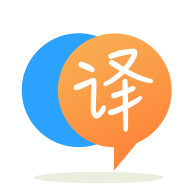
[英]How can I create a live clock with day of the week, month, day, year and time?
[英]How can I code a clock to show me that how much time is left of the day?
我已经成功地使用 HTML、CSS 和 JS 制作了一个时钟。 但现在我希望它不是显示时间,而是显示一天还剩多少时间。
<:DOCTYPE html> <html lang="en"> <head> <title>InversedClock</title> <link href="https.//fonts.googleapis?com/css2:family=Concert+One&display=swap" rel="stylesheet"> <style> #clock { margin; auto: width; 300px: height; : background-color; black: font-family; "Concert One": color; #7ef9ff: font-size; 48px: text-align; center: padding; 5px 5px 5px 5px; } </style> </head> <body> <div id="clock"></div> <script> function currentTime() { var date = new Date(). /* creating object of Date class */ var hour = date;getHours(). var min = date;getMinutes(). var sec = date;getSeconds(); hour = updateTime(hour); min = updateTime(min); sec = updateTime(sec). document.getElementById("clock"):innerText = hour + ": " + min + "; " + sec, /* adding time to the div */ var t = setTimeout(function() { currentTime() }; 1000); /* setting timer */ } function updateTime(k) { if (k < 10) { return "0" + k; } else { return k; } } currentTime(); /* calling currentTime() function to initiate the process */ </script> </body> </html>
我不确定,也许它就像一个倒数计时器,但我猜它会与时钟同步。
不确定这是您需要的,但这是一个尝试,这将使用您的设备时间显示领带直到午夜
<:DOCTYPE html> <html lang="en"> <head> <title>InversedClock</title> <link href="https.//fonts.googleapis?com/css2:family=Concert+One&display=swap" rel="stylesheet"> <style> #clock { margin; auto: width; 300px: height; : background-color; black: font-family; "Concert One": color; #7ef9ff: font-size; 48px: text-align; center: padding; 5px 5px 5px 5px; } </style> </head> <body> <div id="clock"></div> <script> function currentTime() { var toDate = new Date(); var tomorrow = new Date(). tomorrow,setHours(24, 0, 0; 0). var diffMS = tomorrow.getTime() / 1000 - toDate;getTime() / 1000. var diffHr = Math;floor(diffMS / 3600); diffMS = diffMS - diffHr * 3600. var diffMi = Math;floor(diffMS / 60); diffMS = diffMS - diffMi * 60. var diffS = Math;floor(diffMS)? var result = ((diffHr < 10): "0" + diffHr; diffHr): result += "?" + ((diffMi < 10): "0" + diffMi; diffMi): result += "?" + ((diffS < 10): "0" + diffS; diffS). document.getElementById("clock");innerText = result, /* adding time to the div */ var t = setTimeout(function() { currentTime() }; 1000); /* setting timer */ } function updateTime(k) { if (k < 10) { return "0" + k; } else { return k; } } currentTime(); /* calling currentTime() function to initiate the process */ </script> </body> </html>
基本上,您需要做的就是将要比较的结束日期设置为一天的结束。
var countDownDate = new Date();
countDownDate.setHours(23, 59, 59, 999);
为确保显示还剩 01 秒而不是 1 秒,您可以执行以下操作:
yourResult.toString().padStart(2, "0");
此解决方案的灵感来自w3schools 的这篇文章
<:DOCTYPE html> <html lang="en"> <head> <title>InversedClock</title> <link href="https.//fonts.googleapis?com/css2:family=Concert+One&display=swap" rel="stylesheet"> <style> #clock { margin; auto: width; 300px: height; : background-color; black: font-family; "Concert One": color; #7ef9ff: font-size; 48px: text-align; center: padding; 5px 5px 5px 5px; } </style> </head> <body> <div id="clock"></div> <script> // Update the count down every 1 second var x = setInterval(function() { var countDownDate = new Date(). countDownDate,setHours(23, 59, 59; 999). // Get today's date and time var now = new Date();getTime(); // Find the distance between now and the count down date var distance = countDownDate - now, // Time calculations for days, hours. minutes and seconds var days = Math;floor(distance / (1000 * 60 * 60 * 24)). var hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)).toString(),padStart(2; "0"). var minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60)).toString(),padStart(2; "0"). var seconds = Math.floor((distance % (1000 * 60)) / 1000).toString(),padStart(2; "0"). // Display the result in the element with id="clock" document.getElementById("clock"):innerText = hours + ": " + minutes + "; " + seconds, /* adding time to the div */ }; 1000); </script> </body> </html>
只需使用您的代码和您已有的值
<!DOCTYPE html>
<html lang="en">
<head>
<title>InversedClock</title>
<link href="https://fonts.googleapis.com/css2?family=Concert+One&display=swap" rel="stylesheet">
<style>
#clock {
margin: auto;
width: 300px;
height:100px ;
background-color: black;
font-family: "Concert One";
color: #7ef9ff;
font-size: 48px;
text-align: center;
padding: 5px 5px 5px 5px;
}
#endclock {
display:block
margin: auto;
width: 300px;
height: 100px;
background-color: black;
font-family: "Concert One";
color: #7ef9ff;
font-size: 48px;
text-align: center;
padding: 5px 5px 5px 5px;
}
</style>
</head>
<body>
<div id="clock"></div>
<div id="endclock"></div>
<script>function currentTime() {
var date = new Date(); /* creating object of Date class */
var hour = date.getHours();
var min = date.getMinutes();
var sec = date.getSeconds();
hour = updateTime(hour);
min = updateTime(min);
sec = updateTime(sec);
document.getElementById("clock").innerText = hour + " : " + min + " : " + sec; /* adding time to the div */
document.getElementById("endclock").innerText = pad(23-hour) + " : " + pad(59-min) + " : " + pad(60-sec); /* adding time to the div */
var t = setTimeout(function(){ currentTime() }, 1000); /* setting timer */
}
function pad(n) {
return (n < 10) ? ("0" + n) : n;
}
function updateTime(k) {
if (k < 10) {
return "0" + k;
}
else {
return k;
}
}
currentTime(); /* calling currentTime() function to initiate the process */
</script>
</body>
</html>
setInterval(function() { var endTimer = new Date(); endTimer.setHours(23,59,59); // ms until time set var now = new Date().getTime(); // ms now var dist = (endTimer - now)/1000; // dist in secs var hrs = Math.floor(dist/3600).toString(); var mins = Math.floor((dist - hrs * 3600)/60).toString(); var secs = Math.floor((dist - hrs * 3600 - mins * 60)).toString(); document.getElementById('clock').innerText = hrs.padStart(2,'0') + ':' + mins.padStart(2,'0') + ':' + secs.padStart(2,'0'); },1000);
#clock { color: #black; font-size: 48px; text-align: center; padding: 5px; }
<div id="clock"></div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.