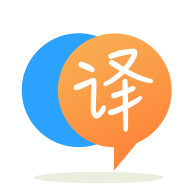
[英].collect(Collectors.toList()) and Streams on Java Method
[英]Java 8 streams: Shorthand for myList.stream().map(Foo::bar).collect(Collectors.toList())
以下是否有通用的簡寫? 歡迎像番石榴這樣的外部依賴項。
myList.stream().map(Foo::bar).collect(Collectors.toList());
如果我必須實現它,它會是這樣的:
static <T, U> List<U> mapApply(List<T> list, Function<T, U> function) {
return list.stream().map(function).collect(Collectors.toList());
}
有沒有適用於任何 Iterable 的? 如果沒有,我該怎么寫? 我開始這樣思考:
static <T, U, V extends Iterable> V<U> mapApply(V<T> iterable, Function<T, U> function) {
return iterable.stream().map(function).collect(???);
}
在Foo::bar
再次返回Foo
實例的情況下,即。 您需要再次將T
轉換為T
,然后您可以使用使用UnaryOperator<T>
List::replaceAll
,因此每個項目都被相同類型的一個替換。 此解決方案改變了原始列表。
List<String> list = Arrays.asList("John", "Mark", "Pepe");
list.replaceAll(s -> "hello " + s);
如果您想將T
轉換為R
,您所能做的就是將當前的解決方案與一系列stream()
-> map()
-> collect()
方法調用或簡單迭代一起使用。
包裝 this 的靜態方法也可以這樣做。 請注意,您不能使用相同的方式從Collection
和Iterable
創建Stream
。 也可以隨意傳遞您的自定義Collector
。
T
是輸入Collection
或Iterable
的泛型類型。R
是映射函數結果的泛型類型(從T
到R
映射) 來自Collection<T>
List<Bar> listBarFromCollection = mapApply(collectionFoo, Foo::bar, Collectors.toList());
static <T, R> List<R> mapApply(Collection<T> collection, Function<T, R> function) {
return collection.stream()
.map(function)
.collect(Collectors.toList());
}
來自Iterable<T>
List<Bar> listBarFromIterable = mapApply(iterableFoo, Foo::bar, Collectors.toList());
static <T, R> List<R> mapApply(Iterable<T> iterable, Function<T, R> function) {
return StreamSupport.stream(iterable.spliterator(), false)
.map(function)
.collect(Collectors.toList());
}
...與Collector
:
如果要傳遞自定義Collector
,它將是Collector<R, ?, U> collector
和方法U
的返回類型而不是List<R>
。 正如@Holger 指出的那樣,將Collector
傳遞給方法與調用實際的stream()
-> map()
-> collect()
沒有太大區別。
你可以實現這樣的方法:
public class StreamShorthandUtil {
public static <T, U, V extends Collection<T>, W> W mapApply(V in, Function<T, U> function, Collector<U, ?, W> collector) {
return in.stream().map(function).collect(collector);
}
// main class for testing
public static void main(String[] args) {
List<String> numbersAsString = Arrays.asList("1", "2", "3", "4", "5");
List<Integer> numbers = mapApply(numbersAsString, Integer::parseInt, Collectors.toList());
}
}
除了輸入和映射器函數之外,此方法還有一個Collector
參數,用於定義返回的類型。
您可以使用Eclipse Collections ,它直接在集合上使用具有豐富 API 的集合,並為collect
(又名map
)等方法提供協變返回類型。
例如:
MutableList<Foo> fooList;
MutableList<Bar> barList = fooList.collect(Foo::bar);
MutableSet<Foo> fooSet;
MutableSet<Bar> barSet = fooSet.collect(Foo::bar);
MutableBag<Foo> fooBag;
MutableBag<Bar> barBag = fooBag.collect(Foo::bar);
Iterate
實用程序類也適用於任何Iterable
並提供豐富的協議集。
Iterable<Foo> fooIterable;
List<Bar> barList = Iterate.collect(fooIterable, Foo::bar, new ArrayList<>());
Set<Bar> barSet = Iterate.collect(fooIterable, Foo::bar, new HashSet<>());
注意:我是 Eclipse Collections 的提交者。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.