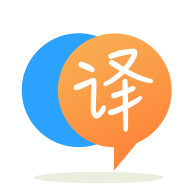
[英]From array of objects extract array of property values for items having another property equal to given value
[英]In array of objects sum up certain property values if another property has given value
如果帐户类型是“储蓄”,我正在尝试获取帐户余额的总和。 我的想法是使用 forEach 循环遍历数组,然后如果帐户类型是储蓄,但不确定为什么它不起作用。
const accounts = [
{acctNo: 123, type: 'Checking', balance: 150},
{acctNo: 234, type: 'Checking', balance: 200},
{acctNo: 345, type: 'Savings', balance: 550},
{acctNo: 456, type: 'Checking', balance: 550},
{acctNo: 567, type: 'Savings', balance: 1500}
];
const sum = accounts.forEach(function(account){
if (account.type=== 'Savings'){
account.reduce(function(a,b){
return {balance: a.balance + b.balance}
})
}
})
console.log(sum)
你不能在 forEach 中返回任何东西,直接减少帐户是没有意义的。 尝试使用过滤器和 map 代替,并确保您确实减少了列表:
accounts.filter(a => a.type === 'Savings')
.map(a => a.balance)
.reduce((a, b) => a + b)
通过链接一些简单的数组方法,您可以做到这一点:
1)使用filter
只获取类型为'Savings'
的账户
2) 使用map
仅获取余额
3)然后对数组求和,我已经使用reduce
完成了它,它很好地与map
和filter
并列,但是如果您愿意,您可以使用显式循环并手动跟踪总和来完成
请注意,我使用了箭头函数,只是因为它更简洁一些并且更清楚(imo)函数正在做什么 - 但每个都可以用旧式function() {... }
表达式替换仍然可以正常工作。
const accounts = [ {acctNo: 123, type: 'Checking', balance: 150}, {acctNo: 234, type: 'Checking', balance: 200}, {acctNo: 345, type: 'Savings', balance: 550}, {acctNo: 456, type: 'Checking', balance: 550}, {acctNo: 567, type: 'Savings', balance: 1500} ]; const sum = accounts.filter(account => account.type === 'Savings').map(account => account.balance).reduce((acc, bal) => acc + bal); console.log(sum)
reduce
是一种数组方法,而不是 object 方法。
您不需要同时使用forEach
和reduce
。 reduce
已经迭代了数组,您只需要将if
条件放入 function 中。
const accounts = [ {acctNo: 123, type: 'Checking', balance: 150}, {acctNo: 234, type: 'Checking', balance: 200}, {acctNo: 345, type: 'Savings', balance: 550}, {acctNo: 456, type: 'Checking', balance: 550}, {acctNo: 567, type: 'Savings', balance: 1500} ]; const sum = accounts.reduce(function(a, account){ if (account.type === 'Savings'){ return a + account.balance; } else { return a; } }, 0) console.log(sum)
关注@RobinZigmond 的评论
filter
=> 从数组中删除所有不属于'Savings'类型的帐户,并将type === 'savings'
的帐户作为数组返回。 map
方法接收这个数组
map
=> 返回一个仅包含储蓄账户余额的数组。 reduce
接收它
reduce
=> 基本上将数组中的所有内容都简化为一件事。 在这种情况下,它接收余额数组并将它们全部相加并将其返回给sum
const accounts = [
{ acctNo: 123, type: 'Checking', balance: 150 },
{ acctNo: 234, type: 'Checking', balance: 200 },
{ acctNo: 345, type: 'Savings', balance: 550 },
{ acctNo: 456, type: 'Checking', balance: 550 },
{ acctNo: 567, type: 'Savings', balance: 1500 }
];
const sum = accounts
.filter((account) => account.type.toLowerCase() === 'savings')
.map((savingsAccounts) => savingsAccounts.balance)
.reduce((accumulator, balance) => accumulator + balance);
console.log(sum);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.