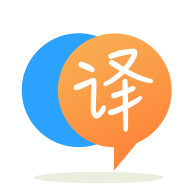
[英]How to compile this example with async_read_until, async_write and Boost.Asio?
[英]How should I use async_read_until and async_write simultaneously in the client app in boost::asio?
我正在尝试构建一个简单的信使。 目前,我已经编写了支持“类似功能的邮件”的客户端和服务器应用程序,即它们缺少您在每个即时消息中拥有的聊天交互。
服务器:每个连接的客户端的服务器都有一个提供实际服务的专用Service
class。 Service
class 的实例有一个 id。
Client :在特定时刻同时开始从关联的 Service 实例读取消息并将消息写入关联的Service
实例。
跟踪器:通过将用户的登录名和Service
ID 保存在 map 中来记录用户的当前会话。 还通过保存键值对(聊天参与者 id 1,聊天参与者 id 2)记录打开的聊天。 我可以互换使用用户的登录名和 ID,因为我有一个数据库。
Service
实例专用于该用户。然后将用户标识为 Bob。Tracker
记录 Bob 使用Service
1 以及 Bob 打开了与 Ann 的聊天。Service
实例专用于用户。然后用户标识为 Ann。Tracker
记录了 Ann 使用Service
2 以及 Ann 打开了与 Bob 的聊天。Service
2 会收到消息并要求Service
1 将消息发送到 Bob 的聊天。 为此,我使用Tracker
。 在我们的例子中,Bob 正在聊天中,所以 Bob 的客户端应用程序应该从Service
1 读取消息。否则, Service
2 只会将新消息存储在数据库中。 当用户打开与某人的聊天时,客户端应用程序同时开始向关联的Service
实例读取和写入消息。
这是我的服务器代码的一部分。 我添加了一些上下文,但您可能想查看Service::onMessageReceived
、 Service::receive_message
、 Service::send_to_chat
/// Struct to track active sessions of clients
struct Tracker {
static std::mutex current_sessions_guard; ///< mutex to lock the map of current sessions between threads
static std::map<long, long> current_sessions;
static std::map<long, int> client_to_service_id;
};
Class 在客户端服务 model 中提供实际服务
class Service {
public:
void send_to_chat(const std::string& new_message) {
asio::async_write(*m_sock.get(), asio::buffer(new_message),
[this]() {
onAnotherPartyMessageSent();
});
}
private:
void onReceivedReady();
void receive_message() {
/// Server loop for reading messages from the client
spdlog::info("[{}] in receive_message", service_id);
asio::async_read_until(*m_sock.get(), *(m_message.get()), '\n',
[this]() {
onMessageReceived();
});
}
void onMessageReceived();
private:
std::shared_ptr<asio::ip::tcp::socket> m_sock; ///< Pointer to an active socket that is used to communicate
///< with the client
int service_id;
long dialog_id = -1, client_id = -1, another_party_id = -1;
std::shared_ptr<asio::streambuf> m_message;
};
方法的定义
void Service::onMessageReceived() {
/// Updates the database with the new message and asks Service instance of another participant
/// to send the message if they opened this chat.
std::istream istrm(m_message.get());
std::string new_message;
std::getline(istrm, new_message);
m_message.reset(new asio::streambuf);
std::unique_lock<std::mutex> tracker_lock(Tracker::current_sessions_guard);
if (Tracker::current_sessions.find(another_party_id) != Tracker::current_sessions.end()) {
if (Tracker::current_sessions[another_party_id] == client_id) {
int another_party_service_id = Tracker::client_to_service_id[another_party_id];
std::string formatted_msg = _form_message_str(login, new_message);
spdlog::info("[{}] sends to chat '{}'", another_party_service_id, new_message);
Server::launched_services[another_party_service_id]->send_to_chat(formatted_msg);
}
}
tracker_lock.unlock();
receive_message();
}
这是我的客户端代码的一部分。 我添加了一些上下文,但您可能想查看AsyncTCPClient::onSentReady
、 AsyncTCPClient::message_send_loop
、 AsyncTCPClient::message_wait_loop
。
/// Struct that stores a session with the given server
struct Session {
asio::ip::tcp::socket m_sock; //!< The socket for the client application to connect to the server
asio::ip::tcp::endpoint m_ep; //!< The server's endpoint
std::string current_chat;
std::shared_ptr<asio::streambuf> m_chat_buf;
std::shared_ptr<asio::streambuf> m_received_message;
};
/// Class that implements an asynchronous TCP client to interact with Service class
class AsyncTCPClient: public asio::noncopyable {
void onSentReady(std::shared_ptr<Session> session) {
msg_wait_thread.reset(new std::thread([this, session] {
asio::async_read_until(session->m_sock, *(session->m_received_message.get()), "\n",
[this, session] () {
message_wait_loop(session);
});
}));
msg_wait_thread->detach();
msg_thread.reset(new std::thread([this, session] {
message_send_loop(session);
}));
msg_thread->detach();
}
void message_send_loop(std::shared_ptr<Session> session) {
/// Starts loop in the current chat enabling the client to keep sending messages to another party
logger->info("'{}' in message_send_loop", session->login);
clear_console();
m_console.write(session->current_chat);
m_console.write("Write your message: ");
std::string new_message;
// We use a do/while loop to prevent empty messages either because of the client input
// or \n's that were not read before
do {
new_message = m_console.read();
} while (new_message.empty());
std::unique_lock<std::mutex> lock_std_out(std_out_guard);
session->current_chat.append(_form_message_str(session->login, new_message));
lock_std_out.unlock();
asio::async_write(session->m_sock, asio::buffer(new_message + "\n"),
[this, session] () {
message_send_loop(session);
});
}
void message_wait_loop(std::shared_ptr<Session> session) {
/// Starts loop in the current chat enabling the client to keep reading messages from another party
logger->info("'{}' in message_wait_loop", session->login);
std::istream istrm(session->m_received_message.get());
std::string received_message;
std::getline(istrm, received_message);
session->m_received_message.reset(new asio::streambuf);
std::unique_lock<std::mutex> lock_std_out(std_out_wait_guard);
session->current_chat.append(received_message + "\n");
lock_std_out.unlock();
clear_console();
m_console.write(session->current_chat);
m_console.write("Write your message: ");
asio::async_read_until(session->m_sock, *(session->m_received_message.get()), "\n",
[this, session] (std::size_t) {
message_wait_loop(session);
});
}
private:
asio::io_context m_ios;
};
因此,当我描述该问题时,我在第 3 点的两个客户端都没有"'{}' in message_wait_loop"
日志)。 但是我在第 2 点为 Bob 的客户提供了这些日志。
我也在这里使用答案中的控制台。 它通过互斥体去除回声并控制标准输入/输出资源。 但是它并不能解决我的问题。
任何帮助,将不胜感激。
代码太多而太少。 这个问题太多了,而实际上建议改进的太少了。 我看到过度使用 shared_ptr,线程,特别是在他们自己的线程上运行异步操作非常奇怪。 更别说分离了:
msg_wait_thread.reset(new std::thread([this, session] {
asio::async_read_until(session->m_sock, *(session->m_received_message.get()), "\n",
[this, session] () {
message_wait_loop(session);
});
}));
msg_wait_thread->detach();
整个事情最好用完全等效的(但更安全)代替
asio::async_read_until(session->m_sock, *(session->m_received_message.get()), "\n",
[this, session] () {
message_wait_loop(session);
});
我想读循环在一个线程上,这样输入就不会阻塞。 但是,如果您将主线程视为“UI 线程”(确实如此)并接受控制台 IO 在那里阻塞,而不是将结果请求发布到单个 IO 线程以进行所有非阻塞操作,则会变得容易得多。
如果您分享指向回购或其他内容的链接,我很乐意多看它。
在评论中,我查看了 github 存储库中的代码并发布了 PR: https://github.com/cepessh/mymsg/pull/1
这是一个非常原始的概念验证。 我已经包含了许多实际上与建议的并发修复无关的更改,但它们发生了:
- 让我跑
- 在审查期间(您可能希望查看其中的一些更改并保留它们)
main
分支中明显缺失的修复(例如Message.read_by_recipient
数据库列的默认值)您应该能够通过提交消息了解进行了哪些更改以及更改的原因。
只有最后两个提交真正关注聊天中讨论的想法。
![]()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.