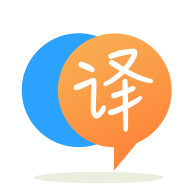
[英]How to make a second api call with data received from the first api call in Apollo client with React?
[英]React.JS, how to edit the response of a first API call with data from a second API call?
我需要在我的组件中显示一些数据,不幸的是,第一次调用我的 API 只返回了我想要显示的部分信息,以及一些 ID。 我需要再次调用这些 ID 来检索其他有意义的数据。 第一个调用包含在 useEffect() React.js function 中:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get(
'/myapi/' + auth.authState.id
);
setData(data);
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
并返回一个对象数组,每个 object 代表给定 Employee 的约会,如下所示:
[
{
"appointmentID": 1,
"employeeID": 1,
"customerID": 1,
"appointmentTime": "11:30",
"confirmed": true
},
... many more appointments
]
现在我还想检索有关客户的信息,例如姓名、电话号码等。我尝试设置另一种方法,例如getData()
,当我遍历各种约会以将它们显示为时,它会返回我需要的信息表格的行,但我学到了在渲染方法中调用的函数不应该有任何副作用的艰难方法。 进行另一个 API 调用的最佳方法是什么,将每个“customerID”替换为存储客户 ID + 其他数据的 object?
[在我尝试过的方法之下,返回一个 [Object Promise]]
const AppointmentElements = () => {
//Loop through each Appointment to create a single row
var output = Object.values(data).map((i) =>
<Appointment
key={i['appointmentID'].toString()}
employee={i["employeeID"]} //returned a [Object premise]
customer={getEmployeeData((i['doctorID']))} //return a [Object Promise]
time={index['appointmentTime']}
confirmed = {i['confirmed']}
/>
);
return output;
};
正如您自己提到的,在渲染方法中调用的函数不应该有任何副作用,您不应该在渲染中调用getEmployeeData
function。
What you can do is, inside the same useEffect
and same getData
where you are calling the first api, call the second api as well, nested within the first api call and put the complete data in a state variable. 然后在 render 方法中,循环遍历这个完整的数据,而不是仅来自第一个 api 的数据。
如果您在 getData 中调用第二个 api 时需要帮助,请告诉我,我会为您提供代码帮助。
您的useEffect
应该类似于:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get('/myapi/' + auth.authState.id);
const updatedData = data.map(value => {
const { data } = await fetchContext.authAxios.get('/mySecondApi/?customerId=' + value.customerID);
// please make necessary changes to the api call
return {
...value, // de-structuring
customerID: data
// as you asked customer data should replace the customerID field
}
}
);
setData(updatedData); // this data would contain the other details of customer in it's customerID field, along with all other fields returned by your first api call
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
这是假设您有一个 api 一次只接受一个客户 ID。
如果您有更好的 api 接受客户 ID 列表,则可以将上述代码修改为:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get('/myapi/' + auth.authState.id);
const customerIdList = data.map(value => value.customerID);
// this fetches list of all customer details in one go
const customersDetails = (await fetchContext.authAxios.post('/mySecondApi/', {customerIdList})).data;
// please make necessary changes to the api call
const updatedData = data.map(value => {
// filtering the particular customer's detail and updating the data from first api call
const customerDetails = customersDetails.filter(c => c.customerID === value.customerID)[0];
return {
...value, // de-structuring
customerID: customerDetails
// as you asked customer data should replace the customerID field
}
}
);
setData(updatedData); // this data would contain the other details of customer in it's customerID field, along with all other fields returned by your first api call
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
如果您的 api 支持,这将减少网络调用的数量和通常首选的方式。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.