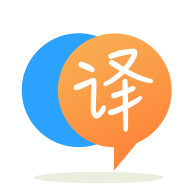
[英]Define a main function in python which can run other functions in python
[英]Can't run multiple functions in a main function in Python
所以我面临的一个问题是:
我定义了 2 个函数和一个 function 使用另一个 function 的变量现在当我使用以下代码运行它们时,它可以正常工作:
def type_anything():
use = input(">")
return use
def print_it():
print(use)
if __name__ == '__main__':
while True:
use = type_anything()
print_it()
Output:
> abcd
abcd
> efgh
efgh
> anything
anything
但是,当我决定创建一个主 function 来运行上述两个函数,然后在“ if __name__ ==
......”行下运行主 function,如下所示:
def type_anything():
use = input("> ")
return use
def print_it():
print(use)
def run_it():
while True:
use = type_anything()
print_it()
if __name__ == '__main__':
run_it()
该程序无法正常运行,而是显示此错误:
> anything
Traceback (most recent call last):
File "C:/<location>/sample.py", line 17, in <module>
run_it()
File "C:/<location>/sample.py", line 13, in run_it
print_it()
File "C:/<location>/sample.py", line 7, in print_it
print(use)
NameError: name 'use' is not defined
为什么会这样? 我需要做什么?
您不能在另一个 function 中使用一个 function 中定义的变量。
每个 function 都需要接收它使用的 arguments。
def type_anything():
use = input(">")
return use
def print_it(use):
print(use)
if __name__ == '__main__':
while True:
use = type_anything()
print_it(use)
这应该可以解决您的问题:
def type_anything():
use = input("> ")
return use
def print_it(use):
print(use)
def run_it():
while True:
use = type_anything()
print_it(use)
if __name__ == '__main__':
run_it()
print_it
function 不知道任何变量的use
,因此会出现错误。
注意type_anything
function 如何returns
变量use
和print_it
function 接受一个参数然后打印它。
请不要养成使用全局变量的习惯,从长远来看,这会破坏您编写的所有内容。 这只是帮助您理解问题的示例!
您的问题是变量 scope。 在第一个示例中,您的变量use
是global
的,因为您在主程序中定义了它:
if __name__ == '__main__':
while True:
use = type_anything()
print_it()
它没有在type_anything
中定义,你可以将这个变量重命名为任何东西,它仍然可以工作:
def type_anything():
x = input(">")
return x
在第二个示例中,您在 function 中定义它:
def run_it():
while True:
use = type_anything()
print_it()
您可以通过use
全局变量来解决此问题:
def run_it():
global use
while True:
use = type_anything()
print_it()
一种更好的方法
将变量use
传递给使用它的 function:
def print_it(use):
print(use)
def run_it():
while True:
use = type_anything()
print_it(use)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.