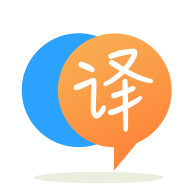
[英]How can i get images from the hard disk resize the images and add them to a list<image> fast?
[英]How can I download the images from the site to my hard disk?
我正在尝试下载图像,而不是仅提取每个图像的时间和日期。 该代码有效,但仅适用于时间和日期。
using System;
using System.Linq;
using System.IO;
using System.Xml;
using System.Net;
using HtmlAgilityPack;
public class Program
{
public static void Main()
{
var wc = new WebClient();
wc.BaseAddress = "https://something.com";
HtmlDocument doc = new HtmlDocument();
var temp = wc.DownloadData("/en");
doc.Load(new MemoryStream(temp));
var secTokenScript = doc.DocumentNode.Descendants()
.Where(e =>
String.Compare(e.Name, "script", true) == 0 &&
String.Compare(e.ParentNode.Name, "div", true) == 0 &&
e.InnerText.Length > 0 &&
e.InnerText.Trim().StartsWith("var region")
).FirstOrDefault().InnerText;
var securityToken = secTokenScript;
securityToken = securityToken.Substring(0, securityToken.IndexOf("arrayImageTimes.push"));
securityToken = secTokenScript.Substring(securityToken.Length).Replace("arrayImageTimes.push('", "").Replace("')", "");
var dates = securityToken.Trim().Split(new string[] { ";"}, StringSplitOptions.RemoveEmptyEntries);
var scriptDates = dates.Select(x => new ScriptDate { DateString = x });
foreach(var date in scriptDates)
{
Console.WriteLine("Date String: '" + date.DateString + "'\tYear: '" + date.Year + "'\t Month: '" + date.Month + "'\t Day: '" + date.Day + "'\t Hours: '" + date.Hours + "'\t Minutes: '" + date.Minutes + "'");
}
}
public class ScriptDate
{
public string DateString {get;set;}
public int Year
{
get
{
return Convert.ToInt32(this.DateString.Substring(0, 4));
}
}
public int Month
{
get
{
return Convert.ToInt32(this.DateString.Substring(4, 2));
}
}
public int Day
{
get
{
return Convert.ToInt32(this.DateString.Substring(6, 2));
}
}
public int Hours
{
get
{
return Convert.ToInt32(this.DateString.Substring(8, 2));
}
}
public int Minutes
{
get
{
return Convert.ToInt32(this.DateString.Substring(10, 2));
}
}
}
}
但是我怎样才能使用相同的代码来下载和保存图像呢?
试过这个但得到异常:
private void Download()
{
using (WebClient client = new WebClient()) // WebClient class inherits IDisposable
{
client.DownloadFile("https://something.com", @"C:\temp\localfile.html");
}
}
System.Net.WebException:“远程服务器返回错误:(500)内部服务器错误。”
我可以获取日期和时间,但无法获取提取图像链接的来源。
其中一张图片的链接示例应该如何构建,如下所示:
https://something.com/image?type=infraPolair®ion=tu×tamp=202012150230
但我想从页面中自动提取所有图像的日期和时间,然后自动构建链接,然后下载图像。
第一个代码我可以获得每个图像的日期和时间,但我无法下载页面的源代码,因此我无法提取和构建图像的链接。
这就是为什么我想以某种方式使用第一个代码来构建图像链接然后下载图像。
不完全确定您想通过日期时间实现什么,但使用 HAP 您可以执行以下操作:
HtmlElementCollection elements = doc.DocumentNode.SelectNodes("//img");
foreach (HtmlElement imageElement in elements)
{
var imageSrc = imageElement.Attributes["src"].Value
Download(imageSrc);
}
…………
private void Download(src)
{
using (WebClient client = new WebClient()) // WebClient class inherits IDisposable
{
client.DownloadFile(src, @"C:\temp\" + uniqueNameForFile + ".jpg");
}
}
这不是一个完美的答案,但应该让你继续。 Src 可能是相对的,因此您可能必须添加基地址。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.