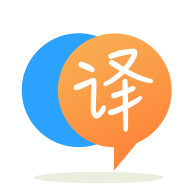
[英]Java.Lang.NoSuchMethodError: 'no non-static method “Landroid/widget/TextView;.setJustificationMode(I)V”'
[英]AndroidJavaException: java.lang.NoSuchMethodError: no non-static method with name='getStatusCode' signature='()I'
当我尝试使用 Google Play 游戏插件(即使我已登录)保存或加载我的游戏时,logcat 会向我显示: 尝试了不同的方法来保存游戏,但它一次又一次地显示。 PS成就和排行榜工作正常。
这是我调用保存和加载过程的脚本:
#if UNITY_ANDROID
using UnityEngine;
using System;
using System.Collections.Generic;
//gpg
using GooglePlayGames;
using GooglePlayGames.BasicApi;
using GooglePlayGames.BasicApi.SavedGame;
//for encoding
using System.Text;
//for extra save ui
using UnityEngine.SocialPlatforms;
//for text, remove
using UnityEngine.UI;
public class SaveManager :MonoBehaviour{
private static SaveManager _instance;
public static SaveManager Instance{
get{
if (_instance == null) {
_instance = new SaveManager();
}
return _instance;
}
}
//keep track of saving or loading during callbacks.
private bool m_saving;
//save name. This name will work, change it if you like.
private static string m_saveName = "game_save_name";
//This is the saved file. Put this in seperate class with other variables for more advanced setup. Remember to change merging, toBytes and fromBytes for more advanced setup.
private string saveString = "";
//check with GPG (or other*) if user is authenticated. *e.g. GameCenter
private bool Authenticated {
get {
return Social.Active.localUser.authenticated;
}
}
//merges loaded bytearray with old save
private void ProcessCloudData(byte[] cloudData) {
if (cloudData == null) {
Debug.Log("No data saved to the cloud yet...");
return;
}
Debug.Log("Decoding cloud data from bytes.");
string progress = FromBytes(cloudData);
Debug.Log("Merging with existing game progress.");
MergeWith(progress);
}
//load save from cloud
public void LoadFromCloud(){
Debug.Log("Loading game progress from the cloud.");
m_saving = false;
((PlayGamesPlatform)Social.Active).SavedGame.OpenWithAutomaticConflictResolution(
m_saveName, //name of file.
DataSource.ReadCacheOrNetwork,
ConflictResolutionStrategy.UseLongestPlaytime,
SavedGameOpened);
}
//overwrites old file or saves a new one
public void SaveToCloud() {
if (Authenticated) {
Debug.Log("Saving progress to the cloud... filename: " + m_saveName);
m_saving = true;
//save to named file
((PlayGamesPlatform)Social.Active).SavedGame.OpenWithAutomaticConflictResolution(
m_saveName, //name of file. If save doesn't exist it will be created with this name
DataSource.ReadCacheOrNetwork,
ConflictResolutionStrategy.UseLongestPlaytime,
SavedGameOpened);
} else {
Debug.Log("Not authenticated!");
}
}
//save is opened, either save or load it.
private void SavedGameOpened(SavedGameRequestStatus status, ISavedGameMetadata game) {
//check success
if (status == SavedGameRequestStatus.Success){
//saving
if (m_saving){
//read bytes from save
byte[] data = ToBytes();
//create builder. here you can add play time, time created etc for UI.
SavedGameMetadataUpdate.Builder builder = new SavedGameMetadataUpdate.Builder();
SavedGameMetadataUpdate updatedMetadata = builder.Build();
//saving to cloud
((PlayGamesPlatform)Social.Active).SavedGame.CommitUpdate(game, updatedMetadata, data, SavedGameWritten);
//loading
} else {
((PlayGamesPlatform)Social.Active).SavedGame.ReadBinaryData(game, SavedGameLoaded);
}
//error
} else {
Debug.LogWarning("Error opening game: " + status);
}
}
//callback from SavedGameOpened. Check if loading result was successful or not.
private void SavedGameLoaded(SavedGameRequestStatus status, byte[] data) {
if (status == SavedGameRequestStatus.Success){
Debug.Log("SaveGameLoaded, success=" + status);
ProcessCloudData(data);
} else {
Debug.LogWarning("Error reading game: " + status);
}
}
//callback from SavedGameOpened. Check if saving result was successful or not.
private void SavedGameWritten(SavedGameRequestStatus status, ISavedGameMetadata game) {
if (status == SavedGameRequestStatus.Success){
Debug.Log("Game " + game.Description + " written");
} else {
Debug.LogWarning("Error saving game: " + status);
}
}
//merge local save with cloud save. Here is where you change the merging betweeen cloud and local save for your setup.
private void MergeWith(string other) {
if (other != "") {
saveString = other;
} else {
Debug.Log("Loaded save string doesn't have any content");
}
}
//return saveString as bytes
private byte[] ToBytes() {
byte[] bytes = Encoding.UTF8.GetBytes(saveString);
return bytes;
}
//take bytes as arg and return string
private string FromBytes(byte[] bytes) {
string decodedString = Encoding.UTF8.GetString(bytes);
return decodedString;
}
// -------------------- ### Extra UI for testing ### --------------------
//call this with Unity button to view all saves on GPG. Bug: Can't close GPG window for some reason without restarting.
public void showUI() {
// user is ILocalUser from Social.LocalUser - will work when authenticated
ShowSaveSystemUI(Social.localUser, (SelectUIStatus status, ISavedGameMetadata game) => {
// do whatever you need with save bundle
});
}
//displays savefiles in the cloud. This will only include one savefile if the m_saveName hasn't been changed
private void ShowSaveSystemUI(ILocalUser user, Action<SelectUIStatus, ISavedGameMetadata> callback) {
uint maxNumToDisplay = 3;
bool allowCreateNew = true;
bool allowDelete = true;
ISavedGameClient savedGameClient = PlayGamesPlatform.Instance.SavedGame;
if (savedGameClient != null) {
savedGameClient.ShowSelectSavedGameUI(user.userName + "\u0027s saves",
maxNumToDisplay,
allowCreateNew,
allowDelete,
(SelectUIStatus status, ISavedGameMetadata saveGame) => {
// some error occured, just show window again
if (status != SelectUIStatus.SavedGameSelected) {
ShowSaveSystemUI(user, callback);
return;
}
if (callback != null)
callback.Invoke(status, saveGame);
});
} else {
// this is usually due to incorrect APP ID
Debug.LogError("Save Game client is null...");
}
}
}
#endif
......................
我遇到了同样的问题,这是因为我通过 R8 勾选了 minify,删除了勾选解决了我的问题,我再次对其进行了测试,启用 R8 minify 只是给了我你所拥有的确切例外。
此选项位于player settings
中other settings
下拉列表的末尾,此异常仅在禁用Developement Build
时发生
确保 function header 与Call()
或CallStatic()
方法匹配。 如果您的方法接受任何 arguments 那么您必须创建一个参数数组并将其传递给Call()
方法。 否则它将通过提到的错误。
假设您有一个 [ICODE]PrintString[/ICODE] 方法,它接受两个 arguments。
public void PrintString(Context context, final String message )
{
//create/show an android toast, with the message and short duration.
new Handler(Looper.getMainLooper()).post(new Runnable() {
@Override
public void run() {
Toast.makeText(context, message, Toast.LENGTH_SHORT).show();
}
});
}
然后你必须像这样调用 Unity 应用程序中的方法,
public void ShowToast(string msg) {
if (Application.platform == RuntimePlatform.Android) {
// Retrieve the UnityPlayer class.
AndroidJavaClass unityPlayerClass = new AndroidJavaClass("com.unity3d.player.UnityPlayer");
// Retrieve the UnityPlayerActivity object ( a.k.a. the current context )
AndroidJavaObject unityActivity = unityPlayerClass.GetStatic<AndroidJavaObject>("currentActivity");
// Retrieve the "Bridge" from our native plugin.
// ! Notice we define the complete package name.
AndroidJavaObject alert = new AndroidJavaObject("com.codemaker.mylibrary.Alert");
// Setup the parameters we want to send to our native plugin.
object[] parameters = new object[2];
parameters[0] = unityActivity;
parameters[1] = msg;
// Call PrintString in bridge, with our parameters.
alert.Call("PrintString", parameters);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.