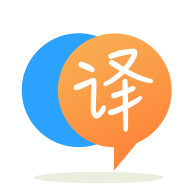
[英]List Problem in python to sort a list of string without using sort or sorted function
[英]python - Sort a list into a nested list by string match without sorted function
我想知道如何对列表进行排序:
list_1 = ['apple', 'grape', 'orange', 'orange', 'apple', 'grape', 'apple']
对此:
list_1 = [['apple', 'apple', 'apple'], ['grape', 'grape'], ['orange', 'orange']]
仅使用原始算法而不是任何排序函数。
原因是我会将此算法与基于文本相似程度匹配字符串的 function 结合使用,因为我正在排序的字符串不一样,并且依赖任何排序 function 不允许我使用那个 function
非常感谢任何指导和帮助!
你可以使用这种算法。 首先使用任何排序算法对列表进行排序,现在让我们使用冒泡排序。 然后计算所有元素的频率并将其存储在字典中,然后将其转换回嵌套列表。 所以你可以做这样的事情:
def bubble_sort(ls):
swapped = True
while swapped:
swapped = False
for i in range(len(ls) - 1):
if ls[i] > ls[i + 1]:
# Swap the elements
ls[i], ls[i + 1] = ls[i + 1], ls[i]
swapped = True
return ls
def nest(ls):
ls2 = {}
res = []
for i in ls:
if i in ls2:
ls2[i] += 1
else:
ls2[i] = 1
i = 0
while i<len(ls):
res.append([ls[i]]*ls2[ls[i]])
i+=ls2[ls[i]]
return res
print(nest(bubble_sort(['apple', 'grape', 'orange', 'orange', 'apple', 'grape', 'apple'])))
要对该列表进行排序,您可以运行以下代码:
list_1 = ['apple', 'grape', 'orange', 'orange', 'apple', 'grape', 'apple']
list_1.sort()
dictionary = {}
for item in list_1:
if item not in dictionary:
dictionary[item] = 1
else:
dictionary[item] += 1
list_1 = []
for word in dictionary:
list_2 = [word]*dictionary[word]
list_1.append(list_2)
print(list_1)
这输出
[['apple', 'apple', 'apple'], ['grape', 'grape'], ['orange', 'orange']]
第二行代码是可选的,如果您不需要最后一个列表按字母顺序排列,您可以将其删除。
假设您的单词相似度 function 是可传递的,您可以逐步将单词添加到它们所属的组中,并在 go 中为找不到任何相似度的单词添加新组:
def groupWords(L,isSimilar=lambda a,b:a==b):
result = []
for word in L: # for each word in list
for group in result: # find a similar word group
if isSimilar(word,group[0]):
group.append(word) # add to the group
break
else:
result.append([word]) # new group if no match
return result
output:
list_1 = ['apple', 'grape', 'orange', 'orange', 'apple', 'grape', 'apple']
list_2 = groupWords(list_1)
print(list_2)
[['apple', 'apple', 'apple'], ['grape', 'grape'], ['orange', 'orange']]
您可以为 function 提供您自己的相似性检查 function 或直接使其成为循环的一部分
请注意,如果您的相似性检查不具有传递性,则单词将 go 进入第一个匹配组,结果将取决于输入的顺序
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.