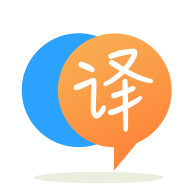
[英]Are There Any Hidden Costs to Passing Around a Struct With a Single Reference?
[英]Passing around a reference to a struct in Rust
我的问题基本上是在我的程序中,我需要将对s
结构的引用传递到多个地方,包括一个新线程。 例如,在 C 中,我可以将其声明为全局结构并以这种方式使用它。 如何在 rust 中做到这一点?
对于某些代码,我还需要使用包装在Rc
中的RefCell
(我之前的问题)。
fn a_thread(s: &SomeStruct) {
//... code using s reference ... //
}
struct SomeStruct {
val: bool,
}
fn main() {
let mut s = SomeStruct { val: true };
let s_rc = Rc::new(RefCell::new(s));
thread::spawn(move || a_thread(&s)); // <= error: use of moved value 's'
//... code using the s_rc ... //
}
如果一个线程修改数据而另一个线程读取它,则必须同步,否则您将遇到数据竞争。 安全的 Rust 通过 static 分析防止数据竞争,因此它不允许您获得&SomeStruct
,而基础值可以由另一个线程修改。
您可以做的是使用互斥体而不是RefCell
和Arc
而不是Rc
:
fn a_thread(s: Arc<Mutex<SomeStruct>) {
// when you need data from s:
{
let s = s.lock().unwrap();
// here you can read from s, or even obtain a `&SomeStruct`
// but as long as you hold on to it, the main thread will be
// blocked in its attempts to modify s
}
}
fn main() {
// create s on the heap
let s = Arc::new(Mutex::new(SomeStruct { val: true }));
// cloning the Arc creates another reference to the value
let s2 = Arc::clone(&s);
thread::spawn(move || a_thread(s2));
//... code using s ... //
{
let s = s.lock().unwrap();
// here you can modify s, but reading will be blocked
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.