置,一旦到达原点 position 就停止:
#include <bits/stdc++.h>
using namespace std;
int m[9][10];
int v[9][10];
struct point{
int x, y, dist;
point(){}
point(int x, int y){this->x = x; this->y = y; dist = 0;}
point(int x, int y, int dist){this->x = x; this->y = y; this->dist = dist;}
bool operator == (const point &rhs) const { return rhs.x == x && rhs.y == y; }
};
point origin(2, 8);
point target(2, 0);
int BFS(){
queue<point> q;
q.push(target);
while(!q.empty()){
point p = q.front();
q.pop();
v[p.y][p.x] = 1;
if(p == origin) return p.dist;
if(p.x > 0 && !v[p.y][p.x - 1] && m[p.y][p.x - 1]) q.push(point(p.x - 1, p.y, p.dist + 1)); //left
if(p.x < 9 && !v[p.y][p.x + 1] && m[p.y][p.x + 1]) q.push(point(p.x + 1, p.y, p.dist + 1)); //right
if(p.y > 0 && !v[p.y - 1][p.x] && m[p.y - 1][p.x]) q.push(point(p.x, p.y - 1, p.dist + 1)); //up
if(p.y < 8 && !v[p.y + 1][p.x] && m[p.y + 1][p.x]) q.push(point(p.x, p.y + 1, p.dist + 1)); //down
}
return -1;
}
int main(){
memset(m, 0, sizeof(m));
memset(v, 0, sizeof(v));
m[origin.y][origin.x] = 1;
string path = "NNEEEENWNENNSEEEWWWSWSESWWWNNNNWWWSSNNNNEE";
point o(origin.x, origin.y);
for(int i = 0; i < path.size(); i++){
if(path[i] == 'N') o.y--;
else if(path[i] == 'S') o.y++;
else if(path[i] == 'W') o.x--;
else if(path[i] == 'E') o.x++;
m[o.y][o.x] = 1;
}
cout<<"dist = "<<BFS()<<endl;
}
OUTPUT:距离 = 14 。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.