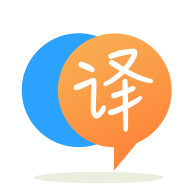
[英]how to parse json data from a rest api using C# in the ASP.Net Core MVC model and display it on a HTML page
[英]How to parse SQL JSON string in C# in asp.net mvc web api?
I have created web api in asp.net mvc where I am calling usp_JsonPract
this SP which is returning JSON string from DB, Now I am facing problem to convert this string on .net mvc web api.
我的存储过程代码:
Create proc [dbo].[usp_JsonPract]
as
BeginSelect category title
,[data] = JSON_QUERY(
(
select din.dishId,din.dishName,din.dishPrice,din.dishImage, din.dishType,
JSON_QUERY(dishPriceAndSize, '$.dishPriceAndSize') AS dishPriceAndSize,
JSON_QUERY(JAddOns, '$.addOns') AS addOns,
din.includedEggs, din.dishDescription, din.rating, din.review,din.discount
from DishMaster din
where din.category = dout.category
--and dishId in ( 11, 12,13 , 7 )
for json path
,INCLUDE_NULL_VALUES
)
)from DishMaster dout
group by category
for json path,without_array_wrapper
存储过程正在返回我想传递给客户端的 JSON 字符串。 我正在使用JsonConvert.DeserializeObject(jsonstr);
转换。
我的 C# 代码:
public object SQLJSONPract()
{
string jsonstr = string.Empty;
object o;
try
{
cmd.CommandText = "usp_JsonPract";
SqlDataAdapter adp = new SqlDataAdapter(cmd);
adp.Fill(ds);
var d = ds.Tables[0].Rows[0][0];
jsonstr = d.ToString();
object a = JsonConvert.DeserializeObject(jsonstr);
return (object)a;
}
catch (Exception ex)
{
return ex.Message;
}
}
给出如下异常:
“未终止的字符串。预期的分隔符:”。 路径
'userDetails[2].data[1].addOns[1].name'
,第 59 行,position 3。”
结果样本 JSON 是这样的:
{
"title": "Rice",
"data": [
{
"dishId": 11,
"dishName": "stream rice",
"dishPrice": 40.0,
"dishImage": "streamrice.jpg",
"dishType": "VEG",
"dishPriceAndSize": [
{
"size": "Half",
"price": 90
},
{
"size": "Full",
"price": 180
}
],
"addOns": [
{
"name": "Extrachess",
"price": 25
},
{
"name": "Chess",
"price": 20
}
],
"includedEggs": false,
"dishDescription": "stream rice is delicious in test",
"rating": 4.5,
"review": "GOOD",
"discount": 20
},
{
"dishId": 12,
"dishName": "stream rice",
"dishPrice": 40.0,
"dishImage": "streamrice.jpg",
"dishType": "VEG",
"dishPriceAndSize": [
{
"size": "Half",
"price": 90
},
{
"size": "Full",
"price": 180
}
],
"addOns": [
{
"name": "Extrachess",
"price": 25
},
{
"name": "Chess",
"price": 20
}
],
"includedEggs": false,
"dishDescription": "stream rice is delicious in test",
"rating": 4.5,
"review": "GOOD",
"discount": 20
},
{
"dishId": 13,
"dishName": "stream rice",
"dishPrice": 40.0,
"dishImage": "streamrice.jpg",
"dishType": "VEG",
"dishPriceAndSize": [
{
"size": "Half",
"price": 90
},
{
"size": "Full",
"price": 180
}
],
"addOns": [
{
"name": "Extrachess",
"price": 25
},
{
"name": "Chess",
"price": 20
}
],
"includedEggs": false,
"dishDescription": "stream rice is delicious in test",
"rating": 4.5,
"review": "GOOD",
"discount": 20
},
{
"dishId": 7,
"dishName": "Chicken Biryani",
"dishPrice": 160.0,
"dishImage": "ChickenBiryani.jpg",
"dishType": "NonVEG",
"dishPriceAndSize": [
{
"size": "Half",
"price": 90
},
{
"size": "Full",
"price": 180
}
],
"addOns": [
{
"name": "Extrachess",
"price": 25
},
{
"name": "Chess",
"price": 20
}
],
"includedEggs": false,
"dishDescription": "Special Chicken Biryani For Our Valued Guest",
"rating": 4.5,
"review": "GOOD",
"discount": 20
}
]}
如果有任何其他建议来实现这一点。 请建议。
创建一个名为JsonPract.cs
的文件并粘贴以下类,以创建几个 class 可以映射到您在问题中发布的 json :
public class JsonPract
{
public string title { get; set; }
public List<Datum> data { get; set; }
}
public class DishPriceAndSize
{
public string size { get; set; }
public int price { get; set; }
}
public class AddOn
{
public string name { get; set; }
public int price { get; set; }
}
public class Datum
{
public int dishId { get; set; }
public string dishName { get; set; }
public double dishPrice { get; set; }
public string dishImage { get; set; }
public string dishType { get; set; }
public List<DishPriceAndSize> dishPriceAndSize { get; set; }
public List<AddOn> addOns { get; set; }
public bool includedEggs { get; set; }
public string dishDescription { get; set; }
public double rating { get; set; }
public string review { get; set; }
public int discount { get; set; }
}
然后您可以将 object 反序列化为:
JsonPract myDeserializedClass = JsonConvert.DeserializeObject<JsonPract>(myJsonResponse);
例如:
public JsonPract SQLJSONPract()
{
string jsonstr = string.Empty;
object o;
try
{
cmd.CommandText = "usp_JsonPract";
SqlDataAdapter adp = new SqlDataAdapter(cmd);
adp.Fill(ds);
var d = ds.Tables[0].Rows[0][0];
jsonstr = d.ToString();
JsonPract a = JsonConvert.DeserializeObject<JsonPract>(jsonstr);
return a;
}
catch (Exception ex)
{
throw ex;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.