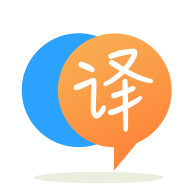
[英]issue passing data back to first view controller with delegate and protocol (Swift)
[英]Passing Data From View Controller B using a button to View Contoller A with Tableview with delegate- protocol
我正在寻找如何将数据从一个子视图 controller 传递到父视图 controller。 它们都包含 tableViews。 我想 select 视图 Controller B 中的单元格,然后单击按钮并将该数据发送回视图 controller A。我正在使用代表和协议。 我的问题是我不确定在 IBAction 中使用什么按钮来使其显示在父视图 controller 中。
我的看法 Controller
class ViewController: UIViewController, DataEnteredDelegate, UITableViewDelegate, UITableViewDataSource, DataSelectedDelegate {
@IBOutlet weak var tableView: UITableView!
var tableArrayHeader = [String]()
var tableViewArray = [String]()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "showSecondViewController", let secondViewController = segue.destination as? SecondViewController {
secondViewController.delegate = self
} else {
if segue.identifier == "showThirdViewController", let thirdViewController = segue.destination as? ThirdViewController {
thirdViewController.selectedDelegate = self
}
}
}
// required method of our custom DataEnteredDelegate protocol
func userDidEnterInformation(info: String) {
navigationController?.popViewController(animated: true)
self.tableArrayHeader.append(info)
self.tableView.reloadData()
}
func userDidSelectInformation(selection: String) {
navigationController?.popViewController(animated: true)
self.tableArrayHeader.append(selection)
self.tableView.reloadData()
}
@IBAction func addingButton(_ sender: Any) {
tableViewArray.insert("new Cell", at: 0)
tableView.insertRows(at: [IndexPath(row: 0, section: 0)], with: .right)
}
func numberOfSections(in tableView: UITableView) -> Int {
return tableArrayHeader.count
}
func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
return tableArrayHeader[section]
}
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
let cell = tableView.dequeueReusableCell(withIdentifier: "headerCell") as? CustomHeaderTableViewCell
cell?.headerLabel?.text = tableArrayHeader[section]
cell?.backgroundColor = UIColor.lightGray
return cell
}
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return 55
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return tableViewArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as! FirstTableViewCell
cell.label.text = tableViewArray[indexPath.row]
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 55
}
// func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
// tableView.deselectRow(at: indexPath, animated: true)
// }
func tableView(_ tableView: UITableView, editingStyleForRowAt indexPath: IndexPath) -> UITableViewCell.EditingStyle {
return .delete
}
func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCell.EditingStyle, forRowAt indexPath: IndexPath) {
if editingStyle == .delete {
tableView.beginUpdates()
tableViewArray.remove(at: indexPath.row)
tableView.deleteRows(at: [indexPath], with: .bottom)
tableView.endUpdates()
} else{
print("there are no cells to delete")
}
}
}
查看 Controller B:
protocol DataSelectedDelegate {
func userDidSelectInformation(selection: String)
}
class ThirdViewController: ViewController {
var selectedDelegate: DataSelectedDelegate?
struct Objects {
var sectionName : String!
var sectionObjects : [String]!
}
var objectArray = [Objects]()
var tableArray = ["this","is","test","date"]
var names = ["Vegetables": ["Tomato", "Potato", "Lettuce"], "Fruits": ["Apple", "Banana"]]
//var objectArray = [String]()
var selectionArray : [String] = []
var selectedIndexPath = [IndexPath]()
var selectedIndex = -1
@IBOutlet weak var thirdTable: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
for (key, value) in names {
print("\(key) -> \(value)")
objectArray.append(Objects(sectionName: key, sectionObjects: value))
}
}
@IBAction func sendDataFromTableToTable(_ sender: Any) {
selectedDelegate?.userDidSelectInformation(selection: // This is where i am unsure of what to put so that the selected cell data will go back when the button is pressed "" )
}
// @IBAction func sendDataFromRowToTable(_ sender: Any) {
// selectedDelegate?.userDidSelectInformation(selection: selectedIndexPath.description ?? "" )
// }
override func numberOfSections(in tableView: UITableView) -> Int {
return objectArray.count
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return objectArray[section].sectionObjects.count
//return tableArray.count
}
override func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
return objectArray[section].sectionName
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 55
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "thirdCell", for: indexPath)
cell.textLabel?.text = objectArray[indexPath.section].sectionObjects[indexPath.row]
cell.textLabel?.text = tableArray[indexPath.row]
if selectedIndexPath.contains(indexPath) {
cell.accessoryType = .checkmark
} else {
cell.accessoryType = .none
}
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
if selectedIndexPath.contains(indexPath) {
selectedIndexPath.removeAll() {
(selectedIndexPath) -> Bool in
selectedIndexPath == indexPath
}
thirdTable.reloadRows(at: [indexPath], with: .fade)
print(selectedIndexPath.description)
} else {
selectedIndexPath.append(indexPath)
thirdTable.reloadRows(at: [indexPath], with: .fade)
}
selectionArray.append(tableArray[indexPath.row])
print(selectionArray)
}
func tableView(_ tableView: UITableView, didDeselectRowAt indexPath: IndexPath) {
if let index = selectionArray.firstIndex(of: tableArray[indexPath.row]) {
selectionArray.remove(at: index)
print(tableArray)
}
}
}
控制器图片:
UITableView 有一个属性indexPathForSelectedRow
可以让您询问选择了哪一行。
在您的按钮操作中,检查该属性。 如果为 nil,则不选择任何单元格。 如果不为零,请使用 indexPath 从 model 中获取数据并将其传递回第一个视图 controller。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.