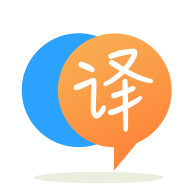
[英]Finding the k-mer that results in minimum hamming distance in a list of dna
[英]Finding the minimum result of a list of results
是否有定义/排序列表的代码?
您可以将 python 的min
function 与key
参数一起使用,如下所示:
def find_closest(start_point, remaining_points):
return min(remaining_points, key=lambda a: distance(start_point, a))
由于您的特定需求(仅循环和 if 语句),这是另一种解决方案。 对于其他不受限制的人,我建议使用上述解决方案。
def find_closest(start_point, remaining_points):
closest = None
closest_distance = 1000000
# Better but more advanced initialisation
# closest_distance = float("inf")
for element in list(remaining_points):
dist = distance(start_point, element)
if dist < closest_distance:
closest = element
closest_distance = dist
return closest
在遍历所有点之前,我们将closest
点初始化为None
(尚未找到)并将closest_distante
为非常高的值(以确保第一个评估点更近)。
然后,对于remaining_points
中的每个点,我们计算它与start_point
的距离并将其存储在dist
中。
如果这个距离dist
小于closest_distance
,那么当前点离当前存储的点closest
,所以我们用当前点更新存储的最近点,并用当前距离dist
更新closest_distance
。
当所有点都被评估后,我们返回最近的点closest
。
min
function: https://www.programiz.com/python-programming/methods/built-in/minlambda
function: https://www.w3schools.com/python/python_lambda.asp一个快速而直接的解决方案是创建一个结果列表,然后用你的剩余点列表来证实索引(因为它们本质上是排成一行的)。 下面是实现这一目标的分步过程,最底部是代码的清理版本。
def find_closest(start_point, remaining_points):
results = [] # list of results for later use
for element in list(remaining_points):
result = distance(start_point, element)
results.append(result) # append the result to the list
# After iteration is finished, find the lowest result
lowest_result = min(results)
# Find the index of the lowest result
lowest_result_index = results.index(lowest_result)
# Corroborate this with the inputs
closest_point = remaining_points[lowest_result_index]
# Return the closest point
return closest_point
或者简化代码:
def find_closest(start_point, remaining_points):
results = []
for element in remaining_points:
results.append(distance(start_point, element))
return remaining_points[results.index(min(results))]
编辑:您评论说您不能使用 Python 的内置min()
function。解决方案是创建您自己的功能性minimum_value()
function。
def minimum_value(lst: list):
min_val = lst[0]
for item in lst:
if item < min_val:
min_val = item
return min_val
def find_closest(start_point, remaining_points):
results = []
for element in remaining_points:
results.append(distance(start_point, element))
return remaining_points[results.index(minimum_value(results))]
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.