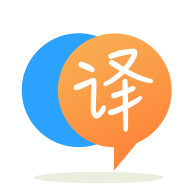
[英]How to handle the lights in PyVista when using a transform matrix for the camera?
[英]How to create shared topology using PyAnsys and PyVista
我使用 PyVista 创建了两个圆柱网格,目的是使用 PyAnsys 在内圆柱(以蓝色显示)和外圆柱(以灰色显示)上执行模态分析。 其中每个圆柱体具有不同的材料特性并构成单个模型的一部分:
网格生成脚本:
import numpy as np
import pyvista as pv
def create_mesh(inner_radius, thickness, height, z_res, c_res, r_res_pipe, r_res_fluid):
# Create a pipe mesh using a structured grid using the specified mesh density:
outer_radius = inner_radius + thickness
# Create a list of radial divisions between the inner and outer radii:
if r_res_pipe % 2 != 0:
r_res_pipe = r_res_pipe + 1 # radial resolution must be even to accommodate array reshaping
radial_divisions = np.linspace(inner_radius, outer_radius, r_res_pipe)
grid = pv.CylinderStructured(
radius=radial_divisions, height=height, theta_resolution=c_res, z_resolution=z_res
)
grid.points = grid.points
original_mesh = pv.UnstructuredGrid(grid)
pipe_mesh = original_mesh
points = pipe_mesh.points.reshape(z_res, c_res, r_res_pipe * 3)
points[:, c_res - 1, :] = points[:, 0, :]
pipe_mesh.points = points.reshape(z_res * (c_res) * 2, int(r_res_pipe + r_res_pipe / 2))
pipe_mesh.plot(color='grey', show_edges=True)
# Create a fluid mesh using a structured grid based on the pipe dimensions:
# Create a list of radial divisions between the inner and outer radii:
if r_res_fluid % 2 != 0:
r_res_fluid = r_res_fluid + 1 # radial resolution must be even to accommodate array reshaping
radial_divisions = np.linspace(0.0, inner_radius, r_res_fluid)
grid = pv.CylinderStructured(
radius=radial_divisions, height=height, theta_resolution=c_res, z_resolution=z_res
)
grid.points = grid.points
original_mesh = pv.UnstructuredGrid(grid)
fluid_mesh = original_mesh
points = fluid_mesh.points.reshape(z_res, c_res, r_res_fluid * 3)
points[:, c_res - 1, :] = points[:, 0, :]
fluid_mesh.points = points.reshape(z_res * (c_res) * 2, int(r_res_fluid + r_res_fluid / 2))
fluid_mesh.plot(color='blue', show_edges=True)
return pipe_mesh, fluid_mesh
为此,我需要共享灰色和蓝色网格之间的拓扑。 是否有可以处理此问题的 MAPDL 命令?
我已经浏览了文档,但找不到任何可以做到这一点的东西(但是,我对图书馆的理解不是很好,我可能会遗漏一些东西)。
查看NUMMRG :
合并重合或等效定义的项目。
NUMMRG 命令不会更改模型的几何形状,只会更改拓扑。
合并操作对于将模型的单独但重合的部分连接在一起很有用。 如果不是要检查所有项目以进行合并,请使用选择命令(NSEL、ESEL 等)来选择项目。 节点、关键点和元素的合并操作中仅包含选定项。
在考虑了@user13974897 的回答后,我为我的问题提出了以下解决方案。
对初始方法的改进:
在这种情况下没有必要使用 PyVista,因为 PyAnsys 能够处理圆柱体。 这些圆柱体可以使用mapdl.cyl4()
方法创建,并且可以使用mapdl.vsweep()
方法进行网格mapdl.vsweep()
。
cyl4: https ://mapdldocs.pyansys.com/mapdl_commands/prep7/_autosummary/ansys.mapdl.core.Mapdl.cyl4.html ? highlight = cyl4#ansys.mapdl.core.Mapdl.cyl4
vsweep: https ://mapdldocs.pyansys.com/mapdl_commands/prep7/_autosummary/ansys.mapdl.core.Mapdl.vsweep.html ? highlight = vsweep#ansys.mapdl.core.Mapdl.vsweep
下面的方法可以应用于 PyVista polydata。 但是,不建议这样做,因为它需要使用矩形网格,这会导致流体柱内的单元严重变形(需要您通过移除体积和调整密度来进行补偿以适应)。 这可能可以通过 TetGen 使用四面体网格来解决,但尚未经过测试。
TetGen: https ://tetgen.pyvista.org/index.html
几何创建、网格划分和拓扑共享:
每个网格都必须分配一个数字标签(在分配单独的材料属性时引用)。 这些在下面的脚本中被命名为pipe_tag
和fluid_tag
。
创建管道几何体并对其进行网格划分:
mapdl = ansys.mapdl.core.launch_mapdl(loglevel='WARNING', override=True)
mapdl.units('SI')
# Create pipe mesh (nodal tag = 1):
pipe_tag = 1
mapdl.clear()
mapdl.prep7()
mapdl.et(1, "SOLID186")
mapdl.mp('DENS', pipe_tag, pipe_density)
mapdl.mp('NUXY', pipe_tag, pipe_poissons_ratio)
mapdl.mp('EX', pipe_tag, pipe_elastic_modulus)
mapdl.cyl4(xcenter=0, ycenter=0, rad1=inner_radius, rad2=outer_radius, depth=length)
mapdl.cm('Pipe','VOLU')
mapdl.esize(element_size)
mapdl.mat(pipe_tag)
mapdl.vsweep('Pipe')
类似地,创建流体柱的几何体并对其进行网格划分:
# Create fluid mesh (nodal tag = 2):
fluid_tag = 2
mapdl.et(2, "SOLID186")
mapdl.mp('DENS', fluid_tag, fluid_density)
mapdl.mp('NUXY', fluid_tag, fluid_poissons_ratio)
mapdl.mp('EX', fluid_tag, fluid_bulk_modulus)
mapdl.cyl4(xcenter=0, ycenter=0, rad1=inner_radius, depth=length)
mapdl.cm('Fluid','VOLU')
mapdl.esize(element_size)
mapdl.mat(fluid_tag)
mapdl.vsweep('Fluid')
最后,使用mapdl.nummrg()
在两个网格之间创建接触节点,设置toler
参数。 此容差决定了项目(在本例中为节点)合并的范围。
# Create contact nodes between the two meshes:
mapdl.nummrg('node', 10e-6)
我意识到对于这样一个小问题,这个答案很可能是冗长的,但有关上述内容的信息似乎很少,不足以保证这样的解释。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.