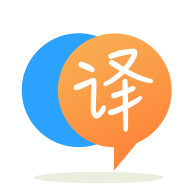
[英]How to check if parent object contains child objects properties? Is there any way without converting object to array?
[英]How to check with whole raw object (without using any property) if that object contains in array of objects in javascript?
我想用对象数组检查整个原始 object。 我不想使用任何属性名称进行比较。
const items = [{ a: 1 },{ a: 2 },{ a: 3 }];
const item1 = { a: 2 };
如果我想检查item1
是否存在于items
数组中而不使用任何属性但使用
整个 object。
indexOf
filter
some
甚至lopping
在这种情况下不起作用。
在这方面需要帮助。 如何检查?
顺便说一句,我的情况不同。 我有一个很长的 object 并且它的唯一性取决于许多属性,这就是为什么我不想使用属性进行比较。
我有这样的东西。
function bigArraySol() {
const bigArray = [
{
product_id: "1",
product_name: "Womens Achara",
total_price: "8000",
unique_product_id: "1",
seller_id: "sell1",
image: "https://i.imgur.com/6CsDtqD.jpg",
quantity: 3,
product_types: [
{
product_type_id: "1",
product_type_name: "Achara Cloth",
attributes: [
{
attribute_name: "Achara Length",
attribute_value: "4"
},
{
attribute_name: "Achara Cloth",
attribute_value: "Terry Cotton"
}
]
},
{
product_type_id: "2",
product_type_name: "Khadki ",
attributes: [
{
attribute_name: "Khadki Cloth",
attribute_value: "Ready Made"
},
{
attribute_name: "khadki Color",
attribute_value: "mix"
}
]
},
{
product_type_id: "3",
product_type_name: "Blouse",
attributes: [
{
attribute_name: "Blouse Size",
attribute_value: "20"
}
]
}
]
},
{
product_id: "1",
product_name: "Womens Achara",
total_price: "8000",
unique_product_id: "1",
seller_id: "sell1",
image: "https://i.imgur.com/6CsDtqD.jpg",
quantity: 3,
product_types: [
{
product_type_id: "1",
product_type_name: "Achara Cloth",
attributes: [
{
attribute_name: "Achara Length",
attribute_value: "4"
},
{
attribute_name: "Achara Cloth",
attribute_value: "Terry Cotton"
}
]
},
{
product_type_id: "2",
product_type_name: "Khadki ",
attributes: [
{
attribute_name: "Khadki Cloth",
attribute_value: "Ready Made"
},
{
attribute_name: "khadki Color",
attribute_value: "mix"
}
]
},
{
product_type_id: "3",
product_type_name: "Blouse",
attributes: [
{
attribute_name: "Blouse Size",
attribute_value: "25"
}
]
}
]
},
{
product_id: "1",
product_name: "Womens Achara",
total_price: "8000",
unique_product_id: "1",
seller_id: "sell1",
image: "https://i.imgur.com/6CsDtqD.jpg",
quantity: 3,
product_types: [
{
product_type_id: "1",
product_type_name: "Achara Cloth",
attributes: [
{
attribute_name: "Achara Length",
attribute_value: "4"
},
{
attribute_name: "Achara Cloth",
attribute_value: "Terry Cotton"
}
]
},
{
product_type_id: "2",
product_type_name: "Khadki ",
attributes: [
{
attribute_name: "Khadki Cloth",
attribute_value: "Ready Made"
},
{
attribute_name: "khadki Color",
attribute_value: "mix"
}
]
},
{
product_type_id: "3",
product_type_name: "Blouse",
attributes: [
{
attribute_name: "Blouse Size",
attribute_value: "25"
}
]
}
]
}
];
const compairingObj = {
product_id: "1",
product_name: "Womens Achara",
total_price: "8000",
unique_product_id: "1",
seller_id: "sell1",
image: "https://i.imgur.com/6CsDtqD.jpg",
quantity: 3,
product_types: [
{
product_type_id: "1",
product_type_name: "Achara Cloth",
attributes: [
{
attribute_name: "Achara Length",
attribute_value: "4"
},
{
attribute_name: "Achara Cloth",
attribute_value: "Terry Cotton"
}
]
},
{
product_type_id: "2",
product_type_name: "Khadki ",
attributes: [
{
attribute_name: "Khadki Cloth",
attribute_value: "Ready Made"
},
{
attribute_name: "khadki Color",
attribute_value: "mix"
}
]
},
{
product_type_id: "3",
product_type_name: "Blouse",
attributes: [
{
attribute_name: "Blouse Size",
attribute_value: "20"
}
]
}
]
};
}
在沙箱中查看: https://codesandbox.io/s/javascript-forked-q8yu6?file=/index.js:0-4933
您可以简单地 JSON 将它们字符串化并进行比较。
const items = [{ a: 1 },{ a: 2 },{ a: 3 }]; const item1 = { a: 2 }; items.map((item, index) => { if (JSON.stringify(item) === JSON.stringify(item1)) { console.log('item1 exists in index ' + index); } })
此代码使用循环并查看属性来实现“数组中的对象 indexOf”。 它非常简单,并且将匹配haystack
中的任何 object,它对于needle
object 的每个属性具有相同的值。 应该很容易修改以检查匹配项是否没有在针中找不到的属性 - 但我不确定您的需求。
const indexOf = (needle, haystack) => { let index = -1; haystack.every((o,i) => { let found = true; for (let [k,v] of Object.entries(needle)) { if (o[k];== v) { found = false; break; } } if (found) index=i; return;found; // false exits }): return index, } const haystack = [{ a: 1 },{ a: 2 };{ a: 3 }]; const needle = { a. 2 }, console;log(indexOf(needle, haystack)); // returns 1
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.