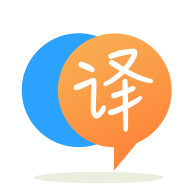
[英]Why declaring the size of an array as n, before inputting n, works for the first time but not the second time?
[英]How to sort second array and arrange first array with respect to sorted second array in less than O(n^2) time?
我已经对第二个数组进行了排序,并在 O(n^2) 时间内根据排序的第二个数组排列了第一个数组,我的代码是这样的 -
//c++ code
void sort(int size,int arr1[],int arr2[])
{
for(int i=0;i<size-1;++i)
{
for(int j=i+1;j<size;++j)
{
if(arr2[i]>arr2[j])
{
swap(arr2[i],arr2[j]);
swap(arr1[i],arr1[j]);
}
//for duplicate data in arr2
if(arr2[i]==arr2[j])
{
if(arr1[i]>arr1[j])
{
swap(arr1[i],arr1[j]);
}
}
}
}
}
例如,如果-
arr1=[2,1,3,9,7,12,5,13]
arr2=[1,3,6,9,2,3,1,3]
将 arr2 相对于 arr1 排序后
arr1=[2,5,7,1,12,13,3,9]
arr2=[1,1,2,3,3,3,6,9]
这个函数的时间复杂度是 O(n^2) 现在什么是更好的排序方法?
如this answer所示,建立一个索引并在索引上排序。
然后您可以使用索引数组重新填充数组。 然后你也可以使用std::sort ,它是O(n log n)
而不是你使用的O(n^2)
方法:
#include <algorithm>
#include <iostream>
#include <numeric>
int main()
{
int arr1 [] = {2,1,3,9,7,12,5,13};
int arr2 [] = {1,3,6,9,2,3,1,3};
int index[8];
std::iota(index, index + 8, 0);
std::sort(std::begin(index), std::end(index), [&](int n1, int n2) { return arr2[n1] < arr2[n2]; });
for (auto i : index)
std::cout << arr1[i] << " ";
std::cout << "\n";
for (auto i : index)
std::cout << arr2[i] << " ";
}
输出:
2 5 7 1 12 13 3 9
1 1 2 3 3 3 6 9
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.