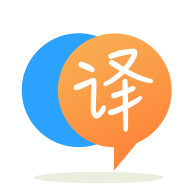
[英].collect(Collectors.toList()) and Streams on Java Method
[英]Java 8 streams: Shorthand for myList.stream().map(Foo::bar).collect(Collectors.toList())
以下是否有通用的简写? 欢迎像番石榴这样的外部依赖项。
myList.stream().map(Foo::bar).collect(Collectors.toList());
如果我必须实现它,它会是这样的:
static <T, U> List<U> mapApply(List<T> list, Function<T, U> function) {
return list.stream().map(function).collect(Collectors.toList());
}
有没有适用于任何 Iterable 的? 如果没有,我该怎么写? 我开始这样思考:
static <T, U, V extends Iterable> V<U> mapApply(V<T> iterable, Function<T, U> function) {
return iterable.stream().map(function).collect(???);
}
在Foo::bar
再次返回Foo
实例的情况下,即。 您需要再次将T
转换为T
,然后您可以使用使用UnaryOperator<T>
List::replaceAll
,因此每个项目都被相同类型的一个替换。 此解决方案改变了原始列表。
List<String> list = Arrays.asList("John", "Mark", "Pepe");
list.replaceAll(s -> "hello " + s);
如果您想将T
转换为R
,您所能做的就是将当前的解决方案与一系列stream()
-> map()
-> collect()
方法调用或简单迭代一起使用。
包装 this 的静态方法也可以这样做。 请注意,您不能使用相同的方式从Collection
和Iterable
创建Stream
。 也可以随意传递您的自定义Collector
。
T
是输入Collection
或Iterable
的泛型类型。R
是映射函数结果的泛型类型(从T
到R
映射) 来自Collection<T>
List<Bar> listBarFromCollection = mapApply(collectionFoo, Foo::bar, Collectors.toList());
static <T, R> List<R> mapApply(Collection<T> collection, Function<T, R> function) {
return collection.stream()
.map(function)
.collect(Collectors.toList());
}
来自Iterable<T>
List<Bar> listBarFromIterable = mapApply(iterableFoo, Foo::bar, Collectors.toList());
static <T, R> List<R> mapApply(Iterable<T> iterable, Function<T, R> function) {
return StreamSupport.stream(iterable.spliterator(), false)
.map(function)
.collect(Collectors.toList());
}
...与Collector
:
如果要传递自定义Collector
,它将是Collector<R, ?, U> collector
和方法U
的返回类型而不是List<R>
。 正如@Holger 指出的那样,将Collector
传递给方法与调用实际的stream()
-> map()
-> collect()
没有太大区别。
你可以实现这样的方法:
public class StreamShorthandUtil {
public static <T, U, V extends Collection<T>, W> W mapApply(V in, Function<T, U> function, Collector<U, ?, W> collector) {
return in.stream().map(function).collect(collector);
}
// main class for testing
public static void main(String[] args) {
List<String> numbersAsString = Arrays.asList("1", "2", "3", "4", "5");
List<Integer> numbers = mapApply(numbersAsString, Integer::parseInt, Collectors.toList());
}
}
除了输入和映射器函数之外,此方法还有一个Collector
参数,用于定义返回的类型。
您可以使用Eclipse Collections ,它直接在集合上使用具有丰富 API 的集合,并为collect
(又名map
)等方法提供协变返回类型。
例如:
MutableList<Foo> fooList;
MutableList<Bar> barList = fooList.collect(Foo::bar);
MutableSet<Foo> fooSet;
MutableSet<Bar> barSet = fooSet.collect(Foo::bar);
MutableBag<Foo> fooBag;
MutableBag<Bar> barBag = fooBag.collect(Foo::bar);
Iterate
实用程序类也适用于任何Iterable
并提供丰富的协议集。
Iterable<Foo> fooIterable;
List<Bar> barList = Iterate.collect(fooIterable, Foo::bar, new ArrayList<>());
Set<Bar> barSet = Iterate.collect(fooIterable, Foo::bar, new HashSet<>());
注意:我是 Eclipse Collections 的提交者。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.