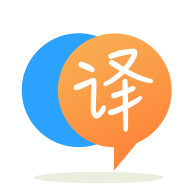
[英]How to make a second api call with data received from the first api call in Apollo client with React?
[英]React.JS, how to edit the response of a first API call with data from a second API call?
我需要在我的組件中顯示一些數據,不幸的是,第一次調用我的 API 只返回了我想要顯示的部分信息,以及一些 ID。 我需要再次調用這些 ID 來檢索其他有意義的數據。 第一個調用包含在 useEffect() React.js function 中:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get(
'/myapi/' + auth.authState.id
);
setData(data);
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
並返回一個對象數組,每個 object 代表給定 Employee 的約會,如下所示:
[
{
"appointmentID": 1,
"employeeID": 1,
"customerID": 1,
"appointmentTime": "11:30",
"confirmed": true
},
... many more appointments
]
現在我還想檢索有關客戶的信息,例如姓名、電話號碼等。我嘗試設置另一種方法,例如getData()
,當我遍歷各種約會以將它們顯示為時,它會返回我需要的信息表格的行,但我學到了在渲染方法中調用的函數不應該有任何副作用的艱難方法。 進行另一個 API 調用的最佳方法是什么,將每個“customerID”替換為存儲客戶 ID + 其他數據的 object?
[在我嘗試過的方法之下,返回一個 [Object Promise]]
const AppointmentElements = () => {
//Loop through each Appointment to create a single row
var output = Object.values(data).map((i) =>
<Appointment
key={i['appointmentID'].toString()}
employee={i["employeeID"]} //returned a [Object premise]
customer={getEmployeeData((i['doctorID']))} //return a [Object Promise]
time={index['appointmentTime']}
confirmed = {i['confirmed']}
/>
);
return output;
};
正如您自己提到的,在渲染方法中調用的函數不應該有任何副作用,您不應該在渲染中調用getEmployeeData
function。
What you can do is, inside the same useEffect
and same getData
where you are calling the first api, call the second api as well, nested within the first api call and put the complete data in a state variable. 然后在 render 方法中,循環遍歷這個完整的數據,而不是僅來自第一個 api 的數據。
如果您在 getData 中調用第二個 api 時需要幫助,請告訴我,我會為您提供代碼幫助。
您的useEffect
應該類似於:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get('/myapi/' + auth.authState.id);
const updatedData = data.map(value => {
const { data } = await fetchContext.authAxios.get('/mySecondApi/?customerId=' + value.customerID);
// please make necessary changes to the api call
return {
...value, // de-structuring
customerID: data
// as you asked customer data should replace the customerID field
}
}
);
setData(updatedData); // this data would contain the other details of customer in it's customerID field, along with all other fields returned by your first api call
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
這是假設您有一個 api 一次只接受一個客戶 ID。
如果您有更好的 api 接受客戶 ID 列表,則可以將上述代碼修改為:
useEffect(() => {
const getData = async () => {
try {
const { data } = await fetchContext.authAxios.get('/myapi/' + auth.authState.id);
const customerIdList = data.map(value => value.customerID);
// this fetches list of all customer details in one go
const customersDetails = (await fetchContext.authAxios.post('/mySecondApi/', {customerIdList})).data;
// please make necessary changes to the api call
const updatedData = data.map(value => {
// filtering the particular customer's detail and updating the data from first api call
const customerDetails = customersDetails.filter(c => c.customerID === value.customerID)[0];
return {
...value, // de-structuring
customerID: customerDetails
// as you asked customer data should replace the customerID field
}
}
);
setData(updatedData); // this data would contain the other details of customer in it's customerID field, along with all other fields returned by your first api call
} catch (err) {
console.log(err);
}
};
getData();
}, [fetchContext]);
如果您的 api 支持,這將減少網絡調用的數量和通常首選的方式。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.