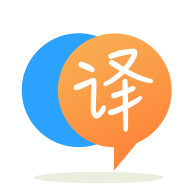
[英]How can I return two fields using Linq on an object with more than two fields?
[英]How can i return several fields directly in a WCF Response object, rather than wrapped in another object?
如何直接从 WCF 服务返回响应,其中字段直接位于 Response 对象中,而不是包装在 Response 对象中的 Result 对象中?
我继承并维护了运行良好的 WCF 服务。 我被要求添加一个 passthru 方法来做某事,然后将请求路由到另一个现有服务(由其他人维护),然后按原样返回结果。 我试图解决的部分是现有服务在其响应对象中返回松散字段,但 WCF 似乎希望我返回单个值(或包含在对象中的值)。
我怎么可能:
例如,我想返回这个(即从其他服务返回的内容)
<soap:Body>
<MethodResponse>
<Value1>123</Value1>
<Value2>abc</Value2>
<Value3>http://123.com</Value3>
<Value4>Success</Value4>
</MethodResponse>
</soap:Body>
而不是这个
<soap:Body>
<MethodResponse>
<MethodResult>
<Value1>123</Value1>
<Value2>abc</Value2>
<Value3>http://123.com</Value3>
<Value4>Success</Value4>
</MethodResult>
</MethodResponse>
</soap:Body>
如果在服务操作签名中未使用 MessageContractAttribute,WCF 将创建一个包装器元素以将参数保存在 SOAP 正文中。 这是默认的 WCF 行为。
解决方案是在对象上使用 [MessageContract]。
这是我的演示:
[MessageContract]
public class MethodResponse
{
[MessageBodyMember]
public string value1 { get; set; }
[MessageBodyMember]
public string value2 { get; set; }
[MessageBodyMember]
public string value3 { get; set; }
}
上面的类是服务器返回的类。
这是客户端收到的消息。
关于 MessageContract 的更多信息,请参考以下链接:
https://docs.microsoft.com/en-us/dotnet/framework/wcf/feature-details/using-message-contracts
如果问题仍然存在,请随时告诉我。
这是我的代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Description;
using System.Text;
using System.Threading.Tasks;
using static ConsoleApp65.soap;
namespace ConsoleApp65
{
[MessageContract]
public class MethodResponse
{
[MessageBodyMember]
public string value1 { get; set; }
[MessageBodyMember]
public string value2 { get; set; }
[MessageBodyMember]
public string value3 { get; set; }
}
[ServiceContract]
public interface Test
{
[OperationContract]
MethodResponse Test();
}
public class TestService : Test
{
public MethodResponse Test()
{
MethodResponse data = new MethodResponse();
data.value1 = "1";
data.value2 = "2";
data.value3 = "3";
return data;
}
}
class Program
{
static void Main(string[] args)
{
// Step 1: Create a URI to serve as the base address.
Uri baseAddress = new Uri("http://localhost:8000/GettingStarted/");
// Step 2: Create a ServiceHost instance.
ServiceHost selfHost = new ServiceHost(typeof(TestService), baseAddress);
try
{
// Step 3: Add a service endpoint.
selfHost.AddServiceEndpoint(typeof(Test), new WSHttpBinding(), "CalculatorService");
// Step 4: Enable metadata exchange.
ServiceMetadataBehavior smb = new ServiceMetadataBehavior();
smb.HttpGetEnabled = true;
selfHost.Description.Behaviors.Add(smb);
// Step 5: Start the service.
selfHost.Open();
Console.WriteLine("The service is ready.");
// Close the ServiceHost to stop the service.
Console.WriteLine("Press <Enter> to terminate the service.");
Console.WriteLine();
Console.ReadLine();
selfHost.Close();
}
catch (CommunicationException ce)
{
Console.WriteLine("An exception occurred: {0}", ce.Message);
selfHost.Abort();
}
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.