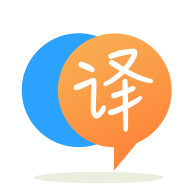
[英]When should I use static memory allocation and when should I use dynamic memory allocation?
[英]when should i use Dynamic memory allocation?
我正在学习 C 中的malloc
、 calloc
、 free
和realloc
,但我不知道什么时候应该使用这些功能。
例如:如果我想在 C 中创建一个创建数组的程序,并且它的大小由用户输入确定:
int n;
printf("Enter THe Number of element in Array:..\n");
scanf("%d",&n);
int x[n];
for(int i = 0 ; i < n ; i++)
{
x[i] = i+ 1;
}
for(int y = 0 ; y < n ; y++)
{
printf("%d\n",x[y]);
}
output:
Enter THe Number of element in Array:..
5
1
2
3
4
5
所以在这里我创建了这个程序,没有使用动态 memory 分配,这让我的事情变得更加复杂。
堆通常比用于容纳自动变量的区域和 static 存储区域(全局变量)大得多。
因此,您可以在以下情况下使用动态分配:
但在你的例子中
int x[n];
不是动态分配。 您只需分配具有大小设置运行时的自动数组。 你不能改变它的大小,当你退出 scope 时你也不能使用它,因为它的生命周期绑定到它定义的 scope。
您的示例使用了一种称为variable-length array的东西,它在某些情况下可以替代动态 memory 。 但是,并非所有版本的 C 都支持 VLA(它们在 1999 年修订版中首次引入,并在 2011 年修订版中成为可选),它们不能是struct
或union
类型的成员,也不能在文件中用作“全局变量” scope。 与固定大小的 arrays 一样,一旦您退出其封闭的 scope(即块或函数),它们的存储就会被释放。 它们通常不能任意大。
在以下情况下,您将使用动态 memory ( malloc
、 calloc
或realloc
):
一个(实际上是非常常见的)情况是,使用在本地分配的 memory(通常但不一定在堆栈上),如您的示例所做的那样,如果 memory 是在 ZC1C425268E68385D1AB5074FC1 内部分配的,则该 memory 将不起作用当 function 返回时,memory 将被重置或“丢失”。
在这种情况下,您需要使用malloc
(或相关)调用动态分配 memory(在堆上)。 这样分配的 Memory 将是“全局的”,并且可以被程序的任何其他部分访问(只要它具有指针),直到通过调用free()
function显式释放它。
这是一个例子:
#include <stdio.h>
#include <stdlib.h>
int* newArray(int n)
{
// int x[n]; // Won't work: This is local (stack) memory that is lost on return
int* x = malloc(n * sizeof(int)); // Works: This is global (heap) memory
return x;
}
int main()
{
int n;
printf("Enter The Number of Elements in Array: ");
scanf("%d", &n);
int* x = newArray(n);
for (int i = 0; i < n; i++) {
x[i] = i + 1;
}
for (int y = 0; y < n; y++) {
printf("%d\n", x[y]);
}
free(x); // You MUST free memory that you've allocated with malloc
return 0;
}
(您可以尝试取消注释“不会工作”行并注释掉“工作”行,自己看看。)
您可以使用动态 memory 分配在运行时分配变量,并且您应该遵循一些步骤,您需要使用此分配 memory
int* x = malloc(n * sizeof(int));
并在使用后释放它
free(x);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.