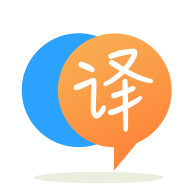
[英]Ubuntu 16.04 LTS and Oracle Instant Client - C++ linking error
[英]Compiling Problem with c++ on Ubuntu 16.04 LTS?
所以我有一个调试器问题。 所以我创建了一个 c++ 程序并在 Ubuntu 16.04 上运行它。 但是,当尝试通过在命令行中键入g++ decrypt.cpp caesor\ cipher.cpp -o decrypt进行编译时,它给了我一些错误。 这是我很长时间以来第一次在 c++ 上工作,希望你们能发现一些我看不到的东西。 这是下面的代码和错误。 谢谢你
======================================
解密.cpp
#include"decrypt.h"
#include<fstream>
#include<string>
using namespace std;
#include<iostream>
Decrypt::Decrypt(string text)
{
str = text;
zero_analysis_array();
//E A R I O are top 5 most commonly used letters in english words
//initializing keys arrays with thier position in alphabets
keys[0] = 'E' - 'A';
keys[1] = 'A' - 'A';
keys[2] = 'R' - 'A';
keys[3] = 'I' - 'A';
keys[4] = 'O' - 'A';
}
void Decrypt::zero_analysis_array()
{
for (int i = 0; i < 26; i++)
{
freq[i] = 0;
}
}
void Decrypt::character_count()
{
char ch;
for (int i = 0; i < str.size(); i++)
{
if (is_alpha(str[i]))
{
ch = str[i];
if (!is_upper(ch))
ch = to_upper(str[i]);
int a = ch - 'A';
if (a > 26 || a < 0)
cout << "a";
freq[ch - 'A']++;
}
}
}
void Decrypt::print_analysis_array()
{
char alpha = 'A';
cout << "\n\n";
for (int i = 0; i < 26; i++)
{
cout << char(alpha + i) << " occurs " << freq[i] << " times\n";
}
int ind = max_index(), key;
cout << "Possible keys:\n";
for (int i = 0; i < 5; i++)
{
key = ind - keys[i];
if (key < 0)
key = key + 26;
cout << key << "\n";
}
}
string Decrypt::decrypt(int key)
{
string decrypt = str;
int code, a, b;
char ch;
for (int i = 0; i < decrypt.size(); i++)
{
if (is_alpha(decrypt[i]))
{
ch = 'A';
if (is_upper(decrypt[i]) == false)
ch = 'a';
a = (decrypt[i] - ch);
b = a + 26 - key;
code = b % 26;
decrypt[i] = ch + code;
}
}
return decrypt;
}
void Decrypt::write_to_file(string fname, string text)
{
fstream file;
file.open(fname, ios::out);
file << text;
file.close();
}
void Decrypt::decryption(string fname)
{
character_count();
print_analysis_array();
string decrypted;
int key;
char choice;
do
{
cout << "Select/Enter key(0-26):";
cin >> key;
while (key < 0 || key>26)
{
cout << "Invalid Key!! Must be between 0-26\nEnter Again:";
cin >> key;
}
decrypted = decrypt(key);
cout << "\nHere is decrypted text:\n\n" << decrypted;
cout << "\n\nDo you want to try again with different key? (y/n):";
cin >> choice;
while (choice != 'Y' && choice != 'y' && choice != 'n' && choice != 'N')
{
cout << "Invalid choice!!.\nEnter (y/n):";
cin >> choice;
}
} while (choice == 'y' || choice == 'Y');
write_to_file(fname, decrypted);
cout << "Decrypted text written to file " << fname << "\n";
}
int Decrypt::max_index()
{
int maxIndex = 0;
int maxFreq = freq[0];
for (int i = 0; i < 26; i++)
{
if (maxFreq < freq[i])
{
maxIndex = i;
maxFreq = freq[i];
}
}
return maxIndex;
}
char Decrypt::to_upper(char ch)
{
if (!is_upper(ch))
return ch - 32;
return ch;
}
bool Decrypt::is_alpha(char ch)
{
return((ch >= 'A' && ch <= 'Z') || (ch >= 'a' && ch <= 'z'));
}
bool Decrypt::is_upper(char ch)
{
return (ch >= 'A' && ch <= 'Z');
}
==================================
凯撒密码.cpp
#include<fstream>
#include <iostream>
#include"decrypt.h"
int main(int argc,char*argv[])
{
if (argc < 2)
{
cout << "Invalid no of Arguments!!!\n";
exit(0);
}
string text=read_from_file(argv[1]);
cout << text;
Decrypt d(text);
d.decryption(argv[2]);
}
string read_from_file(char* fname)
{
fstream file;
string line,text="";
file.open(fname, ios::in);
while (getline(file, line))
{
text = text + line;
}
return text;
}
==========================
decrypt.cpp: In member function ‘void Decrypt::write_to_file(std::__cxx11::string, std::__cxx11::string)’:
decrypt.cpp:88:30: error: no matching function for call to ‘std::basic_fstream<char>::open(std::__cxx11::string&, const openmode&)’
file.open(fname, ios::out);
^
In file included from decrypt.cpp:2:0:
/usr/include/c++/5/fstream:1001:7: note: candidate: void std::basic_fstream<_CharT, _Traits>::open(const char*, std::ios_base::openmode) [with _CharT = char; _Traits = std::char_traits<char>; std::ios_base::openmode = std::_Ios_Openmode]
open(const char* __s,
^
/usr/include/c++/5/fstream:1001:7: note: no known conversion for argument 1 from ‘std::__cxx11::string {aka std::__cxx11::basic_string<char>}’ to ‘const char*’
caesor cipher.cpp: In function ‘int main(int, char**)’:
caesor cipher.cpp:9:15: error: ‘exit’ was not declared in this scope
exit(0);
好吧,编译器实际上会告诉您程序有什么问题(并且还会提示错误所在的位置)。
decrypt.cpp:88:30: error: no matching function for call to ‘std::basic_fstream<char>::open(std::__cxx11::string&, const openmode&)’
file.open(fname, ios::out);
^
这意味着您对std::fstream::open
的调用不正确。 它需要一个 C 字符串,而不是你给它的 C++ std::string
。 这里最简单的解决方法是将调用更改为open
,如下所示:
file.open(fname.c_str(), ios::out);
第二个错误说它找不到名为exit
的 function 。 这是因为编译器还不知道这个 function。 您需要在声明 function 的地方包含适当的 header (在本例中为stdlib.h
),以便编译器知道存在一个名为exit
的 ZC1C425268E68385D1AB5074C17A94F1。
你的错误是不言自明的。 它说:
error: no matching function for call to ‘std::basic_fstream<char>::open(std::__cxx11::string&, const openmode&)’
但它让您知道最接近的匹配是:
note: candidate: void std::basic_fstream<_CharT, _Traits>::open(const char*, std::ios_base::openmode)
如果您注意到,最大的区别在于string
与char *
。 所以给它它正在寻找的 C 字符串:
file.open(fname.c_str(), ios::in);
但是,从 C++11 开始, open()
应该接受std::string
。 因此,假设您使用的是g++
,您也可以添加
-std=c++11
编译时。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.