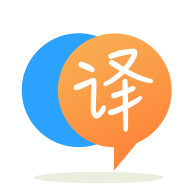
[英]How to sort a text file into a list consisting of tuples and then appending to the list in python
[英]how to sort a list in python consisting of monitor resolutions?
我想对这个列表进行排序,但找不到方法。
我/你:
['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
o / p:
['1920x1080', '1600x900', '1280x1024', '1152x864', '1024x768', '800x600', '720x400', '640x480']
提前致谢。
只需使用sorted
的key
参数:
res = sorted(data, key=lambda x: [int(s) for s in x.split("x")], reverse=True)
print(res)
Output
['1920x1080', '1600x900', '1280x1024', '1152x864', '1024x768', '800x600', '720x400', '640x480']
这将按高度打破联系
您可以使用自定义 function sorted
,这里我取两个维度的乘积(即总像素大小):
l = ['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
from math import prod
sorted(l, key=lambda x: prod(map(int, x.split('x'))), reverse=True)
output:
['1920x1080',
'1600x900',
'1280x1024',
'1152x864',
'1024x768',
'800x600',
'640x480',
'720x400']
我的最后一种方法似乎与您的案例最相关。 当您看到标有“14 英寸屏幕”的设备时,他们指的是对角线而不是高度或宽度。 所以,如果你想说 MacBook 15 > MacBook 13,那就是 go。 如果您纯粹想使用分辨率和像素,请尝试倒数第二个更相关的。
您可以使用sorted()
以及带有 lambda function 的自定义键来提取高度、宽度并应用您的计算。 根据您要执行的操作,您还需要reverse=True
。
lambda function 将每个元素作为x
,然后分别使用x.split('x')[0]
和x.split('x')[1]
提取宽度和/或高度。 然后你可以选择使用毕达哥拉斯定理来获得对角线或者只是乘积它们来获得你的像素等。
我尽量不为此使用任何外部库,例如 numpy 或数学。 在此处阅读有关已sorted
function 的更多信息。
sorted(l, key=lambda x: int(x.split('x')[0]), reverse=True)
sorted(l, key=lambda x: int(x.split('x')[1]), reverse=True)
sorted(l, key=lambda x: int(x.split('x')[0])/int(x.split('x')[1]), reverse=True)
sorted(l, key=lambda x: int(x.split('x')[0])*int(x.split('x')[1]), reverse=True)
屏幕尺寸 = sqrt((宽度^2)+(高度^2))
sorted(l, key=lambda x: (int(x.split('x')[0])**2+int(x.split('x')[1])**2)**(1/2), reverse=True)
['1920x1080',
'1600x900',
'1280x1024',
'1152x864',
'1024x768',
'800x600',
'720x400',
'640x480']
您想根据什么标准进行排序?
l = ['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
按宽度:
sorted(l, key=lambda dim: int(dim.split('x')[0]), reverse=True)
# ['1920x1080', '1600x900', '1280x1024', '1152x864', '1024x768', '800x600', '720x400', '640x480']
按身高:
sorted(l, key=lambda dim: int(dim.split('x')[1]), reverse=True)
# ['1920x1080', '1280x1024', '1600x900', '1152x864', '1024x768', '800x600', '640x480', '720x400']
按总像素数:
import math
sorted(l, key=lambda dim: math.prod(map(int, dim.split('x'))), reverse=True)
# ['1920x1080', '1600x900', '1280x1024', '1152x864', '1024x768', '800x600', '640x480', '720x400']
按对角线长度:
import math
sorted(l, key=lambda dim: math.hypot(*map(int, dim.split('x'))), reverse=True)
# ['1920x1080', '1600x900', '1280x1024', '1152x864', '1024x768', '800x600', '720x400', '640x480']
相关文件:
使用适当的排序指标,您可以将其实现为简单的 function 并将其作为key
参数传递给sort
,例如:
def key(res):
return (*map(int, s.split("x")))
resolutions.sort(key=key, reverse=True)
这将首先按宽度排序,然后按高度排序。 或者更一般地说:
def key(res):
width, height = map(int, s.split("x"))
result = # ... any numeric value calculated from the two
return result
你可以使用这个 function 它会起作用。
def orderByResolution(arr):
mytempdict = {}
result = []
for i in arr:
mykey = int(i[:i.index('x')])
mytempdict[mykey] = i
for i in reversed(sorted(mytempdict)):
result.append(mytempdict[i])
return result
如果你想按照你想要的方式排序 output 你可以试试这个:
def bubbleSort(arr):
arr = arr.copy()
n = len(arr)
# Traverse through all array elements
for i in range(n-1):
# range(n) also work but outer loop will repeat one time more than needed.
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if int(arr[j].split("x")[0]) < int(arr[j + 1].split("x")[0]) :
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
if __name__ == "__main__":
inp_reso = ['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
print(bubbleSort(inp_reso))
但是如果你想按屏幕中的总像素数排序,你必须使用 go :
def bubbleSort(arr):
arr = arr.copy()
n = len(arr)
# Traverse through all array elements
for i in range(n-1):
# range(n) also work but outer loop will repeat one time more than needed.
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if eval(arr[j].replace("x", "*")) < eval(arr[j + 1].replace("x", "*")) :
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
if __name__ == "__main__":
inp_reso = ['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
print(bubbleSort(inp_reso))
l=['1152x864', '1920x1080', '1600x900', '1280x1024', '1024x768', '640x480', '720x400', '800x600']
for i in range(len(l)):
l[i]=float(l[i].replace ('x','.'))
l.sort(reverse=True)
for i in range(len(l)):
l[i]=str(l[i]).replace ('.','x')
print(l)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.