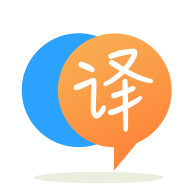
[英]how to count the total number of items for the lists contain a specific item in python
[英]How to count number of a specific item in list? Python3
下面的代码将允许用户在列表中输入指定数量的人。 每个人都有三个属性:姓名、性别和年龄。 代码应该计算列表中“m”字符的数量并计算列表中“f”字符的数量,但是当你到达计数行时会出错。 我将如何解决这个问题?
list1 = []
person = dict()
n = int(input("Enter number of elements: "))
for i in range(0, n):
print ("Enter information :")
person[i] = input("Enter name: "), input("Enter sex (m or f): "), input("Enter age: ")
list1.append(person[i])
i = i + 1
print (list1)
print ("Number of males = " + list1.count('m'))
print ("Number of females = " + list1.count('f'))
尝试这个:
print("Number of males = " + str([i[1] for i in list1].count("m")))
print("Number of females = " + str([i[1] for i in list1].count('f')))
或者,您可以使用默认模块 «collections» 中的计数器
from collections import Counter
li = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4,]
Counter(li)
这将输出Counter({1: 1, 2: 2, 3: 3, 4: 4})
。 计数器 output 是一个dict
,但是,您可以更新它或 select 最常见的项目。
这里有几个问题:
第二条print
指令引发以下错误TypeError: can only concatenate str (not "int") to str
。 这是因为您试图连接 integer 和字符串。 为此,您应该将 integer 转换为如下字符串: print ("Number of males = " + str(list1.count('m')))
无论用户输入是什么, list1.count('m')
始终为 0,因为list1
包含元组,而您正在查找字符串的计数。 您可以通过一个简单的循环获得仅包含性别的列表: [i[1] for i in list1]
固定代码
list1 = []
person = dict()
n = int(input("Enter number of elements: "))
for i in range(0, n):
print ("Enter information :")
person[i] = input("Enter name: "), input("Enter sex (m or f): "), input("Enter age: ")
list1.append(person[i])
i = i + 1
print (list1)
genders=[i[1] for i in list1]
print("Number of males = " + str(genders.count("m")))
print("Number of females = " + str(genders.count('f')))
使用collections.Counter
对对象进行计数。 我还稍微重构了您的代码以实现您的意图:
from collections import Counter
people = []
n = int(input("Enter number of people: "))
for i in range(0, n):
print ("Enter information:")
person = (input("Enter name: "),
input("Enter sex (m or f): "),
int(input("Enter age: ")))
people.append(person)
print (people)
c = Counter([p[1] for p in people])
print ("Number of males = " + str(c['m']))
print ("Number of females = " + str(c['f']))
以下是一些评论,其中包含改进代码的建议:
# make variable name more descriptive, for example users or people:
list1 = []
# no need for defining it here, abd BTW it is a tuple later, not dict:
person = dict()
# elements should be more descriptive, for example users or people:
n = int(input("Enter number of elements: "))
for i in range(0, n):
print ("Enter information :")
# Add int conversion to age: 'int(input("Enter age: "))'.
# Also, person[i] should just one person (a single tuple)
person[i] = input("Enter name: "), input("Enter sex (m or f): "), input("Enter age: ")
list1.append(person[i])
# no need for this, since i is incremented in the statement: 'for i in range(0, n)'
i = i + 1
print (list1)
# Use collections.Counter instead.
# Also count the second element of each element of the list.
print ("Number of males = " + list1.count('m'))
print ("Number of females = " + list1.count('f'))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.