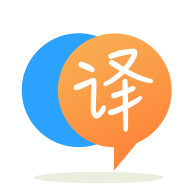
[英]Swift: how to finish a UIView draw animation before changing a label
[英]How to Apply Animation while changing UIView size? + Swift 5
Im new to IOS development and I have two buttons in the UIView and when user select the option portrait
or landscape
, change the UIView re-size and change the background color as well and i need to add animation for that process. 例如:用户 select portrait
,然后用户可以看到红色 UIVIew。 单击landscape
选项后,animation 应该启动,看起来,红色图像出现在前面并更改landscape
模式的大小(更改高度和宽度)和 go 到以前的 Z4757FE07FD492A8BE0EA6A760D683D6E 并更改为绿色。 我在代码上添加了小 UIView animation,这有助于您确定我们应该从哪里开始 animation 并完成它。 如果有人知道如何正确操作,请告诉我并感谢您的帮助。 请参考下面的代码
import UIKit
class ViewController: UIViewController {
let portraitWidth : CGFloat = 400
let portraitHeight : CGFloat = 500
let landscapeWidth : CGFloat = 700
let landscapeHeight : CGFloat = 400
var mainView: UIView!
var mainStackView: UIStackView!
let segment: UISegmentedControl = {
let segementControl = UISegmentedControl()
return segementControl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.translatesAutoresizingMaskIntoConstraints = false
mainStackView = UIStackView()
mainStackView.axis = .vertical
mainStackView.translatesAutoresizingMaskIntoConstraints = false
mainStackView.alignment = .center
mainStackView.distribution = .equalCentering
self.view.addSubview(mainStackView)
self.segment.insertSegment(withTitle: "Portrait", at: 0, animated: false)
self.segment.insertSegment(withTitle: "Landscape", at: 1, animated: false)
self.segment.selectedSegmentIndex = 0
self.segment.addTarget(self, action: #selector(changeOrientation(_:)), for: .valueChanged)
self.segment.translatesAutoresizingMaskIntoConstraints = false
self.segment.selectedSegmentIndex = 0
mainStackView.addArrangedSubview(self.segment)
let safeAreaLayoutGuide = self.view.safeAreaLayoutGuide
NSLayoutConstraint.activate([
self.mainStackView.topAnchor.constraint(equalTo: safeAreaLayoutGuide.topAnchor, constant: 20),
self.mainStackView.centerXAnchor.constraint(equalTo: safeAreaLayoutGuide.centerXAnchor, constant: 0),
])
NSLayoutConstraint.activate([
self.segment.heightAnchor.constraint(equalToConstant: 35),
self.segment.widthAnchor.constraint(equalToConstant: 300)
])
mainView = UIView(frame: .zero)
mainView.translatesAutoresizingMaskIntoConstraints = false
mainStackView.addArrangedSubview(mainView)
mainView.backgroundColor = UIColor.red
NSLayoutConstraint.activate([
mainView.heightAnchor.constraint(equalToConstant: portraitHeight),
mainView.widthAnchor.constraint(equalToConstant: portraitWidth),
mainView.topAnchor.constraint(equalTo: segment.bottomAnchor, constant: 30)
])
}
@IBAction func changeOrientation(_ sender: UISegmentedControl) {
self.mainView.constraints.forEach{ (constraint) in
self.mainView.removeConstraint(constraint)
}
UIView.animate(withDuration: 1.0) {
if (sender.selectedSegmentIndex == 0) {
self.mainView.backgroundColor = UIColor.red
NSLayoutConstraint.activate([
self.mainView.heightAnchor.constraint(equalToConstant: self.portraitHeight),
self.mainView.widthAnchor.constraint(equalToConstant: self.portraitWidth),
self.mainView.topAnchor.constraint(equalTo: self.segment.bottomAnchor, constant: 30)
])
} else {
self.mainView.backgroundColor = UIColor.green
NSLayoutConstraint.activate([
self.mainView.heightAnchor.constraint(equalToConstant: self.landscapeHeight),
self.mainView.widthAnchor.constraint(equalToConstant: self.landscapeWidth),
self.mainView.topAnchor.constraint(equalTo: self.segment.bottomAnchor, constant: 30)
])
}
}
}
}
更新逻辑
@IBAction func changeOrientation(_ sender: UISegmentedControl) {
UIView.animate(withDuration: 4.0) {
if (sender.selectedSegmentIndex == 0) {
self.mainView.backgroundColor = UIColor.red
self.widthConstraint.constant = self.portraitWidth
self.heightConstraint.constant = self.portraitWidth
} else {
self.mainView.backgroundColor = UIColor.green
self.widthConstraint.constant = self.landscapeWidth
self.heightConstraint.constant = self.landscapeHeight
}
self.mainView.layoutIfNeeded()
} }
有可能,基本问题是:
• 不可能对一个约束和另一个约束之间的实际变化进行动画处理。
要更改大小或形状,您只需对约束的长度进行动画处理。
在您学习时,我真的会敦促您简单地为约束设置动画。
所以,不要试图“改变”约束,简单地动画一个或另一个的长度。
yourConstraint.constant = 99 // it's that easy
我也真的敦促你把它放在 storyboard 上。
你必须先掌握它!
堆栈视图也没有任何理由,现在摆脱它。
只要有一个出口
@IBOutlet var yourConstraint: NSLayoutConstraint!
和动画
UIView.animateWithDuration(4.0) {
self.yourConstraint.constant = 666
self.view.layoutIfNeeded()
}
请务必在 Stack Overflow 中搜索“iOS 动画约束”,因为有很多东西需要学习。
在 storyboard 上执行此操作,这样一个简单的事情最多需要 20 秒。 一旦你掌握了 storyboard,只有在有理由的情况下才继续编写代码
忘记堆栈视图
只需对约束的常量进行动画处理,即可更改大小、形状或任何您喜欢的内容。
请务必在 SO 和其他网站上搜索许多精彩的文章“iOS 中的动画约束”!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.